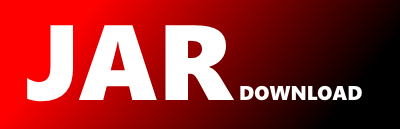
com.greenpepper.confluence.actions.execution.HeaderExecutionAction Maven / Gradle / Ivy
package com.greenpepper.confluence.actions.execution;
import java.util.LinkedList;
import java.util.List;
import com.atlassian.confluence.content.render.xhtml.ConversionContext;
import com.atlassian.confluence.content.render.xhtml.DefaultConversionContext;
import com.atlassian.confluence.core.ContentEntityObject;
import com.atlassian.confluence.pages.ContentTree;
import com.atlassian.confluence.pages.Page;
import com.atlassian.confluence.renderer.PageContext;
import com.greenpepper.server.GreenPepperServerException;
import com.greenpepper.server.domain.Specification;
import com.greenpepper.server.domain.SystemUnderTest;
/**
* HeaderExecutionAction class.
*
* @author oaouattara
* @version $Id: $Id
*/
@SuppressWarnings("serial")
public class HeaderExecutionAction extends ChildrenExecutionAction
{
private Boolean hasChildren;
private Boolean doExecuteChildren;
private boolean pepperize;
private boolean retrieveBody;
/**
* loadHeader.
*
* @return a {@link java.lang.String} object.
*/
public String loadHeader()
{
retrieveReferenceList();
loadSpecification();
return SUCCESS;
}
/**
* setAsImplemented.
*
* @return a {@link java.lang.String} object.
*/
public String setAsImplemented()
{
gpUtil.saveImplementedVersion(getPage(), getPage().getVersion());
return loadHeader();
}
/**
* revert.
*
* @return a {@link java.lang.String} object.
*/
public String revert()
{
gpUtil.revertImplementation(getPage());
return loadHeader();
}
/**
* greenPepperize.
*
* @return a {@link java.lang.String} object.
*/
public String greenPepperize()
{
if(pepperize)
{
try
{
Specification spec = Specification.newInstance(getPage().getTitle());
spec.setRepository(gpUtil.getHomeRepository(spaceKey));
specification = gpUtil.getGPServerService().createSpecification(spec);
return loadHeader();
}
catch (GreenPepperServerException e)
{
addActionError(e.getId());
}
}
else
{
try
{
Specification spec = Specification.newInstance(getPage().getTitle());
spec.setRepository(gpUtil.getHomeRepository(spaceKey));
// Clean spec
gpUtil.getGPServerService().removeSpecification(spec);
gpUtil.saveExecuteChildren(page, false);
gpUtil.saveImplementedVersion(getPage(), null);
gpUtil.savePreviousImplementedVersion(getPage(), null);
specification = null;
}
catch (GreenPepperServerException e)
{
addActionError(e.getId());
return loadHeader();
}
}
return SUCCESS;
}
/**
* updateExecuteChildren.
*
* @return a {@link java.lang.String} object.
*/
public String updateExecuteChildren()
{
gpUtil.saveExecuteChildren(page, doExecuteChildren);
return SUCCESS;
}
/**
* getForcedSystemUnderTests.
*
* @return a {@link java.util.List} object.
*/
public List getForcedSystemUnderTests()
{
return null;
}
/**
* getCanBeImplemented.
*
* @return a boolean.
*/
public boolean getCanBeImplemented()
{
return gpUtil.canBeImplemented(getPage());
}
/**
* getCanBeReverted.
*
* @return a boolean.
*/
public boolean getCanBeReverted()
{
return getPreviousImplementedVersion() != null;
}
/**
* getImplementedVersion.
*
* @return a {@link java.lang.Integer} object.
*/
public Integer getImplementedVersion()
{
return gpUtil.getImplementedVersion(getPage());
}
/**
* getPreviousImplementedVersion.
*
* @return a {@link java.lang.Integer} object.
*/
public Integer getPreviousImplementedVersion()
{
return gpUtil.getPreviousImplementedVersion(getPage());
}
/**
* getRenderedContent.
*
* @return a {@link java.lang.String} object.
*/
public String getRenderedContent()
{
String content;
try
{
content = gpUtil.getPageContent(getPage(), implemented);
}
catch(GreenPepperServerException e)
{
content = "";
}
return gpUtil.getViewRenderer().render(content, new DefaultConversionContext(getPage().toPageContext()));
}
/**
* getExecutableList.
*
* @return a {@link java.util.LinkedList} object.
*/
@SuppressWarnings("unchecked")
public LinkedList getExecutableList()
{
if(!getDoExecuteChildren())
return new LinkedList();
return super.getExecutableList();
}
/**
* Getter for the field hasChildren
.
*
* @return a boolean.
*/
public boolean getHasChildren()
{
if(hasChildren != null)
return hasChildren;
hasChildren = !implemented && !super.getExecutableList().isEmpty();
return hasChildren;
}
/**
* Getter for the field doExecuteChildren
.
*
* @return a boolean.
*/
public boolean getDoExecuteChildren()
{
if(doExecuteChildren != null)
return doExecuteChildren;
doExecuteChildren = getHasChildren() && gpUtil.getExecuteChildren(page);
return doExecuteChildren;
}
/**
* Setter for the field doExecuteChildren
.
*
* @param doExecuteChildren a boolean.
*/
public void setDoExecuteChildren(boolean doExecuteChildren)
{
this.doExecuteChildren = doExecuteChildren;
}
/**
* getExecutionUID.
*
* @return a {@link java.lang.String} object.
*/
public String getExecutionUID()
{
return "HEADER";
}
/**
* getIsSutEditable.
*
* @return a boolean.
*/
public boolean getIsSutEditable()
{
return true;
}
/**
* getAllChildren.
*
* @return a boolean.
*/
public boolean getAllChildren()
{
return true;
}
/**
* getIsSelfIncluded.
*
* @return a boolean.
*/
public boolean getIsSelfIncluded()
{
return true;
}
/**
* isPepperize.
*
* @return a boolean.
*/
public boolean isPepperize()
{
return pepperize;
}
/**
* Setter for the field pepperize
.
*
* @param pepperize a boolean.
*/
public void setPepperize(boolean pepperize)
{
this.pepperize = pepperize;
}
/**
* Getter for the field retrieveBody
.
*
* @return a boolean.
*/
public boolean getRetrieveBody()
{
return retrieveBody;
}
/**
* Setter for the field retrieveBody
.
*
* @param retrieveBody a boolean.
*/
public void setRetrieveBody(boolean retrieveBody)
{
this.retrieveBody = retrieveBody;
}
/**
* isImplementationDue.
*
* @return a boolean.
*/
public boolean isImplementationDue()
{
return gpUtil.isImplementationDue(getPage());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy