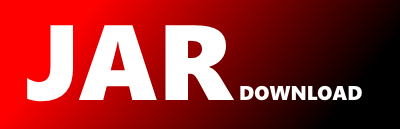
com.github.stefanbirkner.editors.mapper.MapperBuilder Maven / Gradle / Ivy
The newest version!
package com.github.stefanbirkner.editors.mapper;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
/**
* A {@code MapperBuilder} helps you to build a {@link Mapper} by specifying
* the mapping for each pair of String and Object. This is mostly useful for
* building {@link Mapper}s for tests.
*
*
* MapperBuilder<Long> builder = new MapperBuilder<Long>();
* builder.addMapping(1L, "one");
* builder.addMapping(2L, "two");
* Mapper<Long> mapper = builder.toMapper();
* mapper.getValueForText("one"); // returns 1
* mapper.getTextForValue(2L); // returns "two"
*
*
* By default every undefined value and text is mapped to {@code null}.
*
*
* mapper.getValueForText("unknown"); // returns null
* mapper.getTextForValue(20L); // returns null
*
*
* You may specify a default text and value for unmapped values and texts.
*
*
* builder.setDefaultValue(1000L);
* mapper.getValueForText("unknown"); // returns 1000L
*
* builder.setDefaultText("undefined");
* mapper.getTextForValue(20L); // returns "undefined"
*
*
* You can build the {@link Mapper} with a single statement.
*
*
* Mapper<Long> mapper = new MapperBuilder<Long>()
* .addMapping(1L, "one")
* .addMapping(2L, "two")
* .setDefaultValue(1000L)
* .setDefaultText("undefined")
* .toMapper();
*
* @param the type of the mapped values.
* @author Stefan Birkner
* @since 2.1.0
*/
public class MapperBuilder {
private final Map textsForValues = new HashMap();
private T defaultValue = null;
private String defaultText = null;
/**
* Adds a mapping between the specified value and the text.
*
* MapperBuilder<Long> builder = new MapperBuilder<Long>();
* builder.addMapping(1L, "one");
* Mapper<Long> mapper = builder.toMapper();
* mapper.getValueForText("one"); // returns 1
* mapper.getTextForValue(1L); // returns "one"
*
*/
public void addMapping(T value, String text) {
textsForValues.put(value, text);
}
/**
* Sets the text for values that have no mapping. (@code null} is the default.
*
* MapperBuilder<Long> builder = new MapperBuilder<Long>();
* builder.setDefaultText("default text");
* Mapper<Long> mapper = builder.toMapper();
* mapper.getTextForValue(1L); // returns "default text"
*
*/
public void setDefaultText(String defaultText) {
this.defaultText = defaultText;
}
/**
* Sets the value for texts that have no mapping. (@code null} is the default.
*
* MapperBuilder<Long> builder = new MapperBuilder<Long>();
* builder.setDefaultValue(1L);
* Mapper<Long> mapper = builder.toMapper();
* mapper.getValueForText("undefined"); // returns 1L
*
*/
public void setDefaultValue(T defaultValue) {
this.defaultValue = defaultValue;
}
public Mapper toMapper() {
return new MapBasedMapper(textsForValues, defaultValue, defaultText);
}
private static class MapBasedMapper implements Mapper {
private final Map valuesForTexts = new HashMap();
private final Map textsForValues;
private final String defaultText;
private final T defaultValue;
public MapBasedMapper(Map textsForValues, T defaultValue, String defaultText) {
this.defaultValue = defaultValue;
this.textsForValues = new HashMap(textsForValues); //prevent modification
this.defaultText = defaultText;
for (Entry valueAndText : textsForValues.entrySet())
valuesForTexts.put(valueAndText.getValue(), valueAndText.getKey());
}
@Override
public T getValueForText(String text) {
T value = valuesForTexts.get(text);
return defaultIfNull(value, defaultValue);
}
@Override
public String getTextForValue(T value) {
String text = textsForValues.get(value);
return defaultIfNull(text, defaultText);
}
private S defaultIfNull(S value, S valueIfNull) {
return (value == null) ? valueIfNull : value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy