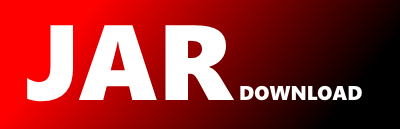
com.sun.xml.xhtml.XhtmlCatalog Maven / Gradle / Ivy
/*
* $Id: XhtmlCatalog.java,v 1.1 1999/04/04 18:57:00 db Exp $
*
* Copyright (c) 1999 Sun Microsystems, Inc. All Rights Reserved.
*
* This software is the confidential and proprietary information of Sun
* Microsystems, Inc. ("Confidential Information"). You shall not
* disclose such Confidential Information and shall use it only in
* accordance with the terms of the license agreement you entered into
* with Sun.
*
* SUN MAKES NO REPRESENTATIONS OR WARRANTIES ABOUT THE SUITABILITY OF THE
* SOFTWARE, EITHER EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
* IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
* PURPOSE, OR NON-INFRINGEMENT. SUN SHALL NOT BE LIABLE FOR ANY DAMAGES
* SUFFERED BY LICENSEE AS A RESULT OF USING, MODIFYING OR DISTRIBUTING
* THIS SOFTWARE OR ITS DERIVATIVES.
*/
package com.sun.xml.xhtml;
import com.sun.xml.parser.Resolver;
/**
* This class provides access to Java resources used to support fast
* access to the DTDs (strict, transitional, frameset) used in the
* XHTML
* working draft. Access to the predefined ISO Latin/1, symbol, and
* mathematical entities is also provided.
*
* Two access methods are provided. The most convenient method
* to start with is to use the static
* configureResolver method, and to use such a resolver with a
* SAX parser. Since there may be cases where that method can't be
* used, static constants are also provided for the PUBLIC ids of
* those DTDs (and entities), and for the associated resource.
*
*
Note that there are issues with the above working draft which
* require workarounds. In particular, there is confusion about just
* what a namespace is for -- the consensus appears to be that XHTML
* itself should be the namespace, with the three DTD variants only
* used to constrain file syntax rather than to define three distinct
* namespaces. Coupled with that problem is that there are no public
* identifiers defined for those DTDs. In this file, the following
* public identifiers are temporarily being used for the DTDS, pending
* assignment of such identifiers by the W3C:
*
* - "-//Sun//DTD XHTML WD 1990304//EN" ... identifies
* the "strict" DTD
*
- "-//Sun//DTD XHTML WD 1990304 Transitional//EN" ... identifies
* the "transitional" DTD
*
- "-//Sun//DTD XHTML WD 1990304 Frameset//EN" ... identifies
* the "frameset" DTD
*
*
*
* This means that an XHTML document using the "strict" DTD could
* be written with doctype containing a PUBLIC id of
* XHTMLtransitional_FPI and
* using XHTMLtransitional_URI
* for its system ID. It could then avoid accessing that URI (with
* associated network delays to Boston, Massachussetts) by using a
* SAX EntityResolver configured to use the local Java resource named
* XHTMLtransitional_resource.
*
*
That is, with an XHTML document that begins with
* <!DOCTYPE html PUBLIC
* "-//Sun//DTD XHTML WD 1990304//EN";
* "http://www.w3.org/TR/1999/WD-html-in-xml-1990304/DTD/xhtml1-strict.dtd"
* >
and with a properly configured resolver, the DTD could be
* locally processed, without any remote network accesses needed.
*
* @see com.sun.xml.parser.Resolver
*
* @author David Brownell
* @version $Revision: 1.1 $
*/
public class XhtmlCatalog
{
// no instances allowed
private XhtmlCatalog () {}
//
// fixed prefixes
//
private static final String RSRCbase
= "com/sun/xml/xhtml/resources";
private static final String DTDbase
= "http://www.w3.org/TR/1999/WD-html-in-xml-1990304/DTD/";
/**
* Configures the specified resolver with mappings from the PUBLIC
* identifiers used in the XHTML specification (as noted above) and
* resources found in this package, facilitating fast non-networked
* access to these DTDs.
*/
public static void configureResolver (Resolver resolver)
{
ClassLoader loader = XhtmlCatalog.class.getClassLoader ();
resolver.registerCatalogEntry (
HTMLlat1x_FPI, HTMLlat1x_resource,
loader);
resolver.registerCatalogEntry (
HTMLspecialx_FPI, HTMLspecialx_resource,
loader);
resolver.registerCatalogEntry (
HTMLsymbolx_FPI, HTMLsymbolx_resource,
loader);
resolver.registerCatalogEntry (
XHTMLstrict_FPI, XHTMLstrict_resource,
loader);
resolver.registerCatalogEntry (
XHTMLtransitional_FPI, XHTMLtransitional_resource,
loader);
resolver.registerCatalogEntry (
XHTMLframeset_FPI, XHTMLframeset_resource,
loader);
}
//
// PREDEFINED INTERNAL ENTITIES
//
/** (Formal) PUBLIC Identifier for ISO Latin/1 entities */
public static final String HTMLlat1x_FPI
= "-//W3C//ENTITIES Latin1//EN//HTML";
/** Java resource name for HTMLlat1x_FPI */
public static final String HTMLlat1x_resource
= RSRCbase + "x.ent";
/** SYSTEM URI for HTMLlat1x_FPI */
public static final String HTMLlat1x_uri
= DTDbase + "HTMLlat1x.ent";
/** (Formal) PUBLIC Identifier for Special entities */
public static final String HTMLspecialx_FPI
= "-//W3C//ENTITIES Special//EN//HTML";
/** Java resource name for HTMLspecialx_FPI */
public static final String HTMLspecialx_resource
= RSRCbase + "x.ent";
/** SYSTEM URI for HTMLspecialx_FPI */
public static final String HTMLspecialx_uri
= DTDbase + "HTMLspecialx.ent";
/** (Formal) PUBLIC Identifier for Symbol entities */
public static final String HTMLsymbolx_FPI
= "-//W3C//ENTITIES Symbolic//EN//HTML";
/** Java resource name for HTMLsymbolx_FPI */
public static final String HTMLsymbolx_resource
= RSRCbase + "x.ent";
/** SYSTEM URI for HTMLsymbolx_FPI */
public static final String HTMLsymbolx_uri
= DTDbase + "HTMLsymbolx.ent";
//
// FILES WITH XHTML DTD DECLARATIONS
//
/** (Formal) PUBLIC Identifier for XHTML "strict" DTD */
public static final String XHTMLstrict_FPI
= "-//Sun//DTD XHTML WD 1990304//EN";
/** Java resource name for XHTMLstrict_FPI */
public static final String XHTMLstrict_resource
= RSRCbase + "xhtml1-strict.dtd";
/** SYSTEM URI for XHTMLstrict_FPI */
public static final String XHTMLstrict_uri
= DTDbase + "xhtml1-strict.dtd";
/** (Formal) PUBLIC Identifier for XHTML "transitional" DTD */
public static final String XHTMLtransitional_FPI
= "-//Sun//DTD XHTML WD 1990304 Transitional//EN";
/** Java resource name for XHTMLtransitional_FPI */
public static final String XHTMLtransitional_resource
= RSRCbase + "xhtml1-transitional.dtd";
/** SYSTEM URI for XHTMLtransitional_FPI */
public static final String XHTMLtransitional_uri
= DTDbase + "xhtml1-transitional.dtd";
/** (Formal) PUBLIC Identifier for XHTML "frameset" DTD */
public static final String XHTMLframeset_FPI
= "-//Sun//DTD XHTML WD 1990304 Frameset//EN";
/** Java resource name for XHTMLframeset_FPI */
public static final String XHTMLframeset_resource
= RSRCbase + "xhtml1-frameset.dtd";
/** SYSTEM URI for XHTMLframeset_FPI */
public static final String XHTMLframeset_uri
= DTDbase + "xhtml1-frameset.dtd";
}