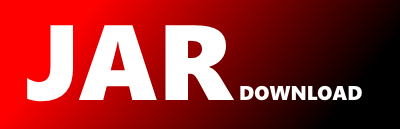
com.greenpepper.maven.plugin.spy.SpyFixture Maven / Gradle / Ivy
package com.greenpepper.maven.plugin.spy;
import com.greenpepper.reflect.CollectionProvider;
import com.greenpepper.reflect.EnterRow;
import com.greenpepper.reflect.Fixture;
import com.greenpepper.reflect.Message;
import com.greenpepper.spy.*;
import org.apache.commons.lang3.StringUtils;
import java.util.*;
import static com.greenpepper.spy.FixtureDescription.FixtureType.WORKFLOW;
public class SpyFixture implements Fixture, FixtureDescription {
private SortedSet constructors = new TreeSet();
private SortedSet properties = new TreeSet();
private SortedSet methods = new TreeSet();
private String name;
private String rawName;
private FixtureType type = WORKFLOW;
public SpyFixture(String fixtureName) {
this.name = fixtureName;
}
@Override
public boolean canCheck(String message) {
return true;
}
@Override
public FixtureType getType() {
return type;
}
public void setType(FixtureType type) {
this.type = type;
}
@Override
public Message check(String message) {
SpyOn spyOn;
switch (type) {
case COLLECTION_PROVIDER:
spyOn = SpyOn.property(this, message);
break;
case SETUP:
default:
spyOn = SpyOn.function(this, message);
}
return spyOn;
}
public Message getter(String message) {
return SpyOn.function(this, message);
}
public boolean respondsTo(String message) {
return true;
}
@Override
public boolean canSend(String message) {
return true;
}
@Override
public Message send(String message) {
return SpyOn.property(this, message);
}
@Override
public Fixture fixtureFor(Object target) {
if (target instanceof SpyCallResult) {
SpyCallResult spyCallResult = (SpyCallResult) target;
SpyFixture spyFixture = null;
for (MethodDescription method : methods) {
if (method.getArity() == 0 && StringUtils.equals(method.getRawName(),spyCallResult.message) ) {
spyFixture = (SpyFixture) method.getSubFixtureDescription();
if (spyFixture != null) {
break;
} else {
spyFixture = new SpyFixture(spyCallResult.message);
spyFixture.rawName = spyCallResult.message;
((Method)method).setSubFixtureSpy(spyFixture);
break;
}
}
}
if (spyFixture == null) {
throw new IllegalArgumentException("The method for the collection provider should have already been seen.");
}
return spyFixture;
}
return this;
}
@Override
public Object getTarget() {
return this;
}
@Override
public String getName() {
return this.name;
}
@Override
public Set getProperties() {
return this.properties;
}
@Override
public Set getMethods() {
return this.methods;
}
@Override
public Set getConstructors() {
return this.constructors;
}
void addConstructors(Constructor constructor) {
this.constructors.add(constructor);
}
void addMethod(Method method) {
this.methods.add(method);
}
void addProperty(Property property) {
this.properties.add(property);
}
public boolean equals(Object other) {
if (other == null) {
return false;
}
if (!(other instanceof SpyFixture)) {
return false;
}
return this.name.equals(((SpyFixture)other).name);
}
public int hashCode() {
return this.name.hashCode();
}
void setRawName(String rawName) {
this.rawName = rawName;
}
@Override
public String getRawName() {
return rawName;
}
@CollectionProvider
public Collection> spyForCollectionProvider() {
type = FixtureType.COLLECTION_PROVIDER;
return Collections.singleton(this);
}
@EnterRow
public void spyForEnterRow() {
type = FixtureType.SETUP;
}
@Override
public PojoDescription getPojo() {
return new Pojo(getRawName() + " item", getProperties());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy