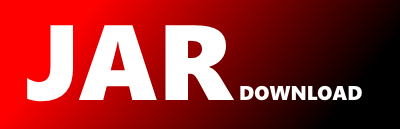
org.springframework.data.redis.cache.RedisCacheManagerAdapter Maven / Gradle / Ivy
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.springframework.data.redis.cache;
import java.lang.reflect.Field;
import java.util.Map;
import org.springframework.beans.BeanUtils;
import org.springframework.data.redis.core.RedisOperations;
import org.springframework.util.ReflectionUtils;
/**
* {@link RedisCacheManager} adapter
*/
public abstract class RedisCacheManagerAdapter extends RedisCacheManager { // @checkstyle:ok
/**
* Constructor
*
* @param source {@link RedisCacheManager}
*/
public RedisCacheManagerAdapter(RedisCacheManager source) {
super(getRedisOperations(source));
BeanUtils.copyProperties(source, this);
this.setUsePrefix(getUsePrefix(source));
this.setCachePrefix(getCachePrefix(source));
this.setLoadRemoteCachesOnStartup(getLoadRemoteCachesOnStartup(source));
this.setDefaultExpiration(getDefaultExpiration(source));
this.setExpires(getExpires(source));
}
/**
* Constructor
*
* @param redisOperations {@link RedisOperations}
*/
public RedisCacheManagerAdapter(RedisOperations, ?> redisOperations) {
super(redisOperations);
}
@Override
protected RedisCache createCache(String cacheName) {
return this.decorateCache(super.createCache(cacheName), cacheName);
}
/**
* Decorate {@link RedisCache}
*
* @param cache {@link RedisCache}
* @param cacheName cache name
* @return {@link RedisCache}
*/
protected RedisCache decorateCache(RedisCache cache, String cacheName) {
return cache;
}
/**
* Get {@link RedisOperations}
*
* @param manager {@link RedisCacheManager}
* @return {@link RedisOperations}
*/
public static RedisOperations, ?> getRedisOperations(RedisCacheManager manager) {
Field field = ReflectionUtils.findField(RedisCacheManager.class, "redisOperations");
ReflectionUtils.makeAccessible(field);
return (RedisOperations, ?>) ReflectionUtils.getField(field, manager);
}
/**
* Get {@link #isUsePrefix()}
*
* @param manager {@link RedisCacheManager}
* @return {@link #isUsePrefix()}
*/
public static boolean getUsePrefix(RedisCacheManager manager) {
Field field = ReflectionUtils.findField(RedisCacheManager.class, "usePrefix");
ReflectionUtils.makeAccessible(field);
return (boolean) ReflectionUtils.getField(field, manager);
}
/**
* Get {@link RedisCachePrefix}
*
* @param manager {@link RedisCacheManager}
* @return {@link RedisCachePrefix}
*/
public static RedisCachePrefix getCachePrefix(RedisCacheManager manager) {
Field field = ReflectionUtils.findField(RedisCacheManager.class, "cachePrefix");
ReflectionUtils.makeAccessible(field);
return (RedisCachePrefix) ReflectionUtils.getField(field, manager);
}
/**
* Get {@link #setLoadRemoteCachesOnStartup(boolean)}
*
* @param manager {@link RedisCacheManager}
* @return {@link #setLoadRemoteCachesOnStartup(boolean)}
*/
public static boolean getLoadRemoteCachesOnStartup(RedisCacheManager manager) {
Field field = ReflectionUtils.findField(RedisCacheManager.class, "loadRemoteCachesOnStartup");
ReflectionUtils.makeAccessible(field);
return (boolean) ReflectionUtils.getField(field, manager);
}
/**
* Get {@link #setDefaultExpiration(long)}
*
* @param manager {@link RedisCacheManager}
* @return {@link #setDefaultExpiration(long)}
*/
public static long getDefaultExpiration(RedisCacheManager manager) {
Field field = ReflectionUtils.findField(RedisCacheManager.class, "defaultExpiration");
ReflectionUtils.makeAccessible(field);
return (long) ReflectionUtils.getField(field, manager);
}
/**
* Get {@link #setExpires(Map)}
*
* @param manager {@link RedisCacheManager}
* @return {@link #setExpires(Map)}
*/
public static Map getExpires(RedisCacheManager manager) {
Field field = ReflectionUtils.findField(RedisCacheManager.class, "expires");
ReflectionUtils.makeAccessible(field);
@SuppressWarnings("unchecked")
Map expires = (Map) ReflectionUtils.getField(field, manager);
return expires;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy