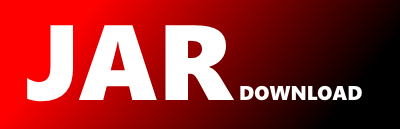
com.greenpepper.server.domain.Execution Maven / Gradle / Ivy
package com.greenpepper.server.domain;
import com.greenpepper.report.XmlReport;
import com.greenpepper.util.FormatedDate;
import com.greenpepper.util.HtmlUtil;
import com.greenpepper.util.StringUtil;
import org.hibernate.annotations.Index;
import javax.persistence.*;
import java.sql.Timestamp;
import java.util.Vector;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.*;
import static org.apache.commons.lang3.StringUtils.defaultString;
@Entity
/**
* Execution class.
*
* @author oaouattara
* @version $Id: $Id
*/
@Table(name="EXECUTION")
@SuppressWarnings("serial")
public class Execution extends AbstractUniqueEntity implements Comparable
{
/** Constant NOT_RUNNED="notrunned"
*/
public static final String NOT_RUNNED = "notrunned";
/** Constant IGNORED="ignored"
*/
public static final String IGNORED = "ignored";
/** Constant SUCCESS="success"
*/
public static final String SUCCESS = "success";
/** Constant FAILED="error"
*/
public static final String FAILED = "error";
private Specification specification;
private SystemUnderTest systemUnderTest;
private String sections;
private String results;
private String stdoutLogs;
private String stderrLogs;
private int success = 0;
private int failures = 0;
private int errors = 0;
private int ignored = 0;
private String executionErrorId;
private Timestamp executionDate;
private boolean executedRemotely;
/**
* none.
*
* @return a {@link com.greenpepper.server.domain.Execution} object.
*/
public static Execution none()
{
return new Execution();
}
/**
* error.
*
* @param specification a {@link com.greenpepper.server.domain.Specification} object.
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
* @param sections a {@link java.lang.String} object.
* @param errorId a {@link java.lang.String} object.
* @return a {@link com.greenpepper.server.domain.Execution} object.
*/
public static Execution error(Specification specification, SystemUnderTest systemUnderTest, String sections, String errorId)
{
Execution execution = new Execution();
execution.setSystemUnderTest(systemUnderTest);
execution.setExecutionDate(new Timestamp(System.currentTimeMillis()));
execution.setSpecification(specification);
execution.setExecutionErrorId(errorId);
execution.setSections(sections);
return execution;
}
/**
* newInstance.
*
* @param specification a {@link com.greenpepper.server.domain.Specification} object.
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
* @param xmlReport a {@link com.greenpepper.report.XmlReport} object.
* @return a {@link com.greenpepper.server.domain.Execution} object.
*/
public static Execution newInstance(Specification specification, SystemUnderTest systemUnderTest, XmlReport xmlReport)
{
if(xmlReport.getGlobalException() != null)
{
return error(specification, systemUnderTest, null, xmlReport.getGlobalException());
}
Execution execution = new Execution();
execution.setExecutionDate(new Timestamp(System.currentTimeMillis()));
execution.setSpecification(specification);
execution.setSystemUnderTest(systemUnderTest);
execution.setFailures( xmlReport.getFailure(0) );
execution.setErrors( xmlReport.getError(0) );
execution.setSuccess( xmlReport.getSuccess(0) );
execution.setIgnored( xmlReport.getIgnored(0) );
String results = xmlReport.getResults(0);
if(results != null)
{
execution.setResults(results);
}
if (xmlReport.getSections(0) != null)
{
StringBuilder sections = new StringBuilder();
int index = 0;
while (xmlReport.getSections(index) != null)
{
if (index > 0) sections.append(',');
sections.append(xmlReport.getSections(index));
index++;
}
execution.setSections(sections.toString());
}
return execution;
}
/**
* newInstance.
*
* @param specification a {@link com.greenpepper.server.domain.Specification} object.
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
* @param xmlReport a {@link com.greenpepper.report.XmlReport} object.
* @param sections a {@link java.lang.String} object.
* @return a {@link com.greenpepper.server.domain.Execution} object.
*/
public static Execution newInstance(Specification specification, SystemUnderTest systemUnderTest, XmlReport xmlReport, String sections)
{
Execution execution = Execution.newInstance(specification, systemUnderTest, xmlReport );
execution.setSections(sections);
return execution;
}
/**
* Getter for the field specification
.
*
* @return a {@link com.greenpepper.server.domain.Specification} object.
*/
@ManyToOne( cascade = {CascadeType.PERSIST, CascadeType.MERGE} )
@JoinColumn(name="SPECIFICATION_ID", nullable = false)
public Specification getSpecification()
{
return specification;
}
/**
* Getter for the field systemUnderTest
.
*
* @return a {@link com.greenpepper.server.domain.SystemUnderTest} object.
*/
@ManyToOne( cascade = {CascadeType.PERSIST, CascadeType.MERGE} )
@JoinColumn(name="SUT_ID", nullable = false)
public SystemUnderTest getSystemUnderTest()
{
return systemUnderTest;
}
/**
* Getter for the field sections
.
*
* @return a {@link java.lang.String} object.
*/
@Basic
@Column(name = "SECTIONS", length=50)
public String getSections()
{
return sections;
}
/**
* Getter for the field results
.
*
* @return a {@link java.lang.String} object.
*/
@Lob
@Column(name = "RESULTS", length = 2147483647)
public String getResults()
{
return results;
}
/**
* Getter for the field executionErrorId
.
*
* @return a {@link java.lang.String} object.
*/
@Lob
@Column(name = "ERRORID", length = 2147483647)
public String getExecutionErrorId()
{
return executionErrorId;
}
/**
* Getter for the field success
.
*
* @return a int.
*/
@Basic
@Column(name = "SUCCESS_COUNT")
public int getSuccess()
{
return success;
}
/**
* Getter for the field ignored
.
*
* @return a int.
*/
@Basic
@Column(name = "IGNORED_COUNT")
public int getIgnored()
{
return ignored;
}
/**
* Getter for the field failures
.
*
* @return a int.
*/
@Basic
@Column(name = "FAILURES_COUNT")
public int getFailures()
{
return failures;
}
/**
* Getter for the field errors
.
*
* @return a int.
*/
@Basic
@Column(name = "ERRORS_COUNT")
public int getErrors()
{
return errors;
}
/**
* Getter for the field executionDate
.
*
* @return a {@link java.sql.Timestamp} object.
*/
@Basic
@Column(name = "EXECUTION_DATE")
@Index(name="executionDateIndex")
public Timestamp getExecutionDate()
{
return executionDate;
}
/**
* Setter for the field specification
.
*
* @param specification a {@link com.greenpepper.server.domain.Specification} object.
*/
public void setSpecification(Specification specification)
{
this.specification = specification;
}
/**
* Setter for the field systemUnderTest
.
*
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
*/
public void setSystemUnderTest(SystemUnderTest systemUnderTest)
{
this.systemUnderTest = systemUnderTest;
}
/**
* Setter for the field sections
.
*
* @param sections a {@link java.lang.String} object.
*/
public void setSections(String sections)
{
this.sections = sections;
}
/**
* Setter for the field results
.
*
* @param results a {@link java.lang.String} object.
*/
public void setResults(String results)
{
this.results = results;
}
/**
* Setter for the field executionErrorId
.
*
* @param executionErrorId a {@link java.lang.String} object.
*/
public void setExecutionErrorId(String executionErrorId)
{
this.executionErrorId = executionErrorId;
}
/**
* Setter for the field success
.
*
* @param success a int.
*/
public void setSuccess(int success)
{
this.success = success;
}
/**
* Setter for the field ignored
.
*
* @param ignored a int.
*/
public void setIgnored(int ignored)
{
this.ignored = ignored;
}
/**
* Setter for the field failures
.
*
* @param failures a int.
*/
public void setFailures(int failures)
{
this.failures = failures;
}
/**
* Setter for the field errors
.
*
* @param errors a int.
*/
public void setErrors(int errors)
{
this.errors = errors;
}
/**
* Setter for the field executionDate
.
*
* @param executionDate a {@link java.sql.Timestamp} object.
*/
public void setExecutionDate(Timestamp executionDate)
{
this.executionDate = executionDate;
}
/**
* hasException.
*
* @return a boolean.
*/
public boolean hasException()
{
return !StringUtil.isEmpty(executionErrorId);
}
/**
* hasFailed.
*
* @return a boolean.
*/
public boolean hasFailed()
{
return hasException() || failures + errors > 0;
}
/**
* hasSucceeded.
*
* @return a boolean.
*/
public boolean hasSucceeded()
{
return !hasFailed() && success > 0;
}
/**
* wasIgnored.
*
* @return a boolean.
*/
public boolean wasIgnored()
{
return !hasFailed() && !hasSucceeded() && ignored != 0;
}
/**
* wasRunned.
*
* @return a boolean.
*/
public boolean wasRunned()
{
return hasFailed() || hasSucceeded() || ignored != 0;
}
/**
* wasRemotelyExecuted.
*
* @return a boolean.
*/
public boolean wasRemotelyExecuted()
{
return this.executedRemotely;
}
/**
* setRemotelyExecuted.
*/
public void setRemotelyExecuted()
{
this.executedRemotely = true;
}
@Transient
public String getStdoutLogs() {
return stdoutLogs;
}
@Transient
public String getStderrLogs() {
return stderrLogs;
}
public void setStdoutLogs(String stdoutLogs) {
this.stdoutLogs = stdoutLogs;
}
public void setStderrLogs(String stderrLogs) {
this.stderrLogs = stderrLogs;
}
/**
* getStatus.
*
* @return a {@link java.lang.String} object.
*/
@Transient
public String getStatus()
{
if(hasFailed()) return FAILED;
if(hasSucceeded()) return SUCCESS;
if(ignored != 0) return IGNORED;
return NOT_RUNNED;
}
/**
* getCleanedResults.
*
* @return a {@link java.lang.String} object.
*/
@Transient
public String getCleanedResults()
{
return HtmlUtil.cleanUpResults(results);
}
/**
* getFormatedExecutionDate.
*
* @return a {@link java.lang.String} object.
*/
@Transient
public String getFormatedExecutionDate()
{
FormatedDate d = new FormatedDate(executionDate);
return d.getFormatedTimestamp();
}
/**
* marshallize.
*
* @return a {@link java.util.Vector} object.
*/
public Vector