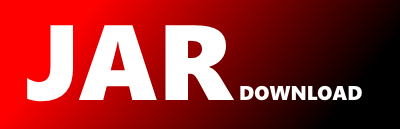
com.github.springbootPlus.excel.result.ExcelImportResult Maven / Gradle / Ivy
package com.github.springbootPlus.excel.result;
import com.github.springbootPlus.excel.parsing.ExcelError;
import org.apache.commons.collections.CollectionUtils;
import java.util.*;
/**
* Excel导入结果
*
* @author lisuo
*/
public class ExcelImportResult {
int titleIndex = 0;
/**
* 头信息,标题行之前的数据,每行表示一个List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy