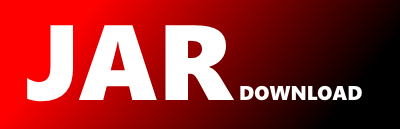
de.japkit.el.juel.DynamicFunctionMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of japkit-juel Show documentation
Show all versions of japkit-juel Show documentation
Include this dependency to use Java EL expressions in your code templates.
package de.japkit.el.juel;
import com.google.common.base.Objects;
import de.japkit.el.ElExtensions;
import de.japkit.el.juel.MethodCache;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Map;
import javax.el.FunctionMapper;
import net.bytebuddy.ByteBuddy;
import net.bytebuddy.dynamic.ClassLoadingStrategy;
import net.bytebuddy.dynamic.DynamicType;
import net.bytebuddy.instrumentation.MethodDelegation;
import net.bytebuddy.modifier.MethodArguments;
import net.bytebuddy.modifier.Ownership;
import net.bytebuddy.modifier.Visibility;
import org.eclipse.xtend.lib.annotations.Data;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Pure;
import org.eclipse.xtext.xbase.lib.StringExtensions;
import org.eclipse.xtext.xbase.lib.util.ToStringBuilder;
/**
* A function mapper that allows to call functions on value stack.
*/
@Data
@SuppressWarnings("all")
public class DynamicFunctionMapper extends FunctionMapper {
public static class Invoker {
private final Object function;
public Invoker(final Object function) {
this.function = function;
}
public Object invoke(final Object... params) {
return ElExtensions.invoke(this.function, null, params);
}
}
private final Map contextMap;
private final MethodCache methodCache;
@Override
public Method resolveFunction(final String prefix, final String localName) {
Method _xblockexpression = null;
{
boolean _isNullOrEmpty = StringExtensions.isNullOrEmpty(prefix);
boolean _not = (!_isNullOrEmpty);
if (_not) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Prefix ");
_builder.append(prefix, "");
_builder.append(" not supported.");
throw new UnsupportedOperationException(_builder.toString());
}
final Object function = this.contextMap.get(localName);
boolean _equals = Objects.equal(function, null);
if (_equals) {
return null;
}
Method _elvis = null;
Method _get = this.methodCache.get(function);
if (_get != null) {
_elvis = _get;
} else {
Method _xblockexpression_1 = null;
{
final DynamicFunctionMapper.Invoker invoker = new DynamicFunctionMapper.Invoker(function);
final Method method = this.createStaticDelegateMethod(localName, invoker);
final Method method1 = method;
this.methodCache.put(function, method1);
_xblockexpression_1 = method1;
}
_elvis = _xblockexpression_1;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Method createStaticDelegateMethod(final String functionName, final DynamicFunctionMapper.Invoker invoker) {
try {
Method _xblockexpression = null;
{
final ArrayList> argTypes = new ArrayList>();
argTypes.add(Object[].class);
ByteBuddy _byteBuddy = new ByteBuddy();
DynamicType.Builder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy