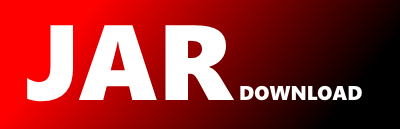
com.greenpepper.ogn.ObjectGraphNavigationFixture Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2007 Pyxis Technologies inc.
*
* This is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA,
* or see the FSF site: http://www.fsf.org.
*/
package com.greenpepper.ogn;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.LinkedList;
import java.util.List;
import com.greenpepper.reflect.AbstractFixture;
import com.greenpepper.reflect.Fixture;
import com.greenpepper.reflect.Message;
import com.greenpepper.reflect.PlainOldFixture;
/**
* ObjectGraphNavigationFixture class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class ObjectGraphNavigationFixture extends AbstractFixture implements ObjectGraphNavigationMessageResolver
{
/**
* Constructor for ObjectGraphNavigationFixture.
*
* @param target a {@link java.lang.Object} object.
*/
public ObjectGraphNavigationFixture(Object target)
{
super(target);
}
/** {@inheritDoc} */
public Fixture fixtureFor( Object target )
{
return new ObjectGraphNavigationFixture( target );
}
/** {@inheritDoc} */
protected Message getCheckMessage( String name )
{
PlainOldFixture plainOldFixture = new PlainOldFixture( target );
if ( plainOldFixture.canCheck( name ) )
{
return plainOldFixture.check( name );
}
return getMessage(name, true, true);
}
/** {@inheritDoc} */
protected Message getSendMessage( String name )
{
PlainOldFixture plainOldFixture = new PlainOldFixture( target );
if ( plainOldFixture.canSend( name ) )
{
return plainOldFixture.send( name );
}
return getMessage(name, false, true);
}
/** {@inheritDoc} */
public Message resolve(ObjectGraphNavigationInfo info)
{
if (info.getTarget() == null)
{
return resolveWithPlainOldFixture(info);
}
else
{
Class clazz = target.getClass();
String[] splits = info.getTarget().split("\\.");
LinkedList invocations = new LinkedList();
for (String split : splits) {
List methods = getMethods(clazz, split);
Method method = methods != null && methods.size() > 0 ? methods.get(0) : null;
if (method == null)
{
method = getGetter(clazz, split);
}
if (method == null)
{
Field field = getField(clazz, split);
if (field == null)
{
break;
}
else
{
clazz = field.getType();
invocations.add(new ObjectGraphFieldInvocation(field, true));
}
}
else
{
clazz = method.getReturnType();
invocations.add(new ObjectGraphMethodInvocation(method, info.isGetter()));
}
}
if (invocations.size() == splits.length)
{
ObjectGraphInvocable methodCall = getInvocation(clazz, info.getMethodName(), info.isGetter());
if (methodCall != null)
{
invocations.add(methodCall);
return new ObjectGraphNavigationMessage(target, invocations);
}
}
}
return null;
}
private Message resolveWithPlainOldFixture(ObjectGraphNavigationInfo info)
{
PlainOldFixture fixture = new PlainOldFixture(target);
if (info.isGetter())
{
if (fixture.canCheck(info.getMethodName()))
{
return fixture.check(info.getMethodName());
}
}
else
{
if (fixture.canSend(info.getMethodName()))
{
return fixture.send(info.getMethodName());
}
}
return null;
}
private Message getMessage(String name, boolean isGetter, boolean checkSut)
{
ObjectGraphNavigation graph = new ObjectGraphNavigation(isGetter, this);
Message message = graph.resolveMessage(name);
if (message != null)
{
return message;
}
if (checkSut)
{
if (getSystemUnderTest() == null) return null;
ObjectGraphNavigationFixture fixture = new ObjectGraphNavigationFixture( getSystemUnderTest() );
return isGetter ? fixture.getCheckMessage(name) : fixture.getSendMessage(name);
}
return null;
}
private ObjectGraphInvocable getInvocation(Class clazz, String name, boolean isGetter)
{
List methods = getMethods(clazz, name);
Method method = methods != null && methods.size() > 0 ? methods.get(0) : null;
if (method == null)
{
method = isGetter ? getGetter(clazz, name) : getSetter(clazz, name);
}
if (method == null)
{
Field field = getField(clazz, name);
if (field != null)
{
return new ObjectGraphFieldInvocation(field, isGetter);
}
}
else
{
return new ObjectGraphMethodInvocation(method, isGetter);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy