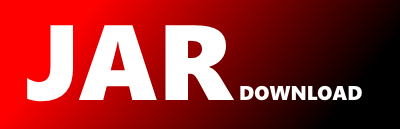
org.springframework.web.servlet.view.mustache.MustacheViewResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mustache-spring-view Show documentation
Show all versions of mustache-spring-view Show documentation
a spring view for the mustache templating language http://mustache.github.com/
/*
* Copyright 2011 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springframework.web.servlet.view.mustache;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Required;
import org.springframework.web.servlet.ViewResolver;
import org.springframework.web.servlet.view.AbstractTemplateViewResolver;
import org.springframework.web.servlet.view.AbstractUrlBasedView;
import com.samskivert.mustache.Mustache;
import com.samskivert.mustache.Template;
import com.samskivert.mustache.Mustache.Compiler;
/**
* @author Sean Scanlon
*
*/
public class MustacheViewResolver extends AbstractTemplateViewResolver implements ViewResolver,
InitializingBean {
private MustacheTemplateLoader templateLoader;
private Compiler compiler = null;
private boolean standardsMode = false;
private boolean escapeHTML = true;
public MustacheViewResolver() {
setViewClass(MustacheView.class);
}
@Override
protected Class> requiredViewClass() {
return MustacheView.class;
}
@Override
protected AbstractUrlBasedView buildView(String viewName) throws Exception {
final MustacheView view = (MustacheView) super.buildView(viewName);
Template template = compiler.compile(templateLoader.getTemplate(view.getUrl()));
view.setTemplate(template);
return view;
}
public void afterPropertiesSet() throws Exception {
templateLoader.setPrefix(getPrefix());
templateLoader.setSuffix(getSuffix());
if (compiler == null) {
compiler = Mustache.compiler()
.escapeHTML(escapeHTML)
.standardsMode(standardsMode)
.withLoader(templateLoader);
}
}
@Required
public void setTemplateLoader(MustacheTemplateLoader templateLoader) {
this.templateLoader = templateLoader;
}
/**
* Whether or not standards mode is enabled.
*
* disabled by default.
*/
public void setStandardsMode(boolean standardsMode) {
this.standardsMode = standardsMode;
}
/**
* Whether or not HTML entities are escaped by default.
*
* default is true.
*/
public void setEscapeHTML(boolean escapeHTML) {
this.escapeHTML = escapeHTML;
}
/**
* You can inject your own custom configured compiler. If you don't inject one then a default one will be created
* for you instead using the standardsMode, escapeHTML, and templateLoader values you've injected.
*
* @param compiler
*/
public void setCompiler(Compiler compiler) {
this.compiler = compiler;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy