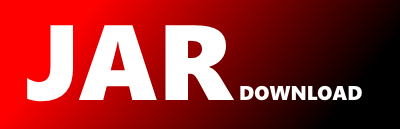
com.greenpepper.server.domain.RequirementSummary Maven / Gradle / Ivy
package com.greenpepper.server.domain;
import com.greenpepper.repository.Marshalizable;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.SUMMARY_ERRORS_IDX;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.SUMMARY_EXCEPTION_IDX;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.SUMMARY_FAILIURES_IDX;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.SUMMARY_SUCCESS_IDX;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.SUMMARY_REFERENCES_IDX;
import java.util.Vector;
/**
* RequirementSummary class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class RequirementSummary implements Marshalizable
{
private int referencesSize = 0;
private int success = 0;
private int errors = 0;
private int failures = 0;
private int exceptions = 0;
/**
* Constructor for RequirementSummary.
*/
public RequirementSummary(){ }
/**
* Getter for the field errors
.
*
* @return a int.
*/
public int getErrors()
{
return errors;
}
/**
* Setter for the field errors
.
*
* @param errors a int.
*/
public void setErrors(int errors)
{
this.errors = errors;
}
/**
* Getter for the field exceptions
.
*
* @return a int.
*/
public int getExceptions()
{
return exceptions;
}
/**
* Setter for the field exceptions
.
*
* @param exceptions a int.
*/
public void setExceptions(int exceptions)
{
this.exceptions = exceptions;
}
/**
* Getter for the field failures
.
*
* @return a int.
*/
public int getFailures()
{
return failures;
}
/**
* Setter for the field failures
.
*
* @param failures a int.
*/
public void setFailures(int failures)
{
this.failures = failures;
}
/**
* Getter for the field success
.
*
* @return a int.
*/
public int getSuccess()
{
return success;
}
/**
* Setter for the field success
.
*
* @param success a int.
*/
public void setSuccess(int success)
{
this.success = success;
}
/**
* Getter for the field referencesSize
.
*
* @return a int.
*/
public int getReferencesSize()
{
return referencesSize;
}
/**
* Setter for the field referencesSize
.
*
* @param referencesSize a int.
*/
public void setReferencesSize(int referencesSize)
{
this.referencesSize = referencesSize;
}
/**
* addErrors.
*
* @param errors a int.
*/
public void addErrors(int errors)
{
this.errors += errors;
}
/**
* addSuccess.
*
* @param success a int.
*/
public void addSuccess(int success)
{
this.success += success;
}
/**
* addFailures.
*
* @param failures a int.
*/
public void addFailures(int failures)
{
this.failures += failures;
}
/**
* addException.
*
* @param hasException a boolean.
*/
public void addException(boolean hasException)
{
if(hasException)exceptions++;
}
/**
* getTestsIgnored.
*
* @return a boolean.
*/
public boolean getTestsIgnored()
{
return success == 0 && !getTestsFailed();
}
/**
* getTestsFailed.
*
* @return a boolean.
*/
public boolean getTestsFailed()
{
return failures + errors + exceptions > 0;
}
/**
* getTestsSucceeded.
*
* @return a boolean.
*/
public boolean getTestsSucceeded()
{
return success > 0 && !getTestsFailed();
}
/**
* marshallize.
*
* @return a {@link java.util.Vector} object.
*/
public Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy