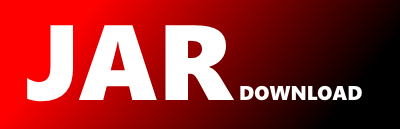
com.greenpepper.confluence.actions.SpecificationAction Maven / Gradle / Ivy
package com.greenpepper.confluence.actions;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.StringTokenizer;
import com.greenpepper.server.GreenPepperServerErrorKey;
import com.greenpepper.server.GreenPepperServerException;
import com.greenpepper.server.domain.Execution;
import com.greenpepper.server.domain.Project;
import com.greenpepper.server.domain.Reference;
import com.greenpepper.server.domain.Repository;
import com.greenpepper.server.domain.Requirement;
import com.greenpepper.server.domain.Runner;
import com.greenpepper.server.domain.Specification;
import com.greenpepper.server.domain.SystemUnderTest;
import com.greenpepper.util.ExceptionUtils;
import com.greenpepper.util.HtmlUtil;
/**
* SpecificationAction class.
*
* @author oaouattara
* @version $Id: $Id
*/
@SuppressWarnings("serial")
public class SpecificationAction extends AbstractGreenPepperAction
{
protected Specification specification;
private List references;
private List repositories;
private List projectSystemUnderTests;
private String selectedSystemUnderTestInfo;
private Execution execution;
private String requirementName;
private String sutName;
private String sutProjectName;
private String repositoryUid;
private String sections;
private boolean isMain;
private boolean isSutEditable;
protected boolean implemented;
/**
* loadSpecification.
*
* @return a {@link java.lang.String} object.
*/
public String loadSpecification()
{
try
{
specification = gpUtil.getSpecification(spaceKey, getPage().getTitle());
}
catch (GreenPepperServerException e)
{
if(!e.getId().equals(GreenPepperServerErrorKey.SPECIFICATION_NOT_FOUND))
addActionError(e.getId());
}
return SUCCESS;
}
/**
* updateSelectedSystemUndertTest.
*
* @return a {@link java.lang.String} object.
*/
public String updateSelectedSystemUndertTest()
{
gpUtil.saveSelectedSystemUnderTestInfo(page, selectedSystemUnderTestInfo);
return SUCCESS;
}
/**
* getSystemUndertTestSelection.
*
* @return a {@link java.lang.String} object.
*/
public String getSystemUndertTestSelection()
{
try
{
specification = gpUtil.getSpecification(spaceKey, getPage().getTitle());
projectSystemUnderTests = gpUtil.getSystemsUnderTests(spaceKey);
}
catch (GreenPepperServerException e)
{
projectSystemUnderTests = new ArrayList();
addActionError(e.getId());
}
return SUCCESS;
}
/**
* addSystemUnderTest.
*
* @return a {@link java.lang.String} object.
*/
public String addSystemUnderTest()
{
try
{
SystemUnderTest sut = SystemUnderTest.newInstance(sutName);
sut.setProject(Project.newInstance(sutProjectName));
sut.setRunner(Runner.newInstance(""));
Specification specification = Specification.newInstance(getPage().getTitle());
specification.setRepository(gpUtil.getHomeRepository(spaceKey));
gpUtil.getGPServerService().addSpecificationSystemUnderTest(sut, specification);
}
catch (GreenPepperServerException e)
{
addActionError(e.getId());
}
return getSystemUndertTestSelection();
}
/**
* removeSystemUnderTest.
*
* @return a {@link java.lang.String} object.
*/
public String removeSystemUnderTest()
{
try
{
SystemUnderTest sut = SystemUnderTest.newInstance(sutName);
sut.setProject(Project.newInstance(sutProjectName));
sut.setRunner(Runner.newInstance(""));
Specification specification = Specification.newInstance(getPage().getTitle());
specification.setRepository(gpUtil.getHomeRepository(spaceKey));
gpUtil.getGPServerService().removeSpecificationSystemUnderTest(sut, specification);
}
catch (GreenPepperServerException e)
{
addActionError(e.getId());
}
return getSystemUndertTestSelection();
}
/**
* run.
*
* @return a {@link java.lang.String} object.
*/
public String run()
{
String locale = getLocale().getLanguage();
SystemUnderTest sut = SystemUnderTest.newInstance(sutName);
sut.setProject(Project.newInstance(sutProjectName));
sut.setRunner(Runner.newInstance(""));
Specification spec = Specification.newInstance(getPage().getTitle());
try
{
spec.setRepository(gpUtil.getHomeRepository(spaceKey));
execution = gpUtil.getGPServerService().runSpecification(sut, spec, implemented, locale);
}
catch (GreenPepperServerException e)
{
execution = Execution.error(spec, sut, null, e.getId());
}
catch (Exception e)
{
execution = Execution.error(spec, sut, null, ExceptionUtils.stackTrace(e, "
", 15));
}
return SUCCESS;
}
/**
* retrieveReferenceList.
*
* @return a {@link java.lang.String} object.
*/
public String retrieveReferenceList()
{
try
{
references = gpUtil.getReferences(spaceKey, getPage().getTitle());
if(isEditMode)
{
repositories = gpUtil.getRepositories(spaceKey);
if(repositories.isEmpty())
throw new GreenPepperServerException("greenpepper.server.repositoriesnotfound", "");
projectSystemUnderTests = gpUtil.getSystemsUnderTests(spaceKey);
if(projectSystemUnderTests.isEmpty())
throw new GreenPepperServerException("greenpepper.server.sutsnotfound", "");
}
}
catch (GreenPepperServerException e)
{
addActionError(e.getId());
isEditMode = false;
}
return SUCCESS;
}
/**
* addReference.
*
* @return a {@link java.lang.String} object.
*/
public String addReference()
{
try
{
gpUtil.getGPServerService().createReference(instanceOfReference());
}
catch (GreenPepperServerException e)
{
addActionError(e.getId());
}
return retrieveReferenceList();
}
/**
* removeReference.
*
* @return a {@link java.lang.String} object.
*/
public String removeReference()
{
isEditMode = true;
try
{
gpUtil.getGPServerService().removeReference(instanceOfReference());
}
catch (GreenPepperServerException e)
{
addActionError(e.getId());
}
return retrieveReferenceList();
}
/**
* Getter for the field specification
.
*
* @return a {@link com.greenpepper.server.domain.Specification} object.
*/
public Specification getSpecification()
{
return specification;
}
/**
* Getter for the field repositories
.
*
* @return a {@link java.util.List} object.
*/
public List getRepositories()
{
return repositories;
}
/**
* Getter for the field projectSystemUnderTests
.
*
* @return a {@link java.util.List} object.
*/
public List getProjectSystemUnderTests()
{
return projectSystemUnderTests;
}
/**
* getSpecificationSystemUnderTests.
*
* @return a {@link java.util.Set} object.
*/
public Set getSpecificationSystemUnderTests()
{
if(specification == null) return new HashSet();
return specification.getTargetedSystemUnderTests();
}
/**
* Getter for the field isSutEditable
.
*
* @return a boolean.
*/
public boolean getIsSutEditable()
{
return this.isSutEditable;
}
/**
* Setter for the field isSutEditable
.
*
* @param isSutEditable a boolean.
*/
public void setIsSutEditable(boolean isSutEditable)
{
this.isSutEditable = isSutEditable;
}
/**
* Getter for the field implemented
.
*
* @return a boolean.
*/
public boolean getImplemented()
{
return implemented;
}
/**
* Setter for the field implemented
.
*
* @param implemented a boolean.
*/
public void setImplemented(boolean implemented)
{
this.implemented = implemented;
}
/**
* Getter for the field requirementName
.
*
* @return a {@link java.lang.String} object.
*/
public String getRequirementName()
{
return requirementName;
}
/**
* Setter for the field requirementName
.
*
* @param requirementName a {@link java.lang.String} object.
*/
public void setRequirementName(String requirementName)
{
this.requirementName = requirementName;
}
/**
* setSutInfo.
*
* @param sutInfo a {@link java.lang.String} object.
*/
public void setSutInfo(String sutInfo)
{
StringTokenizer stk = new StringTokenizer(sutInfo, "@");
this.sutProjectName = stk.nextToken();
this.sutName = stk.nextToken();
}
/**
* Setter for the field sutName
.
*
* @param sutName a {@link java.lang.String} object.
*/
public void setSutName(String sutName)
{
this.sutName = sutName;
}
/**
* Setter for the field sutProjectName
.
*
* @param sutProjectName a {@link java.lang.String} object.
*/
public void setSutProjectName(String sutProjectName)
{
this.sutProjectName = sutProjectName;
}
/**
* Setter for the field repositoryUid
.
*
* @param repositoryUid a {@link java.lang.String} object.
*/
public void setRepositoryUid(String repositoryUid)
{
this.repositoryUid = repositoryUid;
}
/**
* Setter for the field sections
.
*
* @param sections a {@link java.lang.String} object.
*/
public void setSections(String sections)
{
this.sections = sections.trim();
}
/**
* Getter for the field references
.
*
* @return a {@link java.util.List} object.
*/
public List getReferences()
{
return references;
}
/**
* getSelectedSystemUnderTest.
*
* @return a {@link com.greenpepper.server.domain.SystemUnderTest} object.
*/
public SystemUnderTest getSelectedSystemUnderTest()
{
return gpUtil.getSelectedSystemUnderTest(page);
}
/**
* getIsExecutable.
*
* @return a boolean.
*/
public boolean getIsExecutable()
{
return specification != null;
}
/**
* Getter for the field execution
.
*
* @return a {@link com.greenpepper.server.domain.Execution} object.
*/
public Execution getExecution()
{
return execution;
}
/**
* Getter for the field selectedSystemUnderTestInfo
.
*
* @return a {@link java.lang.String} object.
*/
public String getSelectedSystemUnderTestInfo()
{
if(selectedSystemUnderTestInfo != null){return selectedSystemUnderTestInfo;}
selectedSystemUnderTestInfo = gpUtil.getSelectedSystemUnderTestInfo(page);
return selectedSystemUnderTestInfo;
}
/**
* Setter for the field selectedSystemUnderTestInfo
.
*
* @param selectedSystemUnderTestInfo a {@link java.lang.String} object.
*/
public void setSelectedSystemUnderTestInfo(String selectedSystemUnderTestInfo)
{
this.selectedSystemUnderTestInfo = selectedSystemUnderTestInfo;
}
/**
* Getter for the field isMain
.
*
* @return a boolean.
*/
public boolean getIsMain()
{
return isMain;
}
/**
* Setter for the field isMain
.
*
* @param isMain a boolean.
*/
public void setIsMain(boolean isMain)
{
this.isMain = isMain;
}
/**
* getRenderedResults.
*
* @return a {@link java.lang.String} object.
*/
public String getRenderedResults()
{
String results = execution.getResults();
if (results != null)
{
results = results.replaceAll("greenpepper-manage-not-rendered", "greenpepper-manage");
results = results.replaceAll("greenpepper-hierarchy-not-rendered", "greenpepper-hierarchy");
results = results.replaceAll("greenpepper-children-not-rendered", "greenpepper-children");
results = results.replaceAll("greenpepper-labels-not-rendered", "greenpepper-labels");
results = results.replaceAll("greenpepper-group-not-rendered", "greenpepper-group");
results = results.replaceAll("Unknown macro:", "");
return HtmlUtil.cleanUpResults(results);
}
return null;
}
/**
* isInSpecificationSelection.
*
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
* @return a boolean.
*/
public boolean isInSpecificationSelection(SystemUnderTest systemUnderTest)
{
return gpUtil.isInSutList(systemUnderTest, specification.getTargetedSystemUnderTests());}
/********************* Utils *********************/
private Reference instanceOfReference()
throws GreenPepperServerException
{
SystemUnderTest sut = SystemUnderTest.newInstance(sutName);
sut.setProject(Project.newInstance(sutProjectName));
Specification specification = Specification.newInstance(getPage().getTitle());
specification.setRepository(gpUtil.getHomeRepository(spaceKey));
Requirement requirement = Requirement.newInstance(requirementName);
requirement.setRepository(Repository.newInstance(repositoryUid));
return Reference.newInstance(requirement, specification, sut, sections);
}
/**
* getNextFieldId.
*
* @return a int.
*/
public int getNextFieldId()
{
return getFieldId() + 1;
}
}