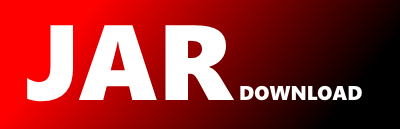
com.github.springbootPlus.excel.util.ExcelUtil Maven / Gradle / Ivy
The newest version!
package com.github.springbootPlus.excel.util;
import com.github.springbootPlus.excel.parsing.ExcelError;
import com.github.springbootPlus.excel.result.ExcelImportResult;
import org.apache.commons.beanutils.PropertyUtils;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.ss.util.NumberToTextConverter;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Objects;
/**
* Excel 操作工具类
*
* @author lisuo
*/
public abstract class ExcelUtil {
/**
* 读取Excel,支持任何不规则的Excel文件,
* 外层List表示所有的数据行,内层List表示每行中的cell单元数据位置
* 假设获取一个Excel第三行第二个单元格的数据,例子代码:
* FileInputStream excelStream = new FileInputStream(path);
* List> list = ExcelUtil.readExcel(excelStream);
* System.out.println(list.get(2).get(1));//第三行第二列,索引行位置是2,列的索引位置是1
*
* @param excelStream Excel文件流
* @param sheetIndex Excel-Sheet 的索引
* @return List>
* @throws Exception
*/
public static List> readExcel(InputStream excelStream, int sheetIndex) throws Exception {
List> datas = new ArrayList>();
Workbook workbook = WorkbookFactory.create(excelStream);
//只读取第一个sheet
Sheet sheet = workbook.getSheetAt(sheetIndex);
int rows = sheet.getPhysicalNumberOfRows();
for (int i = 0; i < rows; i++) {
Row row = sheet.getRow(i);
short cellNum = row.getLastCellNum();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy