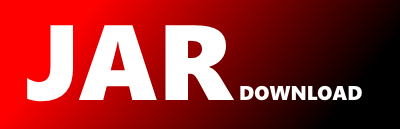
com.greenpepper.expectation.ShouldBe Maven / Gradle / Ivy
/*
* Copyright (c) 2006 Pyxis Technologies inc.
*
* This is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA,
* or see the FSF site: http://www.fsf.org.
*/
package com.greenpepper.expectation;
import com.greenpepper.util.Factory;
import java.util.LinkedList;
import java.util.List;
/**
* ShouldBe class.
*
* @version $Revision: $ $Date: $
* @author oaouattara
*/
public final class ShouldBe
{
/** Constant TRUE
*/
public static final Expectation TRUE = new EqualExpectation(true);
/** Constant FALSE
*/
public static final Expectation FALSE = new EqualExpectation(false);
/** Constant NULL
*/
public static final Expectation NULL = new NullExpectation();
/** Constant NULL
*/
public static final Expectation NOT_NULL = new NotNullExpectation();
private static List> factories = new LinkedList>();
static
{
register(IsInstanceExpectation.class);
register(ErrorExpectation.class);
register(NullExpectation.class);
register(NotNullExpectation.class);
}
private ShouldBe()
{
}
/**
* register.
*
* @param factoryClass a {@link java.lang.Class} object.
*/
public static void register(Class extends Expectation> factoryClass)
{
factories.add(new Factory(factoryClass));
}
/**
* equal.
*
* @param o a {@link java.lang.Object} object.
* @return a {@link com.greenpepper.expectation.Expectation} object.
*/
public static Expectation equal(Object o)
{
return new EqualExpectation(o);
}
/**
* instanceOf.
*
* @param c a {@link java.lang.Class} object.
* @return a {@link com.greenpepper.expectation.Expectation} object.
*/
public static Expectation instanceOf(Class c)
{
return new IsInstanceExpectation(c);
}
/**
* literal.
*
* @param expected a {@link java.lang.String} object.
* @return a {@link com.greenpepper.expectation.Expectation} object.
*/
public static Expectation literal(String expected)
{
if (expected == null)
throw new NullPointerException("expected");
for (int i = factories.size() - 1; i >= 0; i--)
{
Factory factory = factories.get(i);
Expectation expectation = factory.newInstance(expected);
if (expectation != null)
return expectation;
}
return new DuckExpectation(expected);
}
/**
* either.
*
* @param expectation a {@link com.greenpepper.expectation.Expectation} object.
* @return a {@link com.greenpepper.expectation.Either} object.
*/
public static Either either(Expectation expectation)
{
return new Either(expectation);
}
/**
* not.
*
* @param expectation a {@link com.greenpepper.expectation.Expectation} object.
* @return a {@link com.greenpepper.expectation.Expectation} object.
*/
public static Expectation not(Expectation expectation)
{
return new NotExpectation(expectation);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy