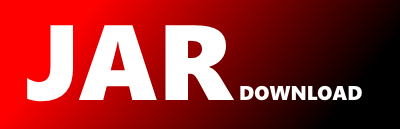
org.w3c.dom.DOMException Maven / Gradle / Ivy
// Copyright (c) 1998 by W3C
//
// DOM is a trademark of W3C
// The DOM level 1 specification, from which this
// source is derived, is copyright by W3C.
// See: http://www.w3.org/TR/REC-DOM-Level-1/
//
package org.w3c.dom;
/**
DOM operations only raise exceptions in "exceptional"
circumstances, i.e., when an operation is impossible
to perform (either for logical reasons, because data is lost, or
because the implementation has become unstable). In general, DOM methods
return specific error values in ordinary
processing situation, such as out-of-bound errors when using
NodeList
.
Implementations may raise other exceptions under other circumstances.
For example, implementations may raise an implementation-dependent
exception if a null
argument is passed.
Some languages and object systems do not support the concept of
exceptions. For such systems, error conditions may be indicated using
native error reporting mechanisms. For some bindings, for example, methods
may return error codes similar to those listed in the corresponding method
descriptions.
*/
public abstract class DOMException
extends RuntimeException
{
/** Constructs an exception with the specified
* descriptive detail message.
* @param code Integer indicating type of error
* @param message Descriptive message */
public DOMException (short code, String message)
{ super (message); this.code = code; }
/**
* Indicates the type of error that
* the DOM implementation is reporting. This
* value is one of the *_ERR codes.
* @serial DOM Exception code
*/
public short code;
/**
If index or size is negative, or greater than the
allowed value
*/
public static final short INDEX_SIZE_ERR = 1;
/**
If the specified range of text does not fit into a String
*/
public static final short DOMSTRING_SIZE_ERR = 2;
/**
If any node is inserted somewhere it doesn't belong
*/
public static final short HIERARCHY_REQUEST_ERR = 3;
/**
If a node is used in a different document than the one that
created it (that doesn't support it)
*/
public static final short WRONG_DOCUMENT_ERR = 4;
/**
If an invalid character is specified, such as in a name.
*/
public static final short INVALID_CHARACTER_ERR = 5;
/**
If data is specified for a node which does not support data
*/
public static final short NO_DATA_ALLOWED_ERR = 6;
/**
If an attempt is made to modify an object where modifications are not allowed
*/
public static final short NO_MODIFICATION_ALLOWED_ERR = 7;
/**
If an attempt was made to reference a node in a context where it does not exist
*/
public static final short NOT_FOUND_ERR = 8;
/**
If the implementation does not support the type of object requested
*/
public static final short NOT_SUPPORTED_ERR = 9;
/**
If an attempt is made to add an attribute that is already inuse elsewhere
*/
public static final short INUSE_ATTRIBUTE_ERR = 10;
}