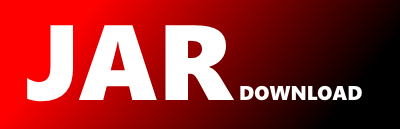
com.greenpepper.runner.repository.AtlassianRepository Maven / Gradle / Ivy
package com.greenpepper.runner.repository;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
import java.util.Vector;
import org.apache.commons.lang3.StringUtils;
import org.apache.xmlrpc.XmlRpcClient;
import org.apache.xmlrpc.XmlRpcRequest;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.greenpepper.document.Document;
import com.greenpepper.html.HtmlDocumentBuilder;
import com.greenpepper.repository.DocumentNotFoundException;
import com.greenpepper.repository.DocumentRepository;
import com.greenpepper.repository.RepositoryException;
import com.greenpepper.util.CollectionUtil;
import com.greenpepper.util.IOUtil;
import com.greenpepper.util.URIUtil;
/**
* AtlassianRepository class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class AtlassianRepository implements DocumentRepository
{
/** Constant PAGE_NOT_FOUND="Page Not Found !"
*/
public static final String PAGE_NOT_FOUND = "Page Not Found !";
/** Constant INSUFFICIENT_PRIVILEGES="INSUFFICIENT PRIVILEGES !"
*/
public static final String INSUFFICIENT_PRIVILEGES = "INSUFFICIENT PRIVILEGES !";
/** Constant SESSION_INVALID="Session Invalid !"
*/
public static final String SESSION_INVALID = "Session Invalid !";
/** Constant PARAMETERS_MISSING="Parameters Missing, expecting:[SpaceKey"{trunked}
*/
public static final String PARAMETERS_MISSING = "Parameters Missing, expecting:[SpaceKey, PageTitle, IncludeStyle] !";
private static final Logger logger = LoggerFactory.getLogger(AtlassianRepository.class);
private final URI root;
private String handler;
private boolean includeStyle;
private String username = "";
private String password = "";
/**
* Constructor for AtlassianRepository.
*
* @param args a {@link java.lang.String} object.
* @throws java.lang.Exception if any.
*/
public AtlassianRepository(String... args) throws Exception
{
this.root = URI.create(URIUtil.raw(args[0]));
String includeAtt = URIUtil.getAttribute(root, "includeStyle");
includeStyle = includeAtt == null ? true : Boolean.valueOf(includeAtt);
handler = URIUtil.getAttribute(root, "handler");
if(handler == null) throw new IllegalArgumentException("Missing handler");
if(args.length == 3)
{
username = args[1];
password = args[2];
}
}
/** {@inheritDoc} */
public Document loadDocument(String location) throws Exception
{
String spec = retrieveSpecification(URI.create(URIUtil.raw(location)));
logger.trace("Page retrieved from the repository for location '{}'\n{}", location,spec);
// check if there is an error in the page
org.jsoup.nodes.Document jsoupDoc = Jsoup.parse(spec);
Elements select = jsoupDoc.select("#conf_actionError_Msg");
for (Element element : select) {
if (element.hasText()) {
if ( StringUtils.equals(element.text(), PAGE_NOT_FOUND)) {
throw new DocumentNotFoundException(location);
} else if (StringUtils.equals(element.text(), PARAMETERS_MISSING)){
throw new RepositoryException(PARAMETERS_MISSING);
} else if (StringUtils.equals(element.text(), SESSION_INVALID)){
throw new RepositoryException(SESSION_INVALID);
} else if (StringUtils.equals(element.text(), INSUFFICIENT_PRIVILEGES)){
throw new RepositoryException(INSUFFICIENT_PRIVILEGES);
}
}
}
return loadHtmlDocument( spec );
}
/** {@inheritDoc} */
public void setDocumentAsImplemeted(String location) throws Exception
{
Vector> args = CollectionUtil.toVector( username , password, args(URI.create(URIUtil.raw(location))));
XmlRpcClient xmlrpc = new XmlRpcClient( root.getScheme() + "://" + root.getAuthority() + root.getPath() );
String msg = (String)xmlrpc.execute( new XmlRpcRequest( handler + ".setSpecificationAsImplemented", args ) );
if(!("".equals(msg))) throw new Exception(msg);
}
/** {@inheritDoc} */
public List listDocuments(String uri)
{
return new ArrayList();
}
/**
* listDocumentsInHierarchy.
*
* @return a {@link java.util.List} object.
* @throws java.lang.Exception if any.
*/
@SuppressWarnings("unchecked")
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy