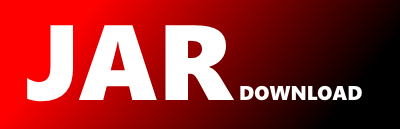
com.wuku.stater.redis.service.RedisService Maven / Gradle / Ivy
package com.wuku.stater.redis.service;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.wuku.stater.redis.properties.RedisProperties;
/**
*
*
* @author SunMy
*
*/
public abstract class RedisService {
protected RedisProperties redisProperties;
public RedisService(RedisProperties redisProperties) {
this.redisProperties = redisProperties;
}
/**
* Redis settings
*
* @param key
* @param value
* @return
*/
public abstract String set(String key, String value);
/**
* Get string object
*
* @param key
* @return
*/
public abstract String get(String key);
/**
* Get map object
*
* @param key
* @return
*/
public abstract Map hgetAll(String key);
/**
* Get all elements in the collection
*
* @param key
* @return
*/
public abstract Set smembers(String key);
/**
* Determine whether an element exists in the set
*
* @param key
* @param member
* @return
*/
public abstract boolean sismember(String key, String member);
/**
* Add multiple elements to the collection
*
* @param key
* @param members
* @return
*/
public Long sadd(String key, List members) {
String[] strArray = new String[members.size()];
strArray = members.toArray(strArray);
return sadd(key, strArray);
}
/**
* Add multiple elements to the collection
*
* @param key
* @param members
* @return
*/
public abstract Long sadd(String key, String... members);
/**
* Set TTL value
*
* @param key
* @param ttl
* @return
*/
public abstract Long ttl(String key, int ttl);
/**
* Set TTL value in batch
*
* @param ttl
* @param keys
* @return
*/
public abstract boolean ttl(int ttl, String... keys);
public abstract Long incr(String key);
public abstract Long incrBy(String key, long increment);
public abstract Double incrByFloat(String key, double increment);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy