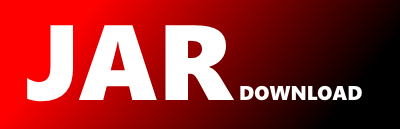
com.github.stephengold.joltjni.SoftBodyCreationSettings Maven / Gradle / Ivy
/*
Copyright (c) 2024 Stephen Gold
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
package com.github.stephengold.joltjni;
import com.github.stephengold.joltjni.readonly.ConstSoftBodyCreationSettings;
import com.github.stephengold.joltjni.readonly.ConstSoftBodySharedSettings;
import com.github.stephengold.joltjni.readonly.QuatArg;
import com.github.stephengold.joltjni.readonly.RVec3Arg;
/**
* Settings used to create a soft body.
*
* @author Stephen Gold [email protected]
*/
public class SoftBodyCreationSettings
extends JoltPhysicsObject
implements ConstSoftBodyCreationSettings {
// *************************************************************************
// constructors
/**
* Instantiate default settings.
*/
public SoftBodyCreationSettings() {
long bodySettingsVa = createDefault();
setVirtualAddress(bodySettingsVa, () -> free(bodySettingsVa));
}
/**
* Instantiate settings for the specified shared settings.
*
* @param settings the shared settings to use (not null, unaffected)
* @param location the desired initial location (in system coordinates, not
* null, unaffected)
* @param orientation the desired initial orientation (relative to system
* axes, not null, unaffected)
* @param objectLayer the desired object layer (≥0)
*/
public SoftBodyCreationSettings(ConstSoftBodySharedSettings settings,
RVec3Arg location, QuatArg orientation, int objectLayer) {
long sharedSettingsVa = settings.targetVa();
double xx = location.xx();
double yy = location.yy();
double zz = location.zz();
float qw = orientation.getW();
float qx = orientation.getX();
float qy = orientation.getY();
float qz = orientation.getZ();
long bodySettingsVa = createFromSharedSettings(
sharedSettingsVa, xx, yy, zz, qx, qy, qz, qw, objectLayer);
setVirtualAddress(bodySettingsVa, () -> free(bodySettingsVa));
}
// *************************************************************************
// new methods exposed
/**
* Alter whether the created body will be allowed to fall asleep. (native
* attribute: mAllowSleeping)
*
* @param allow {@code true} to allow, {@code false} to inhibit
* (default=true)
*/
public void setAllowSleeping(boolean allow) {
long bodySettingsVa = va();
setAllowSleeping(bodySettingsVa, allow);
}
/**
* Alter the collision group to which the body will belong. (native
* attribute: mCollisionGroup)
*
* @param group the desired group (not null, unaffected)
*/
public void setCollisionGroup(CollisionGroup group) {
long bodySettingsVa = va();
long groupVa = group.va();
setCollisionGroup(bodySettingsVa, groupVa);
}
/**
* Alter the friction ratio. (native attribute: mFriction)
*
* @param friction the desired ratio (typically ≥0 and ≤1,
* default=0.2)
*/
public void setFriction(float friction) {
long bodySettingsVa = va();
setFriction(bodySettingsVa, friction);
}
/**
* Alter the gravity multiplier. (native attribute: mGravityFactor)
*
* @param factor the desired multiplier (default=1)
*/
public void setGravityFactor(float factor) {
long bodySettingsVa = va();
setGravityFactor(bodySettingsVa, factor);
}
/**
* Alter the linear damping constant. (native attribute: mLinearDamping)
*
* @param damping the desired value (in units of 1 per second, ≥0, ≤1,
* default=0.1)
*/
public void setLinearDamping(float damping) {
long bodySettingsVa = va();
setLinearDamping(bodySettingsVa, damping);
}
/**
* Alter the maximum speed of vertices. (native attribute: mMaxSpeed)
*
* @param maxSpeed the desired maximum speed (in meters per second, ≥0,
* default=500)
*/
public void setMaxLinearVelocity(float maxSpeed) {
long bodySettingsVa = va();
setMaxLinearVelocity(bodySettingsVa, maxSpeed);
}
/**
* Alter the number of solver iterations. (native attribute: mNumIterations)
*
* @param numIterations the desired number of iterations
*/
public void setNumIterations(int numIterations) {
long bodySettingsVa = va();
setNumIterations(bodySettingsVa, numIterations);
}
/**
* Alter the object layer. (native attribute: mObjectLayer)
*
* @param objLayer the ID of the desired object layer (≥0,
* <numObjectLayers, default=0)
*/
public void setObjectLayer(int objLayer) {
long bodySettingsVa = va();
setObjectLayer(bodySettingsVa, objLayer);
}
/**
* Alter the (initial) location of the body's origin. (native attribute:
* mPosition)
*
* @param location the desired location (in physics-system coordinates, not
* null, unaffected, default=(0,0,0))
*/
public void setPosition(RVec3Arg location) {
long bodySettingsVa = va();
double xx = location.xx();
double yy = location.yy();
double zz = location.zz();
setPosition(bodySettingsVa, xx, yy, zz);
}
/**
* Alter the pressure. (native attribute: mPressure)
*
* @param pressure the desired pressure (default=0)
*/
public void setPressure(float pressure) {
long bodySettingsVa = va();
setPressure(bodySettingsVa, pressure);
}
/**
* Alter the restitution ratio for collisions. (native attribute:
* mRestitution)
*
* @param restitution the desired ratio (typically ≥0 and ≤1,
* default=0)
*/
public void setRestitution(float restitution) {
long bodySettingsVa = va();
setRestitution(bodySettingsVa, restitution);
}
/**
* Alter the (initial) orientation. (native attribute: mRotation)
*
* @param orientation the desired location (relative to system axes, not
* null, unaffected, default=(0,0,0,1))
*/
public void setRotation(QuatArg orientation) {
long bodySettingsVa = va();
float qw = orientation.getW();
float qx = orientation.getX();
float qy = orientation.getY();
float qz = orientation.getZ();
setRotation(bodySettingsVa, qx, qy, qz, qw);
}
// *************************************************************************
// ConstSoftBodyCreationSettings methods
/**
* Test whether the created body will be allowed to fall asleep. The
* settings are unaffected. (native attribute: mAllowSleeping)
*
* @return {@code true} if allowed, otherwise {@code false}
*/
@Override
public boolean getAllowSleeping() {
long bodySettingsVa = va();
boolean result = getAllowSleeping(bodySettingsVa);
return result;
}
/**
* Return the friction ratio. The settings are unaffected. (native
* attribute: mFriction)
*
* @return the ratio (typically ≥0 and ≤1)
*/
@Override
public float getFriction() {
long bodySettingsVa = va();
float result = getFriction(bodySettingsVa);
return result;
}
/**
* Return the gravity factor. The settings are unaffected. (native
* attribute: mGravityFactor)
*
* @return the factor
*/
@Override
public float getGravityFactor() {
long bodySettingsVa = va();
float result = getGravityFactor(bodySettingsVa);
return result;
}
/**
* Return the linear damping constant. The settings are unaffected. (native
* attribute: mLinearDamping)
*
* @return the constant (in units of per second, ≥0, ≤1)
*/
@Override
public float getLinearDamping() {
long bodySettingsVa = va();
float result = getLinearDamping(bodySettingsVa);
return result;
}
/**
* Return the maximum linear speed. The settings are unaffected. (native
* attribute: mMaxSpeed)
*
* @return the maximum speed (in meters per second)
*/
@Override
public float getMaxLinearVelocity() {
long bodySettingsVa = va();
float result = getMaxLinearVelocity(bodySettingsVa);
return result;
}
/**
* Return the index of the object layer. The settings are unaffected.
* (native attribute: mObjectLayer)
*
* @return the layer index (≥0, <numObjectLayers)
*/
@Override
public int getObjectLayer() {
long bodySettingsVa = va();
int result = getObjectLayer(bodySettingsVa);
return result;
}
/**
* Return the (initial) location. The settings are unaffected. (native
* attribute: mPosition)
*
* @return a new location vector (in physics-system coordinates, all
* components finite)
*/
@Override
public RVec3 getPosition() {
long bodySettingsVa = va();
double xx = getPositionX(bodySettingsVa);
assert Double.isFinite(xx) : "xx = " + xx;
double yy = getPositionY(bodySettingsVa);
assert Double.isFinite(yy) : "yy = " + yy;
double zz = getPositionZ(bodySettingsVa);
assert Double.isFinite(zz) : "zz = " + zz;
RVec3 result = new RVec3(xx, yy, zz);
return result;
}
/**
* Return the pressure. The settings are unaffected. (native attribute:
* mPressure)
*
* @return the pressure
*/
@Override
public float getPressure() {
long bodySettingsVa = va();
float result = getPressure(bodySettingsVa);
return result;
}
/**
* Return the restitution ratio. The settings are unaffected. (native
* attribute: mRestitution)
*
* @return the ratio (typically ≥0 and ≤1)
*/
@Override
public float getRestitution() {
long bodySettingsVa = va();
float result = getRestitution(bodySettingsVa);
return result;
}
/**
* Copy the (initial) orientation of the body's axes. The settings are
* unaffected. (native attribute: mRotation)
*
* @return a new rotation quaternion (relative to the physics-system axes)
*/
@Override
public Quat getRotation() {
long bodySettingsVa = va();
float qw = getRotationW(bodySettingsVa);
float qx = getRotationX(bodySettingsVa);
float qy = getRotationY(bodySettingsVa);
float qz = getRotationZ(bodySettingsVa);
Quat result = new Quat(qx, qy, qz, qw);
return result;
}
// *************************************************************************
// native private methods
native private static long createDefault();
native private static long createFromSharedSettings(
long sharedSettingsVa, double xx, double yy, double zz, float qx,
float qy, float qz, float qw, int objectLayer);
native private static void free(long bodySettingsVa);
native private static boolean getAllowSleeping(long bodySettingsVa);
native private static float getFriction(long bodySettingsVa);
native private static float getGravityFactor(long bodySettingsVa);
native private static float getLinearDamping(long bodySettingsVa);
native private static float getMaxLinearVelocity(long bodySettingsVa);
native private static int getObjectLayer(long bodySettingsVa);
native private static double getPositionX(long bodySettingsVa);
native private static double getPositionY(long bodySettingsVa);
native private static double getPositionZ(long bodySettingsVa);
native private static float getPressure(long bodySettingsVa);
native private static float getRestitution(long bodySettingsVa);
native private static float getRotationW(long bodySettingsVa);
native private static float getRotationX(long bodySettingsVa);
native private static float getRotationY(long bodySettingsVa);
native private static float getRotationZ(long bodySettingsVa);
native private static void setAllowSleeping(
long bodySettingsVa, boolean allow);
native private static void setCollisionGroup(
long bodySettingsVa, long groupVa);
native private static void setFriction(long bodySettingsVa, float friction);
native private static void setGravityFactor(
long bodySettingsVa, float factor);
native private static void setLinearDamping(
long bodySettingsVa, float damping);
native private static void setMaxLinearVelocity(
long bodySettingsVa, float maxSpeed);
native private static void setNumIterations(
long bodySettingsVa, int numIterations);
native private static void setObjectLayer(
long bodySettingsVa, int objLayer);
native private static void setPosition(
long bodySettingsVa, double xx, double yy, double zz);
native private static void setPressure(long bodySettingsVa, float pressure);
native private static void setRestitution(
long bodySettingsVa, float restitution);
native private static void setRotation(
long bodySettingsVa, float qx, float qy, float qz, float qw);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy