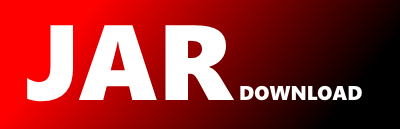
org.w3c.dom.html.HTMLButtonElement Maven / Gradle / Ivy
// Copyright (c) 1998 by W3C
//
// DOM is a trademark of W3C
// The DOM level 1 specification, from which this
// source is derived, is copyright by W3C.
// See: http://www.w3.org/TR/REC-DOM-Level-1/
//
package org.w3c.dom.html;
import org.w3c.dom.*;
/**
Push button. See the
BUTTON element definition
in HTML 4.0.
Property Summary
form
getForm
Returns the
FORM
element containing this control. Returns null if this control is not within the context of a form.
accessKey
getAccessKey
setAccessKey
A single character access key to give access to the form control. See the
accesskey attribute definition
in HTML 4.0.
disabled
getDisabled
setDisabled
The control is unavailable in this context. See the
disabled attribute definition
in HTML 4.0.
name
getName
setName
Form control or object name when submitted with a form. See the
name attribute definition
in HTML 4.0.
tabIndex
getTabIndex
setTabIndex
Index that represents the element's position in the tabbing order. See the
tabindex attribute definition
in HTML 4.0.
type
getType
The type of button. See the
type attribute definition
in HTML 4.0.
value
getValue
setValue
The current form control value. See the
value attribute definition
in HTML 4.0.
*/
public interface HTMLButtonElement
extends HTMLElement
{
/**
* Returns the value of the form
property.
*/
HTMLFormElement getForm ();
/** Assigns the value of the accessKey
property.
*/
void setAccessKey (String accessKey);
/**
* Returns the value of the accessKey
property.
*/
String getAccessKey ();
/** Assigns the value of the disabled
property.
*/
void setDisabled (boolean disabled);
/**
* Returns the value of the disabled
property.
*/
boolean getDisabled ();
/** Assigns the value of the name
property.
*/
void setName (String name);
/**
* Returns the value of the name
property.
*/
String getName ();
/** Assigns the value of the tabIndex
property.
*/
void setTabIndex (int tabIndex);
/**
* Returns the value of the tabIndex
property.
*/
int getTabIndex ();
/**
* Returns the value of the type
property.
*/
String getType ();
/** Assigns the value of the value
property.
*/
void setValue (String value);
/**
* Returns the value of the value
property.
*/
String getValue ();
}