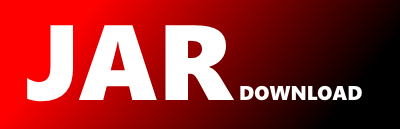
com.sampullara.mustache.ObjectHandler7 Maven / Gradle / Ivy
package com.sampullara.mustache;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.logging.Level;
import java.util.logging.Logger;
import static com.sampullara.mustache.ObjectHandler6.getField;
import static com.sampullara.mustache.ObjectHandler6.getMethod;
/**
* Implementation of Handle Object for Java VMs that include MethodHandles.
*
* User: sam
* Date: 7/24/11
* Time: 2:56 PM
*/
public class ObjectHandler7 implements ObjectHandler {
private static Logger logger = Logger.getLogger(Mustache.class.getName());
private static ClassValue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy