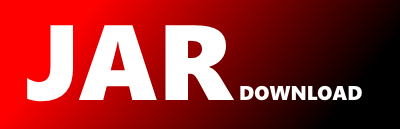
com.github.mustachejava.util.DecoratedCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compiler Show documentation
Show all versions of compiler Show documentation
Implementation of mustache.js for Java
package com.github.mustachejava.util;
import java.util.AbstractCollection;
import java.util.Collection;
import java.util.Iterator;
/**
* Exposes first / last / index / value on each element.
*/
public class DecoratedCollection extends AbstractCollection> {
private final Collection c;
public DecoratedCollection(Collection c) {
this.c = c;
}
@Override
public Iterator> iterator() {
final Iterator iterator = c.iterator();
return new Iterator>() {
int index = 0;
@Override
public boolean hasNext() {
return iterator.hasNext();
}
@Override
public Element next() {
T next = iterator.next();
int current = index++;
return new Element<>(current, current == 0, !iterator.hasNext(), next);
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
};
}
@Override
public int size() {
return c.size();
}
}
class Element {
public final int index;
public final boolean first;
public final boolean last;
public final T value;
public Element(int index, boolean first, boolean last, T value) {
this.index = index;
this.first = first;
this.last = last;
this.value = value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy