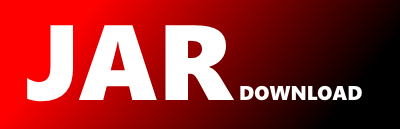
com.greenpepper.repository.FileSystemRepository Maven / Gradle / Ivy
/*
* Copyright (c) 2006 Pyxis Technologies inc.
*
* This is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA,
* or see the FSF site: http://www.fsf.org.
*/
package com.greenpepper.repository;
import com.greenpepper.document.Document;
import com.greenpepper.html.HtmlDocumentBuilder;
import com.greenpepper.util.IOUtil;
import com.greenpepper.util.URIUtil;
import java.io.File;
import java.io.FileFilter;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Hashtable;
import java.util.List;
import java.util.Vector;
import static com.greenpepper.shaded.org.apache.commons.lang3.StringUtils.defaultString;
/**
* FileSystemRepository class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class FileSystemRepository implements DocumentRepository
{
private static final FileFilter NOT_HIDDEN = new NotHiddenFilter();
private final File root;
/**
* Constructor for FileSystemRepository.
*
* @param args a {@link java.lang.String} object.
*/
public FileSystemRepository( String... args )
{
if (args.length != 1) throw new IllegalArgumentException("root");
this.root = new File(URIUtil.decoded(args[0]));
}
/**
* Constructor for FileSystemRepository.
*
* @param root a {@link java.io.File} object.
*/
public FileSystemRepository( File root )
{
this.root = root;
}
/** {@inheritDoc} */
public void setDocumentAsImplemeted(String location) throws Exception{ }
@Override
public DocumentNode getSpecificationsHierarchy(String project, String systemUnderTest) throws IOException {
if (root == null) {
return new DocumentNode("");
}
Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy