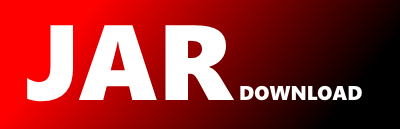
com.greenpepper.maven.plugin.SpecificationNavigatorMojo Maven / Gradle / Ivy
package com.greenpepper.maven.plugin;
import com.greenpepper.maven.plugin.utils.RepositoryIndex;
import com.greenpepper.repository.DocumentNode;
import org.apache.commons.codec.digest.DigestUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import java.io.File;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import static java.lang.String.format;
import static org.apache.commons.lang3.StringUtils.*;
/**
* List the Specifications from the configured repositories.
*
* @goal tree
*
*/
@SuppressWarnings("WeakerAccess")
public class SpecificationNavigatorMojo extends AbstractMojo {
private static final Pattern filterPattern = Pattern.compile("(\\[!?I\\])?(\\[RE\\])?(.*)?");
/**
* This list of repositories.
* @parameter
*/
List repositories;
/**
* Set this to a Repository name defined in the pom.xml.
*
* @parameter property="gp.repo"
*/
String selectedRepository;
/**
* @parameter default-value="${project.build.directory}/greenpepper-reports"
* @required
*/
File reportsDirectory;
/**
* Sets a filter to filter the output of the specs. The filter should have a specific syntax:
*
* "substring"
: a string to look for inside the page name. The search is case insensitive
* "[RE]regular expression"
: a regular expression that will be used to match the page name
*
* Additionnally you can filter on the implemented
status of the page by adding a "[I]"
* as a prefix to your search filter.
*
* [I]
: Give the implemented pages only
* [!I]
: Give the non implemented pages only
*
* Note: A "[I]"
or "[!I]"
as a search filter will filter only on the implemented status.
* Examples:
*
* sun
: all specifications having the substring 'sun'
* [RE]taurus
: the specification matching exactly 'taurus'
* [I]
: all implemented specifications
* [!I]
: all non implemented specifications
* [!I]dummy
: all non implemented specifications having the substring 'dummy'
* [I][RE]'.*moon[^dab]+'
: all implemented specifications having the RE '.*moon[^dab]+'
*
*
* @parameter property="gp.specFilter"
*/
String specFilter;
/**
* Refresh the specificaction list (updating the index file)
*
* @parameter property="greenpepper.refresh" default-value="false"
*/
boolean refresh;
private PrintWriter writer;
HashMap repositoryIndexes = new HashMap();
@SuppressWarnings("WeakerAccess")
public SpecificationNavigatorMojo() {
this.repositories = new ArrayList();
this.writer = new PrintWriter(System.out);
}
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
printBanner();
processAllRepositories();
}
private void processAllRepositories() throws MojoExecutionException, MojoFailureException {
boolean atLeastOneRepositoryProcessed = false;
try {
for (Repository repository : repositories) {
if (isNotEmpty(selectedRepository)) {
if (StringUtils.equals(selectedRepository, repository.getName())) {
listRepositorySpecifications(repository);
atLeastOneRepositoryProcessed = true;
break;
} else {
getLog().debug(format("Skipping repository '%s', selected is '%s' ", repository.getName(), selectedRepository));
}
} else {
listRepositorySpecifications(repository);
}
}
} catch (MojoExecutionException e) {
throw e;
} catch (MojoFailureException e) {
throw e;
} catch (Exception e) {
throw new MojoExecutionException("Error running the Goal", e);
}
if (isNotEmpty(selectedRepository) && !atLeastOneRepositoryProcessed) {
throw new MojoExecutionException("No repository could match your requirements");
}
}
List listRepositorySpecifications(Repository repository) throws Exception {
printRepositoryName(repository);
List filteringSpecs = new ArrayList();
filteringSpecs.addAll(repository.getTests());
for (String suite : repository.getSuites()) {
List tests = repository.getDocumentRepository().listDocuments(suite);
filteringSpecs.addAll(tests);
}
File indexFile = getIndexFileForRepository(repository);
RepositoryIndex repositoryIndex = new RepositoryIndex(indexFile);
if (!indexFile.exists() || refresh) {
DocumentNode documentHierarchy = repository.retrieveDocumentHierarchy();
for (DocumentNode node : DocumentNode.traverser.preOrderTraversal(documentHierarchy)) {
RepositoryIndex.SpecificationInfo specInfo = new RepositoryIndex.SpecificationInfo();
if (node.isExecutable()) {
specInfo.setImplemented(!node.canBeImplemented());
specInfo.setLink(node.getURL());
repositoryIndex.getNameToInfo().put(node.getTitle(), specInfo);
}
}
for (String test : filteringSpecs) {
RepositoryIndex.SpecificationInfo specInfo = new RepositoryIndex.SpecificationInfo();
specInfo.setImplemented(true);
repositoryIndex.getNameToInfo().put(test, specInfo);
}
repositoryIndex.dump();
} else {
System.out.println(format("\tUsing index file '%s'.\n" +
"\tYou can force a refresh by removing it or by using '-Dgreenpepper.refresh=true'.", indexFile.getName()));
System.out.println();
repositoryIndex.load();
}
repositoryIndexes.put(repository.getName(), repositoryIndex);
if (isNotEmpty(specFilter)) {
System.out.println(format("\tFiltering the specifications using '%s'", specFilter));
System.out.println();
}
ArrayList specifications = new ArrayList();
int i = 1;
for (Map.Entry entry : repositoryIndex.getNameToInfo().entrySet()) {
String specification = decideForEntry(i, entry, filteringSpecs, writer);
if (isNotBlank(specification)) {
specifications.add(specification);
}
i++;
}
writer.flush();
System.out.println();
return specifications;
}
private String decideForEntry(int indice, Map.Entry entry, List filteringSpecs, PrintWriter writer) {
boolean lineMatchingReq = false;
if (!filteringSpecs.isEmpty()) {
// We run only those tests
if (filteringSpecs.contains(entry.getKey())) {
// apply the filter
lineMatchingReq = isLineMatchingReq(indice, entry, writer);
}
} else {
lineMatchingReq = isLineMatchingReq(indice, entry, writer);
}
if (lineMatchingReq) {
return entry.getKey();
} else {
return null;
}
}
private boolean isLineMatchingReq(int indice, Map.Entry entry, PrintWriter writer) {
boolean lineMatchingReq = false;
if (isNotEmpty(specFilter)) {
Matcher matcher = filterPattern.matcher(specFilter);
if (matcher.matches()) {
String isImplemented = matcher.group(1);
String isRegEx = matcher.group(2);
String searchStr = matcher.group(3);
boolean matchesImplemented = StringUtils.equals("[I]", isImplemented) && entry.getValue().isImplemented();
boolean matchesNonImplemented = StringUtils.equals("[!I]", isImplemented) && !entry.getValue().isImplemented();
boolean matchesImplementedReq = isEmpty(isImplemented) || (matchesImplemented || matchesNonImplemented);
boolean matchesSearchStringReq = isNotEmpty(isRegEx) || isEmpty(searchStr)
|| (isNotEmpty(searchStr) && containsIgnoreCase(entry.getKey(), searchStr));
boolean matchesRegexStringReq = isEmpty(isRegEx)
|| (isNotEmpty(isRegEx) && Pattern.matches(searchStr, entry.getKey()));
if (matchesImplementedReq && matchesRegexStringReq && matchesSearchStringReq) {
writer.println(outputEntry(indice, entry));
lineMatchingReq = true;
}
}
} else {
writer.println(outputEntry(indice, entry));
lineMatchingReq = true;
}
return lineMatchingReq;
}
private String outputEntry(int indice, Map.Entry entry) {
return format(" [%04d] - [%11s] - [%s]", indice, entry.getValue().isImplemented() ? "implemented" : "", entry.getKey());
}
File getIndexFileForRepository(Repository repository) throws UnsupportedEncodingException {
String indexFilenameWOextension = getRepositoryMetaName(repository);
String indexFilename = indexFilenameWOextension + ".index";
return new File(reportsDirectory, indexFilename);
}
String getRepositoryMetaName(Repository repository) throws UnsupportedEncodingException {
String indentifiers = repository.getProjectName() + repository.getSystemUnderTest() + repository.getType() + repository.getRoot();
return format("%s-%s", repository.getName() , DigestUtils.md5Hex(indentifiers.getBytes("UTF-8")));
}
private void printRepositoryName(Repository repository) {
System.out.println();
System.out.println(format(" Repository : %s (project='%s',sut='%s')",
repository.getName(), repository.getProjectName(), repository.getSystemUnderTest()));
System.out.println(StringUtils.repeat(" =",40));
System.out.println();
}
/**
* addRepository.
*
* @param repository a {@link com.greenpepper.maven.plugin.Repository} object.
*/
void addRepository(Repository repository) {
repositories.add(repository);
}
private void printBanner() {
System.out.println();
System.out.println("----------------------------------------------------------------");
System.out.println(" G R E E N P E P P E R S P E C I F I C A T I O N S L I S T ");
System.out.println("----------------------------------------------------------------");
System.out.println();
}
void setPrintWriter(PrintWriter writer) {
this.writer = writer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy