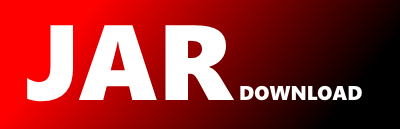
spullara.util.concurrent.Promises Maven / Gradle / Ivy
The newest version!
package spullara.util.concurrent;
import spullara.util.functions.Block;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
public class Promises {
// Code Coverage
private Promises() {}
static {new Promises();}
public static Promise execute(ExecutorService es, final Callable callable) {
final Promise promise = new Promise();
es.submit(new Runnable() {
@Override
public void run() {
try {
promise.set(callable.call());
} catch (Throwable th) {
promise.setException(th);
}
}
});
return promise;
}
public static Promise> collect(List> promises) {
final Promise> promiseOfList = new Promise>();
final int size = promises.size();
final List list = Collections.synchronizedList(new ArrayList(size));
if (promises.size() == 0) {
promiseOfList.set(list);
} else {
for (Promise promise : promises) {
promise.onSuccess(new Block() {
@Override
public void apply(T t) {
list.add(t);
if (list.size() == size) {
promiseOfList.set(list);
}
}
}).onFailure(new Block() {
@Override
public void apply(Throwable throwable) {
promiseOfList.setException(throwable);
}
});
}
}
return promiseOfList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy