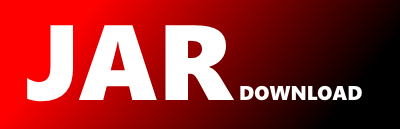
net.abc.tool.util.date.DateUtil Maven / Gradle / Ivy
package net.abc.tool.util.date;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
/**
* @author: zhangwei
* @date: 11:29/2019-01-05
*/
public class DateUtil {
private static Calendar getCalendar() {
return Calendar.getInstance();
}
/**
* 修改日期
* @param date
* @param offset
* @return
*/
public static Date addDay(Date date, int offset){
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.add(5, offset);
return calendar.getTime();
}
/**
* 时分秒格式化成 0
* @param date
* @return
*/
public static Date truncDay(Date date){
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(14, 0);
calendar.set(13, 0);
calendar.set(12, 0);
calendar.set(11, 0);
return calendar.getTime();
}
public static Date truncTime(Date date, ETimeUnit unit){
Calendar cal = getCalendar();
cal.setTime(date);
switch(unit) {
case WEEK:
cal.set(7, 2);
break;
case MONTH:
cal.set(5, 1);
break;
case SEASON:
int month = cal.get(2);
boolean var6;
if (month < 3) {
var6 = false;
} else if (month < 6) {
var6 = true;
} else if (month < 9) {
var6 = true;
} else {
var6 = true;
}
cal.set(5, 1);
break;
case YEAR:
cal.set(2, 0);
cal.set(5, 1);
}
cal.set(11, 0);
cal.set(12, 0);
cal.set(13, 0);
cal.set(14, 0);
return cal.getTime();
}
public static Date addDate(Date date, ETimeUnit unit, int offset) {
Calendar cal = getCalendar();
cal.setTime(date);
switch (unit) {
case DAY:
cal.add(5, offset);
break;
case WEEK:
cal.add(3, offset);
break;
case TENDAYS:
cal.add(5, offset * 10);
break;
case MONTH:
cal.add(2, offset);
break;
case SEASON:
cal.add(2, offset * 4);
break;
case YEAR:
cal.add(1, offset);
}
return cal.getTime();
}
/**
* 将时间格式化成指定格式的字符串
* @param date
* @param pattern
* @return
*/
public static String format(Date date, String pattern){
SimpleDateFormat format = new SimpleDateFormat(pattern);
return format.format(date);
}
/**
* 将字符串解析成时间
* @param source
* @return
* @throws ParseException
*/
public static Date parse(String source) throws ParseException {
SimpleDateFormat format = new SimpleDateFormat();
return format.parse(source);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy