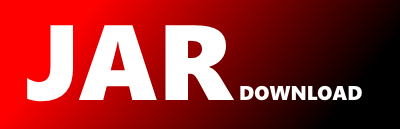
com.greenpepper.server.GreenPepperServerServiceImpl Maven / Gradle / Ivy
/*
* Copyright (c) 2008 Pyxis Technologies inc.
* This is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA,
* or see the FSF site: http://www.fsf.org.
*/
package com.greenpepper.server;
import static com.greenpepper.server.GreenPepperServerErrorKey.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Vector;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.greenpepper.report.XmlReport;
import com.greenpepper.repository.DocumentRepository;
import com.greenpepper.server.database.SessionService;
import com.greenpepper.repository.DocumentNode;
import com.greenpepper.server.domain.EnvironmentType;
import com.greenpepper.server.domain.Execution;
import com.greenpepper.server.domain.Project;
import com.greenpepper.server.domain.Reference;
import com.greenpepper.repository.ReferenceNode;
import com.greenpepper.server.domain.Repository;
import com.greenpepper.server.domain.Requirement;
import com.greenpepper.server.domain.RequirementSummary;
import com.greenpepper.server.domain.Runner;
import com.greenpepper.server.domain.Specification;
import com.greenpepper.server.domain.SystemUnderTest;
import com.greenpepper.server.domain.component.ContentType;
import com.greenpepper.server.domain.dao.DocumentDao;
import com.greenpepper.server.domain.dao.ProjectDao;
import com.greenpepper.server.domain.dao.RepositoryDao;
import com.greenpepper.server.domain.dao.SystemUnderTestDao;
import com.greenpepper.util.StringUtil;
/**
* GreenPepperServerServiceImpl class.
*
* @author oaouattara
* @version $Id: $Id
*/
public class GreenPepperServerServiceImpl implements GreenPepperServerService {
private static Logger log = LoggerFactory.getLogger(GreenPepperServerServiceImpl.class);
private SessionService sessionService;
private ProjectDao projectDao;
private RepositoryDao repositoryDao;
private SystemUnderTestDao sutDao;
private DocumentDao documentDao;
/**
* Constructor for GreenPepperServerServiceImpl.
*
* @param sessionService a {@link com.greenpepper.server.database.SessionService} object.
* @param projectDao a {@link com.greenpepper.server.domain.dao.ProjectDao} object.
* @param repositoryDao a {@link com.greenpepper.server.domain.dao.RepositoryDao} object.
* @param sutDao a {@link com.greenpepper.server.domain.dao.SystemUnderTestDao} object.
* @param documentDao a {@link com.greenpepper.server.domain.dao.DocumentDao} object.
*/
@SuppressWarnings("unused")
public GreenPepperServerServiceImpl(SessionService sessionService, ProjectDao projectDao, RepositoryDao repositoryDao, SystemUnderTestDao sutDao,
DocumentDao documentDao) {
this.sessionService = sessionService;
this.projectDao = projectDao;
this.repositoryDao = repositoryDao;
this.sutDao = sutDao;
this.documentDao = documentDao;
}
/**
* Constructor for GreenPepperServerServiceImpl.
*/
@SuppressWarnings("unused")
public GreenPepperServerServiceImpl() {
}
/**
* Setter for the field sessionService
.
*
* @param sessionService a {@link com.greenpepper.server.database.SessionService} object.
*/
public void setSessionService(SessionService sessionService) {
this.sessionService = sessionService;
}
/**
* Setter for the field projectDao
.
*
* @param projectDao a {@link com.greenpepper.server.domain.dao.ProjectDao} object.
*/
public void setProjectDao(ProjectDao projectDao) {
this.projectDao = projectDao;
}
/**
* Setter for the field repositoryDao
.
*
* @param repositoryDao a {@link com.greenpepper.server.domain.dao.RepositoryDao} object.
*/
public void setRepositoryDao(RepositoryDao repositoryDao) {
this.repositoryDao = repositoryDao;
}
/**
* Setter for the field sutDao
.
*
* @param sutDao a {@link com.greenpepper.server.domain.dao.SystemUnderTestDao} object.
*/
public void setSutDao(SystemUnderTestDao sutDao) {
this.sutDao = sutDao;
}
/**
* Setter for the field documentDao
.
*
* @param documentDao a {@link com.greenpepper.server.domain.dao.DocumentDao} object.
*/
public void setDocumentDao(DocumentDao documentDao) {
this.documentDao = documentDao;
}
/**
* getAllEnvironmentTypes.
*
* @inheritDoc NO NEEDS TO SECURE THIS
* @return a {@link java.util.List} object.
* @throws com.greenpepper.server.GreenPepperServerException if any.
*/
public List getAllEnvironmentTypes() throws GreenPepperServerException {
try {
sessionService.startSession();
List envTypes = sutDao.getAllEnvironmentTypes();
log.debug("Retrieved All Environment Types number: " + envTypes.size());
return envTypes;
} catch (Exception ex) {
throw handleException(ERROR, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Runner getRunner(String name) throws GreenPepperServerException {
try {
sessionService.startSession();
Runner runner = sutDao.getRunnerByName(name);
log.debug("Retrieved Runner name: " + name);
return runner;
} catch (Exception ex) {
throw handleException(ERROR, ex);
} finally {
sessionService.closeSession();
}
}
/**
* getAllRunners.
*
* @inheritDoc NO NEEDS TO SECURE THIS
* @return a {@link java.util.List} object.
* @throws com.greenpepper.server.GreenPepperServerException if any.
*/
public List getAllRunners() throws GreenPepperServerException {
try {
sessionService.startSession();
List runners = sutDao.getAllRunners();
log.debug("Retrieved All Runner number: " + runners.size());
return runners;
} catch (Exception ex) {
throw handleException(ERROR, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void createRunner(Runner runner) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
sutDao.create(runner);
sessionService.commitTransaction();
log.debug("Created Runner: " + runner.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(RUNNER_CREATE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void updateRunner(String oldRunnerName, Runner runner) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
sutDao.update(oldRunnerName, runner);
sessionService.commitTransaction();
log.debug("Updated Runner: " + oldRunnerName);
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(RUNNER_UPDATE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void removeRunner(String name) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
sutDao.removeRunner(name);
sessionService.commitTransaction();
log.debug("Removed Runner: " + name);
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(RUNNER_REMOVE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Repository getRepository(String uid, Integer maxUsers) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(uid);
if (maxUsers != null) {
repository.setMaxUsers(maxUsers);
}
return repository;
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Repository getRegisteredRepository(Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository registeredRepository = loadRepository(repository.getUid());
registeredRepository.setMaxUsers(repository.getMaxUsers());
return registeredRepository;
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Repository registerRepository(Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
Project project = projectDao.getByName(repository.getProject().getName());
if (project == null) {
projectDao.create(repository.getProject().getName());
}
repository = repositoryDao.create(repository);
sessionService.commitTransaction();
log.debug("Registered Repository: " + repository.getUid());
return repository;
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(REPOSITORY_REGISTRATION_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void updateRepositoryRegistration(Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
Project project = projectDao.getByName(repository.getProject().getName());
if (project == null) {
projectDao.create(repository.getProject().getName());
}
repositoryDao.update(repository);
sessionService.commitTransaction();
log.debug("Updated Repository: " + repository.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(REPOSITORY_UPDATE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void removeRepository(String repositoryUid) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
repositoryDao.remove(repositoryUid);
sessionService.commitTransaction();
log.debug("Removed Repository: " + repositoryUid);
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(REPOSITORY_REMOVE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getRepositoriesOfAssociatedProject(String projectName) throws GreenPepperServerException {
try {
sessionService.startSession();
return repositoryDao.getAll(projectName);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Project getProject(String name) throws GreenPepperServerException {
try {
sessionService.startSession();
Project project = projectDao.getByName(name);
log.debug("Retrieved Project name: " + name);
return project;
} finally {
sessionService.closeSession();
}
}
/**
* getAllProjects.
*
* @inheritDoc NO NEEDS TO SECURE THIS
* @return a {@link java.util.List} object.
*/
public List getAllProjects() {
try {
sessionService.startSession();
List projects = projectDao.getAll();
log.debug("Retrieved All Projects number: " + projects.size());
return projects;
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Project createProject(Project project) throws GreenPepperServerException {
Project newProject;
try {
sessionService.startSession();
sessionService.beginTransaction();
newProject = projectDao.create(project.getName());
sessionService.commitTransaction();
log.debug("Created Project: " + project.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(PROJECT_CREATE_FAILED, ex);
} finally {
sessionService.closeSession();
}
return newProject;
}
/** {@inheritDoc} */
public Project updateProject(String oldProjectName, Project project) throws GreenPepperServerException {
Project projectUpdated;
try {
sessionService.startSession();
sessionService.beginTransaction();
projectUpdated = projectDao.update(oldProjectName, project);
sessionService.commitTransaction();
log.debug("Updated Project: " + project.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(PROJECT_UPDATE_FAILED, ex);
} finally {
sessionService.closeSession();
}
return projectUpdated;
}
/**
* getAllSpecificationRepositories.
*
* @inheritDoc NO NEEDS TO SECURE THIS
* @return a {@link java.util.List} object.
* @throws com.greenpepper.server.GreenPepperServerException if any.
*/
public List getAllSpecificationRepositories() throws GreenPepperServerException {
try {
sessionService.startSession();
List repositories = repositoryDao.getAllRepositories(ContentType.TEST);
log.debug("Retrieved All Specification Repositories number: " + repositories.size());
return repositories;
} catch (Exception ex) {
throw handleException(RETRIEVE_SUTS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getSpecificationRepositoriesOfAssociatedProject(String repositoryUid) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(repositoryUid);
List repositories = repositoryDao.getAllTestRepositories(repository.getProject().getName());
log.debug("Retrieved Test Repositories Of Associated Project of " + repository.getUid() + " number: " + repositories.size());
return repositories;
} catch (Exception ex) {
throw handleException(RETRIEVE_SPECIFICATION_REPOS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getAllRepositoriesForSystemUnderTest(SystemUnderTest sut) throws GreenPepperServerException {
return getSpecificationRepositoriesForSystemUnderTest(sut);
}
/** {@inheritDoc} */
public List getSpecificationRepositoriesForSystemUnderTest(SystemUnderTest sut) throws GreenPepperServerException {
try {
sessionService.startSession();
List repositories = repositoryDao.getAllTestRepositories(sut.getProject().getName());
log.debug("Retrieved Test Repositories Of Associated Project of " + sut.getName() + " number: " + repositories.size());
return repositories;
} catch (Exception ex) {
throw handleException(RETRIEVE_SPECIFICATION_REPOS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getRequirementRepositoriesOfAssociatedProject(String repositoryUid) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(repositoryUid);
List repositories = repositoryDao.getAllRequirementRepositories(repository.getProject().getName());
log.debug("Retrieved Requirement Repositories Of Associated Project of " + repository.getUid() + " number: " + repositories.size());
return repositories;
} catch (Exception ex) {
throw handleException(RETRIEVE_REQUIREMENT_REPOS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getSystemUnderTestsOfAssociatedProject(String repositoryUid) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(repositoryUid);
List suts = sutDao.getAllForProject(repository.getProject().getName());
log.debug("Retrieved SUTs Of Associated Project of " + repository.getUid() + " number: " + suts.size());
return suts;
} catch (Exception ex) {
throw handleException(RETRIEVE_SUTS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getSystemUnderTestsOfProject(String projectName) throws GreenPepperServerException {
try {
sessionService.startSession();
List suts = sutDao.getAllForProject(projectName);
log.debug("Retrieved SUTs of Project: " + projectName + " number: " + suts.size());
return suts;
} catch (Exception ex) {
throw handleException(RETRIEVE_SUTS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void addSpecificationSystemUnderTest(SystemUnderTest sut, Specification specification) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
Repository repository = loadRepository(specification.getRepository().getUid());
documentDao.addSystemUnderTest(sut, specification);
sessionService.commitTransaction();
log.debug("Added SUT " + sut.getName() + " to SUT list of specification: " + specification.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SPECIFICATION_ADD_SUT_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void removeSpecificationSystemUnderTest(SystemUnderTest sut, Specification specification) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
Repository repository = loadRepository(specification.getRepository().getUid());
documentDao.removeSystemUnderTest(sut, specification);
sessionService.commitTransaction();
log.debug("Removed SUT " + sut.getName() + " to SUT list of specification: " + specification.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SPECIFICATION_REMOVE_SUT_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public boolean doesSpecificationHasReferences(Specification specification) throws GreenPepperServerException {
try {
sessionService.startSession();
boolean hasReferences = !documentDao.getAllReferences(specification).isEmpty();
log.debug("Does Specification " + specification.getName() + " Has References: " + hasReferences);
return hasReferences;
} catch (Exception ex) {
throw handleException(ERROR, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getSpecificationReferences(Specification specification) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(specification.getRepository().getUid());
List references = documentDao.getAllReferences(specification);
log.debug("Retrieved Specification " + specification.getName() + " Test Cases number: " + references.size());
return references;
} catch (Exception ex) {
throw handleException(RETRIEVE_REFERENCES, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getSpecificationExecutions(Specification specification, SystemUnderTest sut, int maxResults) throws GreenPepperServerException {
try {
sessionService.startSession();
/*
* Repository repository = loadRepository(specification.getRepository().getUid());
* verifyRepositoryPermission(repository, Permission.READ);
*/
return documentDao.getSpecificationExecutions(specification, sut, maxResults);
} catch (Exception ex) {
throw handleException(RETRIEVE_EXECUTIONS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Execution getSpecificationExecution(Long id) throws GreenPepperServerException {
try {
sessionService.startSession();
return documentDao.getSpecificationExecution(id);
} catch (Exception ex) {
throw handleException(RETRIEVE_EXECUTIONS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public boolean doesRequirementHasReferences(Requirement requirement) throws GreenPepperServerException {
try {
sessionService.startSession();
boolean hasReferences = !documentDao.getAllReferences(requirement).isEmpty();
log.debug("Does Requirement " + requirement.getName() + " Document Has References: " + hasReferences);
return hasReferences;
} catch (Exception ex) {
throw handleException(ERROR, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getRequirementReferences(Requirement requirement) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(requirement.getRepository().getUid());
List references = documentDao.getAllReferences(requirement);
log.debug("Retrieved Requirement " + requirement.getName() + " Document References number: " + references.size());
return references;
} catch (Exception ex) {
throw handleException(RETRIEVE_REFERENCES, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public RequirementSummary getRequirementSummary(Requirement requirement) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(requirement.getRepository().getUid());
requirement = documentDao.getRequirementByName(repository.getUid(), requirement.getName());
log.debug("Retrieved Requirement " + requirement.getName() + " Summary");
return requirement.getSummary();
} catch (Exception ex) {
throw handleException(ERROR, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Reference getReference(Reference reference) throws GreenPepperServerException {
try {
sessionService.startSession();
Repository repository = loadRepository(reference.getSpecification().getRepository().getUid());
reference = documentDao.get(reference);
if (reference == null) {
return null;
}
log.debug("Retrieved Reference: " + reference.getRequirement().getName() + "," + reference.getSpecification().getName());
return reference;
} catch (Exception ex) {
throw handleException(RETRIEVE_REFERENCE, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public SystemUnderTest getSystemUnderTest(SystemUnderTest sut, Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
SystemUnderTest sutDb = sutDao.getByName(sut.getProject().getName(), sut.getName());
sessionService.commitTransaction();
log.debug("Retrieved SystemUnderTest: " + sut.getName());
return sutDb;
} catch (Exception ex) {
throw handleException(RETRIEVE_SUTS, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void createSystemUnderTest(SystemUnderTest sut, Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
SystemUnderTest newSut = sutDao.create(sut);
sessionService.commitTransaction();
log.debug("Updated SystemUnderTest: " + newSut.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SUT_CREATE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void updateSystemUnderTest(String oldSystemUnderTestName, SystemUnderTest sut, Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
sutDao.update(oldSystemUnderTestName, sut);
sessionService.commitTransaction();
log.debug("Updated SystemUnderTest: " + oldSystemUnderTestName);
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SUT_UPDATE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void removeSystemUnderTest(SystemUnderTest sut, Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
Repository persistedRepository = repositoryDao.getByUID(repository.getUid());
sutDao.remove(sut.getProject().getName(), sut.getName());
sessionService.commitTransaction();
log.debug("Removed SystemUnderTest: " + sut.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SUT_DELETE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void setSystemUnderTestAsDefault(SystemUnderTest sut, Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
sutDao.setAsDefault(sut);
sessionService.commitTransaction();
log.debug("Setted as default SystemUnderTest: " + sut.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SUT_SET_DEFAULT_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public void removeRequirement(Requirement requirement) throws GreenPepperServerException {
try {
sessionService.startSession();
sessionService.beginTransaction();
documentDao.removeRequirement(requirement);
sessionService.commitTransaction();
log.debug("Removed Requirement: " + requirement.getName());
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(REQUIREMENT_REMOVE_FAILED, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Specification getSpecification(Specification specification) throws GreenPepperServerException {
try {
sessionService.startSession();
Specification specificationFound = documentDao.getSpecificationByName(specification.getRepository().getUid(), specification.getName());
if (specificationFound != null) {
log.debug("Specification found : " + specificationFound.getName());
}
return specificationFound;
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SPECIFICATION_NOT_FOUND, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Specification getSpecificationById(Long id) throws GreenPepperServerException {
try {
sessionService.startSession();
Specification specificationFound = documentDao.getSpecificationById(id);
if (specificationFound != null) {
log.debug("Specification found : " + specificationFound.getName());
}
return specificationFound;
} catch (Exception ex) {
sessionService.rollbackTransaction();
throw handleException(SPECIFICATION_NOT_FOUND, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public List getSpecifications(SystemUnderTest systemUnderTest, Repository repository) throws GreenPepperServerException {
try {
sessionService.startSession();
List specifications = documentDao.getSpecifications(systemUnderTest, repository);
log.debug("Retrieved specifications for sut: " + systemUnderTest.getName() + " and repoUID:" + repository.getUid());
return specifications;
} catch (Exception ex) {
throw handleException(SPECIFICATIONS_NOT_FOUND, ex);
} finally {
sessionService.closeSession();
}
}
/** {@inheritDoc} */
public Vector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy