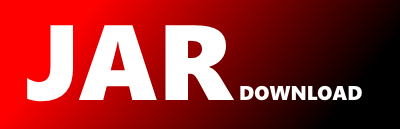
com.greenpepper.server.domain.Runner Maven / Gradle / Ivy
package com.greenpepper.server.domain;
import com.greenpepper.report.XmlReport;
import com.greenpepper.server.GreenPepperServerException;
import com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller;
import com.greenpepper.server.rpc.xmlrpc.client.XmlRpcClientExecutor;
import com.greenpepper.server.rpc.xmlrpc.client.XmlRpcClientExecutorFactory;
import com.greenpepper.util.*;
import com.greenpepper.util.cmdline.CommandLineBuilder;
import com.greenpepper.util.cmdline.CommandLineExecutor;
import org.hibernate.annotations.CollectionOfElements;
import org.hibernate.annotations.Sort;
import org.hibernate.annotations.SortType;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.persistence.*;
import java.io.File;
import java.io.IOException;
import java.util.*;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.*;
import static com.greenpepper.util.IOUtils.uniquePath;
import static org.apache.commons.lang3.StringUtils.defaultString;
/**
* Runner Class.
* Definition of a Runner.
*
* Copyright (c) 2006-2007 Pyxis technologies inc. All Rights Reserved.
*
* @author JCHUET
* @version $Id: $Id
*/
@Entity
@Table(name="RUNNER")
@SuppressWarnings("serial")
public class Runner extends AbstractVersionedEntity implements Comparable
{
private static final Logger LOG = LoggerFactory.getLogger(Runner.class);
private static final String AGENT_HANDLER = "greenpepper-agent1";
private String name;
private String cmdLineTemplate;
private String mainClass;
private EnvironmentType envType;
private String serverName;
private String serverPort;
private Boolean secured;
private SortedSet classpaths = new TreeSet();
/**
* newInstance.
*
* @param name a {@link java.lang.String} object.
* @return a {@link com.greenpepper.server.domain.Runner} object.
*/
public static Runner newInstance(String name)
{
Runner runner = new Runner();
runner.setName(name);
return runner;
}
/**
* Getter for the field name
.
*
* @return a {@link java.lang.String} object.
*/
@Basic
@Column(name = "NAME", unique = true, nullable = false, length=255)
public String getName()
{
return name;
}
/**
* getEnvironmentType.
*
* @return a {@link com.greenpepper.server.domain.EnvironmentType} object.
*/
@ManyToOne( cascade = {CascadeType.PERSIST, CascadeType.MERGE} )
@JoinColumn(name="ENVIRONMENT_TYPE_ID")
public EnvironmentType getEnvironmentType()
{
return envType;
}
/**
* Getter for the field serverName
.
*
* @return a {@link java.lang.String} object.
*/
@Basic
@Column(name = "SERVER_NAME", nullable = true, length=255)
public String getServerName()
{
return serverName;
}
/**
* Getter for the field serverPort
.
*
* @return a {@link java.lang.String} object.
*/
@Basic
@Column(name = "SERVER_PORT", nullable = true, length=8)
public String getServerPort()
{
return serverPort;
}
/**
* isSecured.
*
* @return a boolean.
*/
@Basic
@Column(name = "SECURED", nullable = true)
public boolean isSecured()
{
return secured != null && secured;
}
/**
* Getter for the field cmdLineTemplate
.
*
* @return a {@link java.lang.String} object.
*/
@Basic
@Column(name = "CMD_LINE_TEMPLATE", nullable = true, length=510)
public String getCmdLineTemplate()
{
return cmdLineTemplate;
}
/**
* Getter for the field mainClass
.
*
* @return a {@link java.lang.String} object.
*/
@Basic
@Column(name = "MAIN_CLASS", nullable = true, length=255)
public String getMainClass()
{
return mainClass;
}
/**
* Getter for the field classpaths
.
*
* @return a {@link java.util.SortedSet} object.
*/
@CollectionOfElements
@JoinTable( name="RUNNER_CLASSPATHS", joinColumns={@JoinColumn(name="RUNNER_ID")} )
@Column(name = "elt", nullable = true, length=255)
@Sort(type = SortType.COMPARATOR, comparator = ClasspathComparator.class)
public SortedSet getClasspaths()
{
return classpaths;
}
/**
* Setter for the field name
.
*
* @param name a {@link java.lang.String} object.
*/
public void setName(String name)
{
this.name = name;
}
/**
* setEnvironmentType.
*
* @param envType a {@link com.greenpepper.server.domain.EnvironmentType} object.
*/
public void setEnvironmentType(EnvironmentType envType)
{
this.envType = envType;
}
/**
* Setter for the field serverName
.
*
* @param serverName a {@link java.lang.String} object.
*/
public void setServerName(String serverName)
{
this.serverName = StringUtil.toNullIfEmpty(serverName);
}
/**
* Setter for the field serverPort
.
*
* @param serverPort a {@link java.lang.String} object.
*/
public void setServerPort(String serverPort)
{
this.serverPort = StringUtil.toNullIfEmpty(serverPort);
}
/**
* Setter for the field secured
.
*
* @param secured a {@link java.lang.Boolean} object.
*/
public void setSecured(Boolean secured)
{
this.secured = secured != null && secured;
}
/**
* Setter for the field cmdLineTemplate
.
*
* @param cmdLineTemplate a {@link java.lang.String} object.
*/
public void setCmdLineTemplate(String cmdLineTemplate)
{
this.cmdLineTemplate = StringUtil.toNullIfEmpty(cmdLineTemplate);
}
/**
* Setter for the field mainClass
.
*
* @param mainClass a {@link java.lang.String} object.
*/
public void setMainClass(String mainClass)
{
this.mainClass = StringUtil.toNullIfEmpty(mainClass);
}
/**
* Setter for the field classpaths
.
*
* @param classpaths a {@link java.util.SortedSet} object.
*/
public void setClasspaths(SortedSet classpaths)
{
this.classpaths = classpaths;
}
/**
* execute.
*
* @param specification a {@link com.greenpepper.server.domain.Specification} object.
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
* @param implementedVersion a boolean.
* @param sections a {@link java.lang.String} object.
* @param locale a {@link java.lang.String} object.
* @return a {@link com.greenpepper.server.domain.Execution} object.
*/
public Execution execute(Specification specification, SystemUnderTest systemUnderTest, boolean implementedVersion, String sections, String locale)
{
if(isRemote())
{
return executeRemotely(specification, systemUnderTest, implementedVersion, sections, locale);
}
else
{
return executeLocally(specification, systemUnderTest, implementedVersion, sections, locale);
}
}
@SuppressWarnings("unchecked")
protected Execution executeRemotely(Specification specification, SystemUnderTest systemUnderTest, boolean implementedVersion, String sections, String locale)
{
LOG.debug("Execute Remotely {} on agentURL {}", specification.getName(), agentUrl());
try
{
sections = defaultString(sections);
locale = defaultString(locale);
XmlRpcClientExecutor xmlrpc = XmlRpcClientExecutorFactory.newExecutor(agentUrl());
Vector params = CollectionUtil.toVector(marshallize(), systemUnderTest.marshallize(), specification.marshallize(), implementedVersion, sections, locale);
Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy