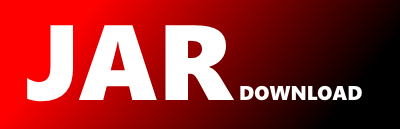
org.w3c.dom.html.HTMLAnchorElement Maven / Gradle / Ivy
// Copyright (c) 1998 by W3C
//
// DOM is a trademark of W3C
// The DOM level 1 specification, from which this
// source is derived, is copyright by W3C.
// See: http://www.w3.org/TR/REC-DOM-Level-1/
//
package org.w3c.dom.html;
import org.w3c.dom.*;
/**
The anchor element. See the
A element definition
in HTML 4.0.
Property Summary
accessKey
getAccessKey
setAccessKey
A single character access key to give access to the form control. See the
accesskey attribute definition
in HTML 4.0.
charset
getCharset
setCharset
The character encoding of the linked resource. See the
charset attribute definition
in HTML 4.0.
coords
getCoords
setCoords
Comma-separated list of lengths, defining an active region geometry. See also
shape
for the shape of the region. See the
coords attribute definition
in HTML 4.0.
href
getHref
setHref
The URI of the linked resource. See the
href attribute definition
in HTML 4.0.
hreflang
getHreflang
setHreflang
Language code of the linked resource. See the
hreflang attribute definition
in HTML 4.0.
name
getName
setName
Anchor name. See the
name attribute definition
in HTML 4.0.
rel
getRel
setRel
Forward link type. See the
rel attribute definition
in HTML 4.0.
rev
getRev
setRev
Reverse link type. See the
rev attribute definition
in HTML 4.0.
shape
getShape
setShape
The shape of the active area. The coordinates are given by
coords
. See the
shape attribute definition
in HTML 4.0.
tabIndex
getTabIndex
setTabIndex
Index that represents the element's position in the tabbing order. See the
tabindex attribute definition
in HTML 4.0.
target
getTarget
setTarget
Frame to render the resource in. See the
target attribute definition
in HTML 4.0.
type
getType
setType
Advisory content type. See the
type attribute definition
in HTML 4.0.
*/
public interface HTMLAnchorElement
extends HTMLElement
{
/** Assigns the value of the accessKey
property.
*/
void setAccessKey (String accessKey);
/**
* Returns the value of the accessKey
property.
*/
String getAccessKey ();
/** Assigns the value of the charset
property.
*/
void setCharset (String charset);
/**
* Returns the value of the charset
property.
*/
String getCharset ();
/** Assigns the value of the coords
property.
*/
void setCoords (String coords);
/**
* Returns the value of the coords
property.
*/
String getCoords ();
/** Assigns the value of the href
property.
*/
void setHref (String href);
/**
* Returns the value of the href
property.
*/
String getHref ();
/** Assigns the value of the hreflang
property.
*/
void setHreflang (String hreflang);
/**
* Returns the value of the hreflang
property.
*/
String getHreflang ();
/** Assigns the value of the name
property.
*/
void setName (String name);
/**
* Returns the value of the name
property.
*/
String getName ();
/** Assigns the value of the rel
property.
*/
void setRel (String rel);
/**
* Returns the value of the rel
property.
*/
String getRel ();
/** Assigns the value of the rev
property.
*/
void setRev (String rev);
/**
* Returns the value of the rev
property.
*/
String getRev ();
/** Assigns the value of the shape
property.
*/
void setShape (String shape);
/**
* Returns the value of the shape
property.
*/
String getShape ();
/** Assigns the value of the tabIndex
property.
*/
void setTabIndex (int tabIndex);
/**
* Returns the value of the tabIndex
property.
*/
int getTabIndex ();
/** Assigns the value of the target
property.
*/
void setTarget (String target);
/**
* Returns the value of the target
property.
*/
String getTarget ();
/** Assigns the value of the type
property.
*/
void setType (String type);
/**
* Returns the value of the type
property.
*/
String getType ();
/**
Removes keyboard focus from this element.
*/
void blur ();
/**
Gives keyboard focus to this element.
*/
void focus ();
}