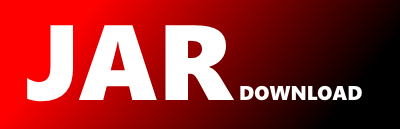
org.w3c.dom.html.HTMLDocument Maven / Gradle / Ivy
// Copyright (c) 1998 by W3C
//
// DOM is a trademark of W3C
// The DOM level 1 specification, from which this
// source is derived, is copyright by W3C.
// See: http://www.w3.org/TR/REC-DOM-Level-1/
//
package org.w3c.dom.html;
import org.w3c.dom.*;
/**
An
HTMLDocument
is the root of the HTML hierarchy and holds the entire content. Beside providing access to the hierarchy, it also provides some convenience methods for accessing certain sets of information from the document.
The following properties have been deprecated in favor of the corresponding ones for the BODY element:
-
alinkColor
-
background
-
bgColor
-
fgColor
-
linkColor
-
vlinkColor
Property Summary
title
getTitle
setTitle
The title of a document as specified by the
TITLE
element in the head of the document.
referrer
getReferrer
Returns the URI of the page that linked to this page. The value is an empty string if the user navigated to the page directly (not through a link, but, for example, via a bookmark).
domain
getDomain
The domain name of the server that served the document, or a null string if the server cannot be identified by a domain name.
URL
getURL
The complete URI of the document.
body
getBody
setBody
The element that contains the content for the document. In documents with
BODY
contents, returns the
BODY
element, and in frameset documents, this returns the outermost
FRAMESET
element.
images
getImages
A collection of all the
IMG
elements in a document. The behavior is limited to
IMG
elements for backwards compatibility.
applets
getApplets
A collection of all the
OBJECT
elements that include applets and
APPLET
(
deprecated ) elements in a document.
links
getLinks
A collection of all
AREA
elements and anchor (
A
) elements in a document with a value for the
href
attribute.
forms
getForms
A collection of all the forms of a document.
anchors
getAnchors
A collection of all the anchor (
A
) elements in a document with a value for the
name
attribute.
Note. For reasons of backwards compatibility, the returned set of anchors only contains those anchors created with the
name
attribute, not those created with the
id
attribute.
cookie
getCookie
setCookie
The cookies associated with this document. If there are none, the value is an empty string. Otherwise, the value is a string: a semicolon-delimited list of "name, value" pairs for all the cookies associated with the page. For example,
name=value;expires=date
.
*/
public interface HTMLDocument
extends Document
{
/** Assigns the value of the title
property.
*/
void setTitle (String title);
/**
* Returns the value of the title
property.
*/
String getTitle ();
/**
* Returns the value of the referrer
property.
*/
String getReferrer ();
/**
* Returns the value of the domain
property.
*/
String getDomain ();
/**
* Returns the value of the URL
property.
*/
String getURL ();
/** Assigns the value of the body
property.
*/
void setBody (HTMLElement body);
/**
* Returns the value of the body
property.
*/
HTMLElement getBody ();
/**
* Returns the value of the images
property.
*/
HTMLCollection getImages ();
/**
* Returns the value of the applets
property.
*/
HTMLCollection getApplets ();
/**
* Returns the value of the links
property.
*/
HTMLCollection getLinks ();
/**
* Returns the value of the forms
property.
*/
HTMLCollection getForms ();
/**
* Returns the value of the anchors
property.
*/
HTMLCollection getAnchors ();
/** Assigns the value of the cookie
property.
*/
void setCookie (String cookie);
/**
* Returns the value of the cookie
property.
*/
String getCookie ();
/**
Note.
This method and the ones following allow a user to add to or replace the structure model of a document using strings of unparsed HTML. At the time of writing alternate methods for providing similar functionality for both HTML and XML documents were being considered. The following methods may be deprecated at some point in the future in favor of a more general-purpose mechanism.
Open a document stream for writing. If a document exists in the target, this method clears it.
*/
void open ();
/**
Closes a document stream opened by
open()
and forces rendering.
*/
void close ();
/**
Write a string of text to a document stream opened by
open()
. The text is parsed into the document's structure model.
@param text
The string to be parsed into some structure in the document structure model.
*/
void write (String text);
/**
Write a string of text followed by a newline character to a document stream opened by
open()
. The text is parsed into the document's structure model.
@param text
The string to be parsed into some structure in the document structure model.
*/
void writeln (String text);
/**
Returns the Element whose
id
is given by elementId. If no such element exists, returns
null
. Behavior is not defined if more than one element has this
id
.
@return The matching element.
@param elementId
The unique
id
value for an element.
*/
Element getElementById (String elementId);
/**
Returns the (possibly empty) collection of elements whose
name
value is given by
elementName
.
@return The matching elements.
@param elementName
The
name
attribute value for an element.
*/
NodeList getElementsByName (String elementName);
}