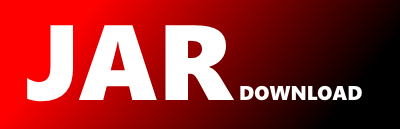
com.greenpepper.repository.ReferenceNode Maven / Gradle / Ivy
package com.greenpepper.repository;
import com.greenpepper.shaded.org.apache.commons.lang3.StringUtils;
import java.util.List;
import java.util.Vector;
import static com.greenpepper.shaded.org.apache.commons.lang3.StringUtils.isNotBlank;
/**
* ReferenceNode class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class ReferenceNode extends DocumentNode implements Marshalizable
{
/** Constant NODE_REPOSITORY_UID_INDEX=4
*/
private final static int NODE_REPOSITORY_UID_INDEX = 4;
/** Constant NODE_SUT_NAME_INDEX=5
*/
private final static int NODE_SUT_NAME_INDEX = 5;
/** Constant NODE_SECTION_INDEX=6
*/
private final static int NODE_SECTION_INDEX = 6;
private static final int NODE_URL_INDEX = 7;
private String repositoryUID;
private String sutName;
private String section;
/**
* Constructor for ReferenceNode.
*
* @param title a {@link java.lang.String} object.
* @param repositoryUID a {@link java.lang.String} object.
* @param sutName a {@link java.lang.String} object.
* @param section a {@link java.lang.String} object.
*/
public ReferenceNode(String title, String repositoryUID, String sutName, String section)
{
super(title);
this.repositoryUID = repositoryUID;
this.sutName = sutName;
this.section = section;
}
/**
* Getter for the field repositoryUID
.
*
* @return a {@link java.lang.String} object.
*/
public String getRepositoryUID()
{
return repositoryUID;
}
/**
* Getter for the field sutName
.
*
* @return a {@link java.lang.String} object.
*/
public String getSutName()
{
return sutName;
}
/**
* Getter for the field section
.
*
* @return a {@link java.lang.String} object.
*/
public String getSection()
{
return section;
}
/** {@inheritDoc} */
@Override
public void addChildren(DocumentNode child)
{
throw new RuntimeException("Reference node should not have children");
}
/** {@inheritDoc} */
@Override
public Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy