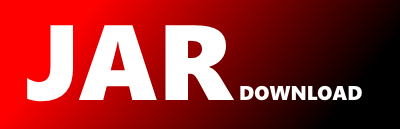
org.w3c.dom.Document Maven / Gradle / Ivy
// Copyright (c) 1998 by W3C
//
// DOM is a trademark of W3C
// The DOM level 1 specification, from which this
// source is derived, is copyright by W3C.
// See: http://www.w3.org/TR/REC-DOM-Level-1/
//
package org.w3c.dom;
/**
The Document
interface represents the entire
HTML or XML document. Conceptually, it is the root of the
document tree, and provides the primary access to the
document's data.
Since elements, text nodes, comments, processing instructions,
etc. cannot exist outside the context of a
Document
, the Document
interface also
contains the factory methods needed to create these objects.
The Node
objects created have a ownerDocument
attribute which associates them with the Document
within whose
context they were created.
Property Summary
doctype
getDoctype
The Document Type Declaration (see DocumentType
)
associated with
this document. For HTML documents as well as XML documents without a
document type declaration this returns null
. The DOM Level
1 does not support editing the Document Type Declaration, therefore
docType
cannot be altered in any way.
implementation
getImplementation
The DOMImplementation
object that handles this
document. A DOM application may use objects from multiple
implementations.
documentElement
getDocumentElement
This is a convenience attribute that allows direct
access to the child node that is the root element of the
document. For HTML documents, this is the element with
the tagName "HTML".
*/
public interface Document
extends Node
{
/**
* Returns the value of the doctype
property.
*/
DocumentType getDoctype ();
/**
* Returns the value of the implementation
property.
*/
DOMImplementation getImplementation ();
/**
* Returns the value of the documentElement
property.
*/
Element getDocumentElement ();
/**
Creates an element of the type specified. Note that the
instance returned implements the Element interface, so
attributes can be specified directly on the returned
object.
@return A new Element
object.
@param tagName
The name of the element type to
instantiate. For XML, this is case-sensitive. For HTML, the
tagName
parameter may be provided in any case,
but it must be mapped to the canonical uppercase form by
the DOM implementation.
@exception INVALID_CHARACTER_ERR: Raised if the specified name contains
an invalid character.
*/
Element createElement (String tagName) throws DOMException;
/**
Creates an empty DocumentFragment
object.
@return A new DocumentFragment
.
*/
DocumentFragment createDocumentFragment ();
/**
Creates a Text
node given the specified
string.
@return The new Text
object.
@param data
The data for the node.
*/
Text createTextNode (String data);
/**
Creates a Comment
node given the specified
string.
@return The new Comment
object.
@param data
The data for the node.
*/
Comment createComment (String data);
/**
Creates a CDATASection
node whose value is
the specified string.
@return The new CDATASection
object.
@param data
The data for the CDATASection
contents.
@exception NOT_SUPPORTED_ERR: Raised if this document is an HTML
document.
*/
CDATASection createCDATASection (String data) throws DOMException;
/**
Creates a ProcessingInstruction
node given
the specified name and data strings.
@return The new ProcessingInstruction
object.
@param target
The target part of the processing instruction.
@param data
The data for the node.
@exception INVALID_CHARACTER_ERR: Raised if an invalid character is specified.
NOT_SUPPORTED_ERR: Raised if this document is an HTML document.
*/
ProcessingInstruction createProcessingInstruction (String target, String data) throws DOMException;
/**
Creates an Attr
of the given name.
Note that the Attr
instance
can then be set on an Element
using the
setAttribute
method.
@return A new Attr
object.
@param name
The name of the attribute.
@exception INVALID_CHARACTER_ERR: Raised if the specified name contains
an invalid character.
*/
Attr createAttribute (String name) throws DOMException;
/**
Creates an EntityReference object.
@return The new EntityReference
object.
@param name
The name of the entity to reference.
@exception INVALID_CHARACTER_ERR: Raised if the specified name contains
an invalid character.
NOT_SUPPORTED_ERR: Raised if this document is an HTML document.
*/
EntityReference createEntityReference (String name) throws DOMException;
/**
Returns a NodeList
of all the Element
s
with a given tag name in the order in which they would be encountered
in a preorder traversal of the Document
tree.
@return A new NodeList
object containing
all the matched Element
s.
@param tagname
The name of the tag to match on. The special value "*"
matches all tags.
*/
NodeList getElementsByTagName (String tagname);
}