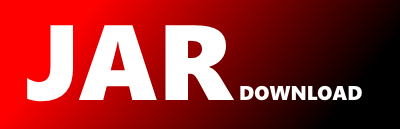
com.github.stormbit.vksdk.utils.Utils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vk-bot-sdk-kotlin Show documentation
Show all versions of vk-bot-sdk-kotlin Show documentation
The Kotlin library for working with VK api
The newest version!
package com.github.stormbit.vksdk.utils
import com.github.stormbit.vksdk.clients.Client
import com.github.stormbit.vksdk.objects.Chat
import com.github.stormbit.vksdk.objects.Message
import com.github.stormbit.vksdk.objects.models.Address
import com.github.stormbit.vksdk.vkapi.UploadableFile
import com.github.stormbit.vksdk.vkapi.execute
import com.github.stormbit.vksdk.vkapi.methods.Attachment
import com.github.stormbit.vksdk.vkapi.methods.Media
import io.ktor.client.request.forms.*
import io.ktor.http.*
import io.ktor.util.date.*
import io.ktor.utils.io.core.*
import kotlinx.serialization.KSerializer
import kotlinx.serialization.builtins.MapSerializer
import kotlinx.serialization.json.*
import kotlin.math.pow
internal fun regexSearch(pattern: Regex, string: String, group: Int = 0): String? {
return pattern.find(string)?.groups?.get(group)?.value
}
fun String.toJsonObject() = Json.encodeToJsonElement(this).jsonObject
fun Map<*, *>.toJsonObject() = JsonObject(map {
it.key.toString() to it.value.toJsonElement()
}.toMap())
fun Any?.toJsonElement(): JsonElement = when (this) {
null -> JsonNull
is Number -> JsonPrimitive(this)
is String -> JsonPrimitive(this)
is Boolean -> JsonPrimitive(this)
is Map<*, *> -> this.toJsonObject()
is Iterable<*> -> JsonArray(this.map { it.toJsonElement() })
is Array<*> -> JsonArray(this.map { it.toJsonElement() })
else -> JsonPrimitive(this.toString())
}
internal val json = Json {
encodeDefaults = false
ignoreUnknownKeys = true
isLenient = true
allowStructuredMapKeys = true
prettyPrint = false
useArrayPolymorphism = false
}
internal const val VK_API_VERSION = 5.122
internal const val BASE_API_URL = "https://api.vk.com/method/"
internal fun Address.Timetable.serialize(): String = json.encodeToString(Address.Timetable.serializer(), this)
inline val Media.mediaString get() = buildString {
append(ownerId)
append("_").append(id)
if (accessKey != null) append("_").append(accessKey!!)
}
inline val Attachment.attachmentString get() = buildString {
append(typeAttachment.value)
append(mediaString)
}
internal inline val GMTDate.unixTime: Int
get() = (GMTDate(seconds, minutes, hours, dayOfMonth, month, year).timestamp / 1000).toInt()
fun GMTDate.toDMYString(): String = buildString {
append(dayOfMonth.toString().padStart(2, '0'))
append((month.ordinal + 1).toString().padStart(2, '0'))
append(year.toString().padStart(4, '0'))
}
internal inline val Pair.formPart: FormPart
get() = FormPart(first, second, Headers.Empty)
internal inline val UploadableFile.formPart: FormPart
get() = FormPart(key, ByteReadPacket(content.bytes), content.filename.filenameHeader)
private inline val String.filenameHeader: Headers
get() = headersOf(HttpHeaders.ContentDisposition, "filename=$this")
infix fun Int.pow(component: Int) = toDouble().pow(component.toDouble()).toInt()
fun JsonObject.getString(key: String): String? = this[key]?.jsonPrimitive?.content
fun JsonObject.getInt(key: String): Int? = this[key]?.jsonPrimitive?.int
fun JsonObject.getLong(key: String): Long? = this[key]?.jsonPrimitive?.long
fun JsonObject.getBoolean(key: String): Boolean? = this[key]?.jsonPrimitive?.boolean
fun JsonObject.getJsonArray(key: String): JsonArray? = this[key]?.jsonArray
fun JsonObject.getJsonObject(key: String): JsonObject? = this[key]?.jsonObject
fun JsonArray.getString(index: Int): String = this[index].jsonPrimitive.content
fun JsonArray.getInt(index: Int): Int = this[index].jsonPrimitive.int
fun JsonArray.getLong(index: Int): Long = this[index].jsonPrimitive.long
fun JsonArray.getBoolean(index: Int): Boolean = this[index].jsonPrimitive.boolean
fun JsonArray.getJsonArray(index: Int): JsonArray = this[index].jsonArray
fun JsonArray.getJsonObject(index: Int): JsonObject = this[index].jsonObject
inline val Int.peerIdToGroupId: Int get() = -this
inline val Int.peerIdToChatId: Int get() = this - Chat.CHAT_PREFIX
inline val Int.groupIdToPeerId: Int get() = -this
inline val Int.chatIdToPeerId: Int get() = this + Chat.CHAT_PREFIX
inline val Int.isGroupId: Boolean get() = this < 0
inline val Int.isChatPeerId: Boolean get() = this > Chat.CHAT_PREFIX
inline val Int.isEmailPeerId: Boolean get() = this < 0 && (-this).isChatPeerId
inline val Int.isUserPeerId: Boolean get() = !isGroupId && !isChatPeerId && !isEmailPeerId
fun Boolean.toInt() = if (this) 1 else 0
fun Int.toBoolean() = this > 0
@Suppress("NOTHING_TO_INLINE")
internal inline fun ParametersBuilder.append(key: String, value: Any?) {
if (value != null && value.toString().isNotEmpty()) append(key, value.toString())
}
@Suppress("NOTHING_TO_INLINE")
inline fun map(key: KSerializer, value: KSerializer) = MapSerializer(key, value)
suspend inline fun Message.send(client: Client): Int = client.messages.send(
peerId = peerId,
randomId = randomId,
text = text,
stickerId = stickerId,
payload = payload,
attachments = attachmentsToSend,
keyboard = keyboard,
replyToMessageId = replyTo,
forwardedMessages = forwardedMessagesToSend
).execute()
internal suspend fun Client.getClientId(): Int {
val response = users.get().execute()
return response[0].id
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy