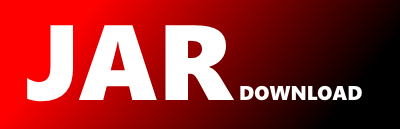
com.greenpepper.server.domain.Specification Maven / Gradle / Ivy
package com.greenpepper.server.domain;
import java.util.HashSet;
import java.util.Set;
import java.util.Vector;
import java.util.TreeSet;
import java.util.SortedSet;
import javax.persistence.CascadeType;
import javax.persistence.Entity;
import javax.persistence.JoinColumn;
import javax.persistence.JoinTable;
import javax.persistence.ManyToMany;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import javax.persistence.Transient;
import javax.persistence.UniqueConstraint;
import org.hibernate.annotations.Sort;
import org.hibernate.annotations.SortType;
import com.greenpepper.server.GreenPepperServerErrorKey;
import com.greenpepper.server.GreenPepperServerException;
import com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.SPECIFICATION_DIALECT_IDX;
import static com.greenpepper.server.rpc.xmlrpc.XmlRpcDataMarshaller.SPECIFICATION_SUTS_IDX;
import static org.apache.commons.lang3.StringUtils.isNotBlank;
/**
* Specification Class.
*
* Copyright (c) 2006 Pyxis technologies inc. All Rights Reserved.
*
* @author JCHUET
* @version $Id: $Id
*/
@Entity
@Table(name="SPECIFICATION", uniqueConstraints = {@UniqueConstraint(columnNames={"NAME", "REPOSITORY_ID"})})
@SuppressWarnings("serial")
public class Specification extends Document
{
private SortedSet targetedSystemUnderTests = new TreeSet();
protected Set references = new HashSet();
private Set executions = new HashSet();
@Transient
private String dialectClass;
/**
* newInstance.
*
* @param name a {@link java.lang.String} object.
* @return a {@link com.greenpepper.server.domain.Specification} object.
*/
public static Specification newInstance(String name)
{
Specification specification = new Specification();
specification.setName(name);
return specification;
}
/**
* Getter for the field targetedSystemUnderTests
.
*
* @return a {@link java.util.SortedSet} object.
*/
@ManyToMany( targetEntity= SystemUnderTest.class, cascade={CascadeType.PERSIST, CascadeType.MERGE} )
@JoinTable( name="SUT_SPECIFICATION", joinColumns={@JoinColumn(name="SPECIFICATION_ID")}, inverseJoinColumns={@JoinColumn(name="SUT_ID")} )
@Sort(type=SortType.COMPARATOR, comparator=SystemUnderTestByNameComparator.class)
public SortedSet getTargetedSystemUnderTests()
{
return targetedSystemUnderTests;
}
/**
* Getter for the field executions
.
*
* @return a {@link java.util.Set} object.
*/
@OneToMany(mappedBy="specification", cascade=CascadeType.ALL)
public Set getExecutions()
{
return this.executions;
}
/**
* Getter for the field references
.
*
* @return a {@link java.util.Set} object.
*/
@OneToMany(mappedBy="specification", cascade=CascadeType.ALL)
public Set getReferences()
{
return references;
}
/**
* Setter for the field targetedSystemUnderTests
.
*
* @param targetedSystemUnderTests a {@link java.util.SortedSet} object.
*/
public void setTargetedSystemUnderTests(SortedSet targetedSystemUnderTests)
{
this.targetedSystemUnderTests = targetedSystemUnderTests;
}
/**
* Setter for the field executions
.
*
* @param executions a {@link java.util.Set} object.
*/
public void setExecutions(Set executions)
{
this.executions = executions;
}
/**
* Setter for the field references
.
*
* @param references a {@link java.util.Set} object.
*/
public void setReferences(Set references)
{
this.references = references;
}
/**
* addSystemUnderTest.
*
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
*/
public void addSystemUnderTest(SystemUnderTest systemUnderTest)
{
targetedSystemUnderTests.add(systemUnderTest);
}
/**
* addExecution.
*
* @param execution a {@link com.greenpepper.server.domain.Execution} object.
*/
public void addExecution(Execution execution)
{
execution.setSpecification(this);
executions.add(execution);
}
/**
* removeSystemUnderTest.
*
* @param systemUnderTest a {@link com.greenpepper.server.domain.SystemUnderTest} object.
*/
public void removeSystemUnderTest(SystemUnderTest systemUnderTest)
{
targetedSystemUnderTests.remove(systemUnderTest);
if(targetedSystemUnderTests.isEmpty())
addSystemUnderTest(getRepository().getProject().getDefaultSystemUnderTest());
}
/**
* removeReference.
*
* @param reference a {@link com.greenpepper.server.domain.Reference} object.
* @throws com.greenpepper.server.GreenPepperServerException if any.
*/
public void removeReference(Reference reference) throws GreenPepperServerException
{
if(!references.contains(reference))
{
throw new GreenPepperServerException( GreenPepperServerErrorKey.REFERENCE_NOT_FOUND, "Reference not found");
}
references.remove(reference);
reference.setSpecification(null);
}
/**
* marshallize.
*
* @return a {@link java.util.Vector} object.
*/
public Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy