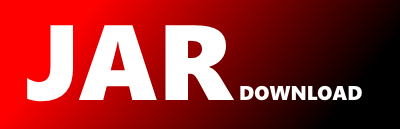
com.greenpepper.util.cmdline.CommandLineExecutor Maven / Gradle / Ivy
package com.greenpepper.util.cmdline;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import static org.apache.commons.lang3.StringUtils.isNotBlank;
/**
* CommandLineExecutor class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class CommandLineExecutor
{
private static Logger log = LoggerFactory.getLogger(CommandLineExecutor.class);
private static final int SUCCESS = 0;
private StreamGobbler gobbler;
private String[] cmdLine;
private String stdOutFile;
private String stdErrFile;
private boolean dontShowStdOut;
/**
* Constructor for CommandLineExecutor.
*
* @param cmdLine an array of {@link java.lang.String} objects.
*/
public CommandLineExecutor(String[] cmdLine)
{
this.cmdLine = cmdLine;
}
/**
* executeAndWait.
*
* @throws java.lang.Exception if any.
*/
public void executeAndWait() throws Exception
{
Process p = launchProcess();
checkForErrors(p);
if (log.isDebugEnabled())
{
// GP-551 : Keep trace of outputs
if (isNotBlank(getOutput()))
{
log.debug("System Output during execution : \n" + getOutput());
}
if (gobbler.hasErrors())
{
log.debug("System Error Output during execution : \n" + getError());
}
}
}
private Process launchProcess() throws Exception
{
log.debug("Launching cmd: {}", getCmdLineToString());
Process p = Runtime.getRuntime().exec(cmdLine);
gobbler = new StreamGobblerImpl(p, stdOutFile, stdErrFile, dontShowStdOut);
Thread reader = new Thread(gobbler);
reader.start();
p.waitFor();
return p;
}
public void setStdOutFile(String stdOutFile) {
this.stdOutFile = stdOutFile;
}
public void setStdErrFile(String stdErrFile) {
this.stdErrFile = stdErrFile;
}
/**
* getOutput.
*
* @return a {@link java.lang.String} object.
*/
public String getOutput()
{
return gobbler.getOutput();
}
public String getError() {
return gobbler.getError();
}
private void checkForErrors(Process p) throws Exception
{
if(p.exitValue() != SUCCESS)
{
if(gobbler.hasErrors())
{
throw new Exception(gobbler.getError());
}
throw new Exception("Process was terminated abnormally");
}
}
private String getCmdLineToString()
{
StringBuilder sb = new StringBuilder();
for(String cmd : cmdLine)
{
sb.append(cmd).append(" ");
}
return sb.toString();
}
public void setDontShowStdOut(boolean dontShowStdOut) {
this.dontShowStdOut = dontShowStdOut;
}
public boolean isDontShowStdOut() {
return dontShowStdOut;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy