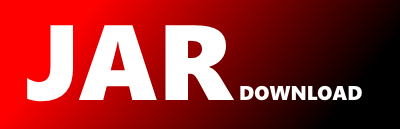
net.abc.tool.util.base.ObjectUtils Maven / Gradle / Ivy
package net.abc.tool.util.base;
import java.lang.reflect.Array;
import java.util.Collection;
import java.util.Map;
import java.util.Optional;
/**
* @author: zhangwei
* @date: 23:54/2018-11-29
*/
public abstract class ObjectUtils {
public static boolean isArray(Object obj) {
return (obj != null && obj.getClass().isArray());
}
public static boolean isEmpty(Object[] array) {
return (array == null || array.length == 0);
}
public static boolean isEmpty(Object obj) {
if (obj == null) {
return true;
}
if (obj instanceof Optional) {
return !((Optional) obj).isPresent();
}
if (obj instanceof CharSequence) {
return ((CharSequence) obj).length() == 0;
}
if (obj.getClass().isArray()) {
return Array.getLength(obj) == 0;
}
if (obj instanceof Collection) {
return ((Collection) obj).isEmpty();
}
if (obj instanceof Map) {
return ((Map) obj).isEmpty();
}
// else
return false;
}
public static A[] addObjectToArray(A[] array, O obj) {
Class> compType = Object.class;
if (array != null) {
compType = array.getClass().getComponentType();
}
else if (obj != null) {
compType = obj.getClass();
}
int newArrLength = (array != null ? array.length + 1 : 1);
@SuppressWarnings("unchecked")
A[] newArr = (A[]) Array.newInstance(compType, newArrLength);
if (array != null) {
System.arraycopy(array, 0, newArr, 0, array.length);
}
newArr[newArr.length - 1] = obj;
return newArr;
}
public static T checkNotNull(T arg, String text) {
if (arg == null) {
throw new NullPointerException(text);
} else {
return arg;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy