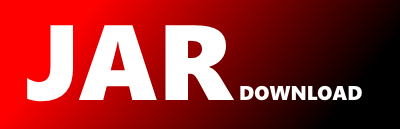
org.w3c.dom.html.HTMLObjectElement Maven / Gradle / Ivy
// Copyright (c) 1998 by W3C
//
// DOM is a trademark of W3C
// The DOM level 1 specification, from which this
// source is derived, is copyright by W3C.
// See: http://www.w3.org/TR/REC-DOM-Level-1/
//
package org.w3c.dom.html;
import org.w3c.dom.*;
/**
Generic embedded object.
Note. In principle, all properties on the object element are read-write but in some environments some properties may be read-only once the underlying object is instantiated. See the
OBJECT element definition
in HTML 4.0.
Property Summary
form
getForm
Returns the
FORM
element containing this control. Returns null if this control is not within the context of a form.
code
getCode
setCode
Applet class file. See the
code
attribute for HTMLAppletElement.
align
getAlign
setAlign
Aligns this object (vertically or horizontally) with respect to its surrounding text. See the
align attribute definition
in HTML 4.0. This attribute is deprecated in HTML 4.0.
archive
getArchive
setArchive
Space-separated list of archives. See the
archive attribute definition
in HTML 4.0.
border
getBorder
setBorder
Width of border around the object. See the
border attribute definition
in HTML 4.0. This attribute is deprecated in HTML 4.0.
codeBase
getCodeBase
setCodeBase
Base URI for
classid
,
data
, and
archive
attributes. See the
codebase attribute definition
in HTML 4.0.
codeType
getCodeType
setCodeType
Content type for data downloaded via
classid
attribute. See the
codetype attribute definition
in HTML 4.0.
data
getData
setData
A URI specifying the location of the object's data. See the
data attribute definition
in HTML 4.0.
declare
getDeclare
setDeclare
Declare (for future reference), but do not instantiate, this object. See the
declare attribute definition
in HTML 4.0.
height
getHeight
setHeight
Override height. See the
height attribute definition
in HTML 4.0.
hspace
getHspace
setHspace
Horizontal space to the left and right of this image, applet, or object. See the
hspace attribute definition
in HTML 4.0. This attribute is deprecated in HTML 4.0.
name
getName
setName
Form control or object name when submitted with a form. See the
name attribute definition
in HTML 4.0.
standby
getStandby
setStandby
Message to render while loading the object. See the
standby attribute definition
in HTML 4.0.
tabIndex
getTabIndex
setTabIndex
Index that represents the element's position in the tabbing order. See the
tabindex attribute definition
in HTML 4.0.
type
getType
setType
Content type for data downloaded via
data
attribute. See the
type attribute definition
in HTML 4.0.
useMap
getUseMap
setUseMap
Use client-side image map. See the
usemap attribute definition
in HTML 4.0.
vspace
getVspace
setVspace
Vertical space above and below this image, applet, or object. See the
vspace attribute definition
in HTML 4.0. This attribute is deprecated in HTML 4.0.
width
getWidth
setWidth
Override width. See the
width attribute definition
in HTML 4.0.
*/
public interface HTMLObjectElement
extends HTMLElement
{
/**
* Returns the value of the form
property.
*/
HTMLFormElement getForm ();
/** Assigns the value of the code
property.
*/
void setCode (String code);
/**
* Returns the value of the code
property.
*/
String getCode ();
/** Assigns the value of the align
property.
*/
void setAlign (String align);
/**
* Returns the value of the align
property.
*/
String getAlign ();
/** Assigns the value of the archive
property.
*/
void setArchive (String archive);
/**
* Returns the value of the archive
property.
*/
String getArchive ();
/** Assigns the value of the border
property.
*/
void setBorder (String border);
/**
* Returns the value of the border
property.
*/
String getBorder ();
/** Assigns the value of the codeBase
property.
*/
void setCodeBase (String codeBase);
/**
* Returns the value of the codeBase
property.
*/
String getCodeBase ();
/** Assigns the value of the codeType
property.
*/
void setCodeType (String codeType);
/**
* Returns the value of the codeType
property.
*/
String getCodeType ();
/** Assigns the value of the data
property.
*/
void setData (String data);
/**
* Returns the value of the data
property.
*/
String getData ();
/** Assigns the value of the declare
property.
*/
void setDeclare (boolean declare);
/**
* Returns the value of the declare
property.
*/
boolean getDeclare ();
/** Assigns the value of the height
property.
*/
void setHeight (String height);
/**
* Returns the value of the height
property.
*/
String getHeight ();
/** Assigns the value of the hspace
property.
*/
void setHspace (String hspace);
/**
* Returns the value of the hspace
property.
*/
String getHspace ();
/** Assigns the value of the name
property.
*/
void setName (String name);
/**
* Returns the value of the name
property.
*/
String getName ();
/** Assigns the value of the standby
property.
*/
void setStandby (String standby);
/**
* Returns the value of the standby
property.
*/
String getStandby ();
/** Assigns the value of the tabIndex
property.
*/
void setTabIndex (int tabIndex);
/**
* Returns the value of the tabIndex
property.
*/
int getTabIndex ();
/** Assigns the value of the type
property.
*/
void setType (String type);
/**
* Returns the value of the type
property.
*/
String getType ();
/** Assigns the value of the useMap
property.
*/
void setUseMap (String useMap);
/**
* Returns the value of the useMap
property.
*/
String getUseMap ();
/** Assigns the value of the vspace
property.
*/
void setVspace (String vspace);
/**
* Returns the value of the vspace
property.
*/
String getVspace ();
/** Assigns the value of the width
property.
*/
void setWidth (String width);
/**
* Returns the value of the width
property.
*/
String getWidth ();
}