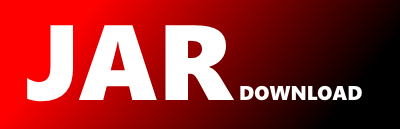
com.greenpepper.maven.plugin.runner.Runner Maven / Gradle / Ivy
package com.greenpepper.maven.plugin.runner;
import static com.greenpepper.maven.plugin.SpecificationRunnerMojo.recoverLinkInResult;
import static com.greenpepper.util.URIUtil.escapeFileSystemForbiddenCharacters;
import static java.lang.String.format;
import static org.apache.commons.io.FileUtils.writeStringToFile;
import static org.apache.commons.lang3.StringUtils.contains;
import static org.apache.commons.lang3.StringUtils.defaultString;
import static org.apache.commons.lang3.StringUtils.endsWithIgnoreCase;
import static org.apache.commons.lang3.StringUtils.isNotBlank;
import static org.apache.commons.lang3.StringUtils.join;
import java.io.File;
import java.io.IOException;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.greenpepper.GreenPepperCore;
import com.greenpepper.maven.plugin.utils.RepositoryIndex;
import com.greenpepper.repository.DocumentRepository;
import com.greenpepper.runner.SpecificationRunnerMonitor;
import com.greenpepper.server.domain.EnvironmentType;
import com.greenpepper.server.domain.Execution;
import com.greenpepper.server.domain.Specification;
import com.greenpepper.server.domain.SystemUnderTest;
public class Runner extends com.greenpepper.server.domain.Runner {
private static final Logger LOGGER = LoggerFactory.getLogger(Runner.class);
public static final String DEFAULT_RUNNER_NAME = "GP Core " + GreenPepperCore.VERSION;
private static final String XML_OPTION = " --xml";
private int forkCount = 1;
private boolean redirectOutputToFile;
private boolean includeProjectClasspath;
private RepositoryIndex repositoryIndex;
public Runner() {
setEnvironmentType(EnvironmentType.newInstance("JAVA"));
}
public static Runner createDefault(String jvm, String javaOptions, List optionsList) {
Runner defaultRunner = new Runner();
defaultRunner.setName(DEFAULT_RUNNER_NAME);
defaultRunner.setMainClass("com.greenpepper.runner.Main");
String cmdLineTemplate = format("%s %s -cp ${classpaths} ${mainClass} ${inputPath} ${outputPath} "
+ "-r ${repository} -f ${fixtureFactory} %s", jvm, defaultString(javaOptions), join(optionsList, " "));
defaultRunner.setCmdLineTemplate(cmdLineTemplate);
defaultRunner.setIncludeProjectClasspath(true);
return defaultRunner;
}
public void execute(Specification specification, SystemUnderTest systemUnderTest, String outputPath, SpecificationRunnerMonitor monitor) throws IOException {
monitor.testRunning(specification.getName());
Execution execution;
String escapedOutputPath = escapeFileSystemForbiddenCharacters(outputPath);
String xmlReportPath = escapedOutputPath + ".xml";
// set the --xml option internally cause we need it for the plugin
if (!contains(getCmdLineTemplate(), XML_OPTION)) {
setCmdLineTemplate(getCmdLineTemplate() + XML_OPTION);
}
// by setting imlemented version to true, we rely on the specification name itself to hold the implemented tag
String stdOutFile = escapedOutputPath + "-output.log";
String stdErrFile = escapedOutputPath + "-err.log";
if(isRemote()) {
execution = executeRemotely(specification, systemUnderTest, true, null, null);
writeStringToFile(new File(stdOutFile), execution.getStdoutLogs());
writeStringToFile(new File(stdErrFile), execution.getStderrLogs());
} else {
execution = executeLocally(specification, systemUnderTest, true, null, null, xmlReportPath, stdOutFile, stdErrFile, redirectOutputToFile);
}
if (isNotBlank(execution.getExecutionErrorId())) {
if (contains(execution.getExecutionErrorId(), DocumentRepository.THIS_SPECIFICATION_WAS_NEVER_SET_AS_IMPLEMENTED)) {
LOGGER.debug(format("%s: %s", DocumentRepository.THIS_SPECIFICATION_WAS_NEVER_SET_AS_IMPLEMENTED, specification.getName()));
monitor.testDone(0, 0, 0, 0);
} else {
LOGGER.error("Failed to execute the specification : " + execution.getExecutionErrorId());
monitor.testDone(0, 0, 1, 0);
}
} else {
monitor.testDone(execution.getSuccess(), execution.getFailures(), execution.getErrors(), execution.getIgnored());
}
String cleanedResults = execution.getCleanedResults();
String htmlOutputPath = endsWithIgnoreCase(escapedOutputPath, ".html") ? escapedOutputPath : escapedOutputPath + ".html";
if (isNotBlank(cleanedResults)) {
writeStringToFile(new File(htmlOutputPath), cleanedResults);
}
if (repositoryIndex != null && repositoryIndex.getNameToInfo().containsKey(specification.getName()) ) {
recoverLinkInResult(specification.getName(), cleanedResults, repositoryIndex);
}
}
public int getForkCount() {
return forkCount;
}
public void setForkCount(int forkCount) {
this.forkCount = forkCount;
}
public void setRedirectOutputToFile(boolean redirectOutputToFile) {
this.redirectOutputToFile = redirectOutputToFile;
}
public boolean isIncludeProjectClasspath() {
return includeProjectClasspath;
}
@SuppressWarnings("WeakerAccess")
public void setIncludeProjectClasspath(boolean includeProjectClasspath) {
this.includeProjectClasspath = includeProjectClasspath;
}
@Override
public void setCmdLineTemplate(String cmdLineTemplate) {
String newCmdLine = cmdLineTemplate.replaceAll("\\$?(\\{[^}]+})", "\\$$1");
super.setCmdLineTemplate(newCmdLine);
}
public void setRepositoryIndex(RepositoryIndex repositoryIndex) {
this.repositoryIndex = repositoryIndex;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy