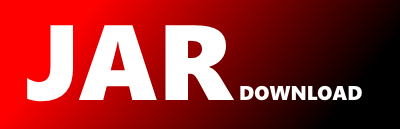
org.w3c.dom.html.HTMLTableElement Maven / Gradle / Ivy
// Copyright (c) 1998 by W3C
//
// DOM is a trademark of W3C
// The DOM level 1 specification, from which this
// source is derived, is copyright by W3C.
// See: http://www.w3.org/TR/REC-DOM-Level-1/
//
package org.w3c.dom.html;
import org.w3c.dom.*;
/**
The create* and delete* methods on the table allow authors to construct and modify tables. HTML 4.0 specifies that only one of each of the
CAPTION
,
THEAD
, and
TFOOT
elements may exist in a table. Therefore, if one exists, and the createTHead() or createTFoot() method is called, the method returns the existing THead or TFoot element. See the
TABLE element definition
in HTML 4.0.
Property Summary
caption
getCaption
setCaption
Returns the table's
CAPTION
, or void if none exists.
tHead
getTHead
setTHead
Returns the table's
THEAD
, or
null
if none exists.
tFoot
getTFoot
setTFoot
Returns the table's
TFOOT
, or
null
if none exists.
rows
getRows
Returns a collection of all the rows in the table, including all in
THEAD
,
TFOOT
, all
TBODY
elements.
tBodies
getTBodies
Returns a collection of the defined table bodies.
align
getAlign
setAlign
Specifies the table's position with respect to the rest of the document. See the
align attribute definition
in HTML 4.0. This attribute is deprecated in HTML 4.0.
bgColor
getBgColor
setBgColor
Cell background color. See the
bgcolor attribute definition
in HTML 4.0. This attribute is deprecated in HTML 4.0.
border
getBorder
setBorder
The width of the border around the table. See the
border attribute definition
in HTML 4.0.
cellPadding
getCellPadding
setCellPadding
Specifies the horizontal and vertical space between cell content and cell borders. See the
cellpadding attribute definition
in HTML 4.0.
cellSpacing
getCellSpacing
setCellSpacing
Specifies the horizontal and vertical separation between cells. See the
cellspacing attribute definition
in HTML 4.0.
frame
getFrame
setFrame
Specifies which external table borders to render. See the
frame attribute definition
in HTML 4.0.
rules
getRules
setRules
Specifies which internal table borders to render. See the
rules attribute definition
in HTML 4.0.
summary
getSummary
setSummary
Supplementary description about the purpose or structure of a table. See the
summary attribute definition
in HTML 4.0.
width
getWidth
setWidth
Specifies the desired table width. See the
width attribute definition
in HTML 4.0.
*/
public interface HTMLTableElement
extends HTMLElement
{
/** Assigns the value of the caption
property.
*/
void setCaption (HTMLTableCaptionElement caption);
/**
* Returns the value of the caption
property.
*/
HTMLTableCaptionElement getCaption ();
/** Assigns the value of the tHead
property.
*/
void setTHead (HTMLTableSectionElement tHead);
/**
* Returns the value of the tHead
property.
*/
HTMLTableSectionElement getTHead ();
/** Assigns the value of the tFoot
property.
*/
void setTFoot (HTMLTableSectionElement tFoot);
/**
* Returns the value of the tFoot
property.
*/
HTMLTableSectionElement getTFoot ();
/**
* Returns the value of the rows
property.
*/
HTMLCollection getRows ();
/**
* Returns the value of the tBodies
property.
*/
HTMLCollection getTBodies ();
/** Assigns the value of the align
property.
*/
void setAlign (String align);
/**
* Returns the value of the align
property.
*/
String getAlign ();
/** Assigns the value of the bgColor
property.
*/
void setBgColor (String bgColor);
/**
* Returns the value of the bgColor
property.
*/
String getBgColor ();
/** Assigns the value of the border
property.
*/
void setBorder (String border);
/**
* Returns the value of the border
property.
*/
String getBorder ();
/** Assigns the value of the cellPadding
property.
*/
void setCellPadding (String cellPadding);
/**
* Returns the value of the cellPadding
property.
*/
String getCellPadding ();
/** Assigns the value of the cellSpacing
property.
*/
void setCellSpacing (String cellSpacing);
/**
* Returns the value of the cellSpacing
property.
*/
String getCellSpacing ();
/** Assigns the value of the frame
property.
*/
void setFrame (String frame);
/**
* Returns the value of the frame
property.
*/
String getFrame ();
/** Assigns the value of the rules
property.
*/
void setRules (String rules);
/**
* Returns the value of the rules
property.
*/
String getRules ();
/** Assigns the value of the summary
property.
*/
void setSummary (String summary);
/**
* Returns the value of the summary
property.
*/
String getSummary ();
/** Assigns the value of the width
property.
*/
void setWidth (String width);
/**
* Returns the value of the width
property.
*/
String getWidth ();
/**
Create a table header row or return an existing one.
@return A new table header element (
THEAD
).
*/
HTMLElement createTHead ();
/**
Delete the header from the table, if one exists.
*/
void deleteTHead ();
/**
Create a table footer row or return an existing one.
@return A footer element (
TFOOT
).
*/
HTMLElement createTFoot ();
/**
Delete the footer from the table, if one exists.
*/
void deleteTFoot ();
/**
Create a new table caption object or return an existing one.
@return A
CAPTION
element.
*/
HTMLElement createCaption ();
/**
Delete the table caption, if one exists.
*/
void deleteCaption ();
/**
Insert a new empty row in the table.
Note. A table row cannot be empty according to HTML 4.0 Recommendation.
@return The newly created row.
@param index
The row number where to insert a new row.
*/
HTMLElement insertRow (int index);
/**
Delete a table row.
@param index
The index of the row to be deleted.
*/
void deleteRow (int index);
}