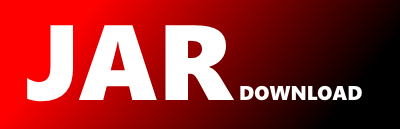
com.github.squirrelgrip.extension.protobuf.ProtobufExtension.kt Maven / Gradle / Ivy
The newest version!
package com.github.squirrelgrip.extension.protobuf
import com.fasterxml.jackson.core.JsonParser
import com.fasterxml.jackson.databind.JsonNode
import com.fasterxml.jackson.dataformat.protobuf.ProtobufMapper
import com.fasterxml.jackson.dataformat.protobuf.schema.ProtobufSchema
import com.github.squirrelgrip.format.ObjectMapperFactory
import com.github.squirrelgrip.format.SchemaDataFormat
import com.github.squirrelgrip.util.notCatching
import java.io.*
import java.net.URL
import java.nio.file.Path
object Protobuf : SchemaDataFormat(
object : ObjectMapperFactory {
override fun builder(): ProtobufMapper.Builder =
ProtobufMapper.builder()
}
) {
override fun getSchema(clazz: Class<*>): ProtobufSchema =
objectMapper.generateSchemaFor(clazz)
}
/**
* Converts Any to a Protobuf representation
*/
fun Any.toProtobuf(schema: ProtobufSchema = Protobuf.getSchema(this.javaClass)): ByteArray = Protobuf.objectWriter(schema).writeValueAsBytes(this)
fun Any.toProtobuf(file: File, schema: ProtobufSchema = Protobuf.getSchema(this.javaClass)) = Protobuf.objectWriter(schema).writeValue(file, this)
fun Any.toProtobuf(path: Path, schema: ProtobufSchema = Protobuf.getSchema(this.javaClass)) = Protobuf.objectWriter(schema).writeValue(path.toFile(), this)
fun Any.toProtobuf(outputStream: OutputStream, schema: ProtobufSchema = Protobuf.getSchema(this.javaClass)) = Protobuf.objectWriter(schema).writeValue(outputStream, this)
fun Any.toProtobuf(writer: Writer, schema: ProtobufSchema = Protobuf.getSchema(this.javaClass)) = Protobuf.objectWriter(schema).writeValue(writer, this)
fun Any.toProtobuf(dataOutput: DataOutput, schema: ProtobufSchema = Protobuf.getSchema(this.javaClass)) = Protobuf.objectWriter(schema).writeValue(dataOutput, this)
inline fun String.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this)
inline fun InputStream.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this)
inline fun Reader.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this)
inline fun URL.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this)
inline fun ByteArray.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this)
inline fun ByteArray.toInstance(offset: Int, len: Int, schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this, offset, len)
inline fun DataInput.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this)
inline fun File.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this)
inline fun Path.toInstance(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): T = Protobuf.objectReader(schema).readValue(this.toFile())
inline fun String.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this)
inline fun InputStream.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this)
inline fun Reader.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this)
inline fun URL.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this)
inline fun ByteArray.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this)
inline fun ByteArray.toInstanceList(offset: Int, len: Int, schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this, offset, len)
inline fun DataInput.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this)
inline fun File.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this)
inline fun Path.toInstanceList(schema: ProtobufSchema = Protobuf.getSchema(T::class.java)): List = Protobuf.listObjectReader(schema).readValue(this.toFile())
fun String.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this)
fun InputStream.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this)
fun Reader.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this)
fun URL.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this)
fun ByteArray.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this)
fun ByteArray.toJsonNode(offset: Int, length: Int): JsonNode = Protobuf.objectMapper.readTree(this, offset, length)
fun JsonParser.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this)
fun File.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this)
fun Path.toJsonNode(): JsonNode = Protobuf.objectMapper.readTree(this.toFile())
fun String.isProtobuf(): Boolean = notCatching { this.toJsonNode() }
fun InputStream.isProtobuf(): Boolean = notCatching { this.toJsonNode() }
fun Reader.isProtobuf(): Boolean = notCatching { this.toJsonNode() }
fun URL.isProtobuf(): Boolean = notCatching { this.toJsonNode() }
fun ByteArray.isProtobuf(): Boolean = notCatching { this.toJsonNode() }
fun ByteArray.isProtobuf(offset: Int, length: Int): Boolean = notCatching { this.toJsonNode(offset, length) }
fun JsonParser.isProtobuf(): Boolean = notCatching { this.toJsonNode() }
fun File.isProtobuf(): Boolean = notCatching { this.toJsonNode() }
fun Path.isProtobuf(): Boolean = notCatching { this.toFile().toJsonNode() }
© 2015 - 2024 Weber Informatics LLC | Privacy Policy