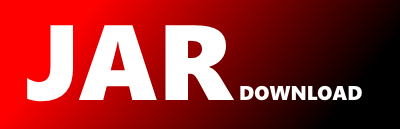
com.github.stormbit.vksdk.longpoll.updateshandlers.UpdatesHandlerGroup.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vk-bot-sdk-kotlin Show documentation
Show all versions of vk-bot-sdk-kotlin Show documentation
The Kotlin library for working with VK api
The newest version!
package com.github.stormbit.vksdk.longpoll.updateshandlers
import com.github.stormbit.vksdk.clients.Client
import com.github.stormbit.vksdk.events.*
import com.github.stormbit.vksdk.events.chat.*
import com.github.stormbit.vksdk.events.message.ChatMessageEvent
import com.github.stormbit.vksdk.events.message.CommunityMessageEvent
import com.github.stormbit.vksdk.events.message.UserMessageEvent
import com.github.stormbit.vksdk.longpoll.events.Events
import com.github.stormbit.vksdk.objects.Message
import com.github.stormbit.vksdk.objects.models.MessageEvent
import com.github.stormbit.vksdk.objects.models.ServiceAction
import com.github.stormbit.vksdk.utils.*
import com.github.stormbit.vksdk.utils.json
import com.github.stormbit.vksdk.vkapi.RoutePath
import kotlinx.serialization.json.JsonObject
import kotlinx.serialization.json.jsonObject
import kotlinx.serialization.json.jsonPrimitive
import com.github.stormbit.vksdk.objects.models.Message as MessageModel
class UpdatesHandlerGroup(private val client: Client) : UpdatesHandler() {
override suspend fun handleCurrentUpdate(currentUpdate: JsonObject) {
val event = currentUpdate.getValue("type").jsonPrimitive.content
val obj = currentUpdate.getValue("object").jsonObject
val updateType = Events.fromString(event)
when (updateType) {
Events.MESSAGE_NEW -> {
// check if message is received
handleMessageUpdate(obj)
// handle every
handleEveryLongPollUpdate(obj)
}
Events.MESSAGE_EVENT -> {
handleMessageEvent(obj)
// handle every
handleEveryLongPollUpdate(currentUpdate)
}
else -> handleEveryLongPollUpdate(currentUpdate)
}
}
private suspend fun handleEveryLongPollUpdate(obj: JsonObject) {
events[Events.EVERY.value]?.invoke(EveryEvent(obj))
}
/**
* Handle chat events
*/
private suspend fun handleChatEvents(messageModel: MessageModel, message: Message) {
try {
if (!messageModel.isServiceAction) return
val action = messageModel.serviceAction!!
val fromId = messageModel.fromId
val chatId = messageModel.peerId
val event = when (action) {
is ServiceAction.ChatCreate -> ChatCreateEvent(
"",
fromId,
chatId,
message
)
is ServiceAction.ChatTitleUpdate -> ChatTitleUpdateEvent(
null,
action.newTitle,
fromId,
chatId,
message
)
is ServiceAction.ChatPhotoUpdate -> {
val photo = messageModel.attachments[0].photo!!
ChatPhotoUpdateEvent(
photo.attachmentString,
fromId,
chatId,
message
)
}
is ServiceAction.ChatInviteUser -> ChatJoinEvent(
fromId,
action.memberId,
chatId,
message
)
is ServiceAction.ChatKickUser -> ChatLeaveEvent(
fromId,
action.memberId,
chatId,
message
)
is ServiceAction.ChatPhotoRemove -> ChatPhotoRemoveEvent(
fromId,
chatId,
message
)
is ServiceAction.ChatPinMessage -> ChatPinMessageEvent(
action.memberId,
chatId,
action.conversationMessageId,
message
)
is ServiceAction.ChatUnpinMessage -> ChatUnpinMessageEvent(
action.memberId,
chatId,
action.conversationMessageId,
message
)
is ServiceAction.ChatInviteUserByLink -> ChatInviteUserByLinkEvent(
action.memberId,
message
)
is ServiceAction.ChatInviteUserByCall -> ChatInviteUserByCallEvent(
action.memberId,
message
)
is ServiceAction.ChatScreenshot -> ChatScreenshotEvent(fromId, message)
is ServiceAction.ChatGroupCallInProgress -> ChatGroupCallInProgressEvent(message)
}
client.messageHandler?.pass(event, RoutePath(message.text))
} catch (e: Exception) {
log.error("Some error occurred when parsing chat event, error is: ", e)
}
}
/**
* Handle new message
*/
private suspend fun handleMessageUpdate(updateObject: JsonObject) {
try {
val messageObject = json.decodeFromJsonElement(MessageModel.serializer(), updateObject)
val message = Message(messageObject)
if (messageObject.serviceAction != null) {
handleChatEvents(messageObject, message)
} else {
val event = when (message.senderType) {
Message.SenderType.COMMUNITY -> CommunityMessageEvent(message, message.peerId)
Message.SenderType.USER -> UserMessageEvent(message, message.peerId)
Message.SenderType.CHAT -> ChatMessageEvent(message, message.peerId)
}
client.messageHandler?.pass(event, RoutePath(message.text))
}
} catch (e: Exception) {
log.error("Some error occurred when parsing message event, error is: ", e)
}
}
/**
* Handle message event
*/
private suspend fun handleMessageEvent(updateObject: JsonObject) {
val messageEvent = json.decodeFromJsonElement(MessageEvent.serializer(), updateObject)
events[Events.MESSAGE_EVENT.value]?.invoke(messageEvent)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy