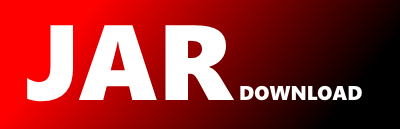
com.github.streamone.shiro.session.RedissonSessionDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shiro-redisson Show documentation
Show all versions of shiro-redisson Show documentation
A redis based implementation of Shiro Cache, using redisson for basic capabilities.
The newest version!
package com.github.streamone.shiro.session;
import org.apache.shiro.session.Session;
import org.apache.shiro.session.UnknownSessionException;
import org.apache.shiro.session.mgt.eis.AbstractSessionDAO;
import org.apache.shiro.session.mgt.eis.SessionDAO;
import org.redisson.RedissonScript;
import org.redisson.api.RScript;
import org.redisson.api.RedissonClient;
import org.redisson.client.codec.Codec;
import org.redisson.codec.JsonJacksonCodec;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* A {@link SessionDAO} implementation backed by Redisson Objects.
*
* @author streamone
*/
public class RedissonSessionDao extends AbstractSessionDAO {
public static final String SESSION_INFO_KEY_PREFIX = "session:info:";
public static final String SESSION_ATTR_KEY_PREFIX = "session:attr:";
private RedissonClient redisson;
private Codec codec = new JsonJacksonCodec();
@Override
protected Serializable doCreate(Session session) {
Serializable sessionId = generateSessionId(session);
assignSessionId(session, sessionId);
String infoKey = getSessionInfoKey(sessionId.toString());
String attrKey = getSessionAttrKey(sessionId.toString());
new RedissonSession(this.redisson, this.codec, infoKey, attrKey, session);
return sessionId;
}
@Override
protected Session doReadSession(Serializable sessionId) {
String infoKey = getSessionInfoKey(sessionId.toString());
String attrKey = getSessionAttrKey(sessionId.toString());
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy