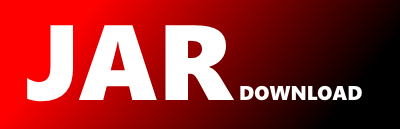
org.springframework.boot.autoconfigure.jdbc.plus.JdbcUrl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-starter-data-jpa-plus Show documentation
Show all versions of spring-boot-starter-data-jpa-plus Show documentation
Extended implementation of Spring Boot Data JPA Starter
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springframework.boot.autoconfigure.jdbc.plus;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* JDBC URL
*/
public abstract class JdbcUrl { // @checkstyle:ok
/**
* Constructor
*
* @param url URL
* @throws IllegalArgumentException if failed to parse URL
*/
public JdbcUrl(String url) throws IllegalArgumentException {
// check format
Matcher matcher = Pattern.compile(this.getUrlPattern()).matcher(url);
if (!matcher.matches() || matcher.groupCount() != 2) {
throw new IllegalArgumentException("Failed to parse URL: " + url);
}
// extract parameters
Map params = new LinkedHashMap<>();
for (String query : matcher.group(2).trim().replaceAll("^" + Pattern.quote(this.getQueryStarter()), "")
.split(Pattern.quote(this.getQueryJoiner()))) {
query = query.trim();
if (query.isEmpty()) {
continue;
}
String[] pieces = query.split(Pattern.quote(this.getParamJoiner()));
if (pieces.length != 2) {
continue;
}
params.put(pieces[0].trim(), pieces[1].trim());
}
this.path = matcher.group(1);
this.params = params;
}
/**
* Path
*/
private String path;
/**
* Parameters
*/
private Map params = new LinkedHashMap<>();
/**
* Add parameter
*
* @param key key
* @param value value
*/
protected void addParam(String key, Object value) {
this.params.put(key, value);
}
/**
* Get URL pattern
*
* @return URL pattern
*/
protected abstract String getUrlPattern();
/**
* Get prefix
*
* @return prefix
*/
protected abstract String getPrefix();
/**
* Get query starter
*
* @return query starter
*/
protected abstract String getQueryStarter();
/**
* Get query joiner
*
* @return query joiner
*/
protected abstract String getQueryJoiner();
/**
* Get parameter joiner
*
* @return parameter joiner
*/
protected abstract String getParamJoiner();
/**
* Build URL
*
* @return URL
*/
@Override
public String toString() {
List queries = new ArrayList<>();
for (Entry entry : this.params.entrySet()) {
StringBuilder query = new StringBuilder();
query.append(entry.getKey());
query.append(this.getParamJoiner());
query.append(this.convertParamValue(entry.getValue()));
queries.add(query.toString());
}
StringBuilder url = new StringBuilder();
url.append(this.getPrefix());
url.append(this.path);
if (!queries.isEmpty()) {
url.append(this.getQueryStarter());
url.append(String.join(this.getQueryJoiner(), queries));
}
return url.toString();
}
/**
* Convert parameter value
*
* @param value value
* @return converted value
*/
protected String convertParamValue(Object value) {
return String.valueOf(value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy