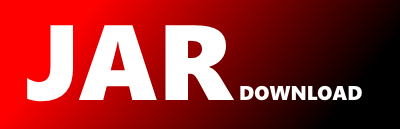
com.greenpepper.confluence.macros.GreenPepperInclude Maven / Gradle / Ivy
package com.greenpepper.confluence.macros;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import com.atlassian.confluence.pages.Page;
import com.atlassian.confluence.renderer.PageContext;
import com.atlassian.confluence.renderer.radeox.macros.MacroUtils;
import com.atlassian.confluence.util.velocity.VelocityUtils;
import com.atlassian.renderer.RenderContext;
import com.atlassian.renderer.v2.macro.MacroException;
import com.greenpepper.confluence.utils.MacroCounter;
import com.greenpepper.server.GreenPepperServerException;
/**
* GreenPepperInclude class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class GreenPepperInclude extends AbstractGreenPepperMacro
{
private static final String INCLUDED_PAGE_PARAM_NAME = "gp$included";
/** {@inheritDoc} */
@Override
public boolean isInline()
{
return true;
}
/** {@inheritDoc} */
public String execute(@SuppressWarnings("rawtypes") Map parameters, String body, RenderContext renderContext) throws MacroException
{
final PageContext context = (PageContext) renderContext;
final boolean isRoot = ( getIncludedPagesParam( context ) == null );
try
{
checkMandatoryPageTitleParameter( parameters );
final String spaceKey = getSpaceKey( parameters, renderContext, false );
final String pageTitle = getPageTitle( parameters, renderContext, spaceKey );
final Page owner = (Page)context.getEntity();
Page page = gpUtil.getPageManager().getPage( spaceKey, pageTitle );
List includedPages = getSafeIncludedPagesParam( context, owner );
if (includedPages.contains( page ))
{
throw new GreenPepperServerException( "greenpepper.include.recursivitydetection", "" );
}
try
{
includedPages.add( page );
return render( parameters, context, pageTitle, page );
}
finally
{
includedPages.remove( page );
}
}
catch (GreenPepperServerException gpe)
{
return getErrorView( "greenpepper.include.macroid", gpe.getId() , gpe.getLocalizedMessage() );
}
catch (Exception e)
{
return getErrorView( "greenpepper.include.macroid", e.getMessage() );
}
finally {
if (isRoot) {
cleanIncludedPagesParam(context);
}
}
}
private void checkMandatoryPageTitleParameter(@SuppressWarnings("rawtypes") Map parameters) throws GreenPepperServerException
{
if (!parameters.containsKey( "pageTitle" ))
{
throw new GreenPepperServerException( "greenpepper.children.pagenotfound", "" );
}
}
@SuppressWarnings("unchecked")
private List getIncludedPagesParam(PageContext context)
{
return (List) context.getParam( INCLUDED_PAGE_PARAM_NAME );
}
private List getSafeIncludedPagesParam(PageContext context, Page owner)
{
List pages = getIncludedPagesParam(context);
if (pages == null)
{
pages = new ArrayList();
pages.add( owner );
context.addParam( INCLUDED_PAGE_PARAM_NAME, pages );
}
return pages;
}
@SuppressWarnings("unchecked")
private String render(@SuppressWarnings("rawtypes") Map parameters, PageContext context, String pageTitle, Page page)
{
Map contextMap = MacroUtils.defaultVelocityContext();
String title = (String) parameters.get( "title" );
contextMap.put( "title", title != null ? title : pageTitle );
contextMap.put( "includeHtml", gpUtil.getViewRenderer().render(page));
contextMap.put( "executionUID", "GP_INCLUDE_" + MacroCounter.instance().getNextCount() );
contextMap.put( "expanded", isExpanded( parameters ) );
return VelocityUtils.getRenderedTemplate( "/templates/greenpepper/confluence/macros/greenPepperInclude.vm", contextMap );
}
private void cleanIncludedPagesParam(PageContext context) {
List pages = getIncludedPagesParam(context);
if (pages != null) {
pages.clear();
}
context.addParam( INCLUDED_PAGE_PARAM_NAME, null );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy