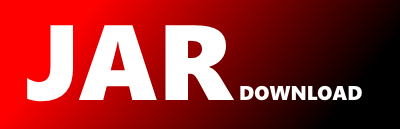
com.inamik.text.tables.cell.base.FunctionWithCharAndWidth Maven / Gradle / Ivy
The newest version!
/*
* iNamik Text Tables for Java
*
* Copyright (C) 2016 David Farrell ([email protected])
*
* Licensed under The MIT License (MIT), see LICENSE.txt
*/
package com.inamik.text.tables.cell.base;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
public abstract class FunctionWithCharAndWidth
{
public abstract Collection apply(Character character, Integer width, Collection cell);
public static final FunctionWithCharAndWidth IDENTITY = new FunctionWithCharAndWidth() {
@Override
public FunctionWithWidth withChar(char _1) {return FunctionWithWidth.IDENTITY; }
@Override
public FunctionWithChar withWidth(int _1) {
return FunctionWithChar.IDENTITY;
}
@Override
public Collection apply(Character _1, Integer _2, Collection cell) {
return cell;
}
};
/*
* From line.FunctionWithCharAndWidth
*/
public static FunctionWithCharAndWidth from(final com.inamik.text.tables.line.base.FunctionWithCharAndWidth f) {
return new FunctionWithCharAndWidth() {
@Override
public FunctionWithWidth withChar(char character) {
// lift(curry(f, character))
//
return FunctionWithWidth.from(f.withChar(character));
}
@Override
public FunctionWithChar withWidth(int width) {
// lift(curry_2(f, character))
//
return FunctionWithChar.from(f.withWidth(width));
}
@Override
public Collection apply(Character character, Integer width, Collection cell) {
// map(cell, curry(curry(f, character), width))
//
final List r = new ArrayList(cell.size());
for (String line: cell) { r.add(f.apply(character, width, line)); }
return Collections.unmodifiableCollection(r);
}
};
}
public FunctionWithWidth withChar(final char character) {
// curry(this, character)
//
final FunctionWithCharAndWidth f = this;
return new FunctionWithWidth() {
@Override
public Collection apply(Integer width, Collection cell) {
return f.apply(character, width, cell);
}
};
}
public FunctionWithChar withWidth(final int width) {
// curry_2(this, width)
//
final FunctionWithCharAndWidth f = this;
return new FunctionWithChar() {
@Override
public Collection apply(Character character, Collection cell) {
return f.apply(character, width, cell);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy