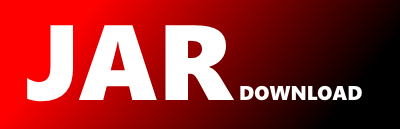
com.github.t9t.jooq.json.JsonDSL Maven / Gradle / Ivy
Show all versions of jooq-postgresql-json Show documentation
package com.github.t9t.jooq.json;
import org.jooq.Field;
import org.jooq.impl.DSL;
import java.util.Collection;
/**
* Functions for {@code json} PostgreSQL operator support in jOOQ
*
* Reference: https://www.postgresql.org/docs/11/functions-json.html
*/
public final class JsonDSL {
/**
* Create a jOOQ {@link Field} wrapping a {@link Json} object representing a {@code json} value for the JSON
* string. Note that the JSON is not validated (any formatting errors will only occur when
* interacting with the database).
*
* @param json JSON string
* @return {@code json} {@code Field} for the JSON string
*/
public static Field field(String json) {
return field(Json.of(json));
}
/**
* Create a jOOQ {@link Field} wrapping the {@link Json} object.
*
* @param json {@code Jsonb} object to wrap
* @return {@code json} {@code Field} for the {@code Jsonb} object
*/
public static Field field(Json json) {
return DSL.field("{0}", Json.class, json);
}
/**
* Get JSON array element (indexed from zero, negative integers count from the end), using the
* ->
operator
*
* Example: '[{"a":"foo"},{"b":"bar"},{"c":"baz"}]'::json->2
* Example result: {"c":"baz"}
*
* @param jsonField A JSON {@code Field} containing an array to get the array element from
* @param index Array index; negative values count from the end
* @return A {@code Field} representing the extracted array element
*/
public static Field arrayElement(Field jsonField, int index) {
return DSL.field("{0}->{1}", Json.class, jsonField, index);
}
/**
* Get JSON array element as {@code text} rather than {@code json(b)} (indexed from zero, negative integers
* count from the end), using the ->>
operator
*
* Example: '[1,2,3]'::json->>2
* Example result: 3
*
* @param jsonField A JSON {@code Field} containing an array to get the array element from
* @param index Array index; negative values count from the end
* @return A {@code Field} representing the extracted array element, as text
*/
public static Field arrayElementText(Field jsonField, int index) {
return DSL.field("{0}->>{1}", String.class, jsonField, index);
}
/**
* Get JSON object field by key using the ->
operator
*
* Example: '{"a": {"b":"foo"}}'::json->'a'
* Example result: {"b":"foo"}
*
* @param jsonField The JSON {@code Field} to extract the field from
* @param key JSON field key name
* @return A {@code Field} representing the extracted value
*/
public static Field fieldByKey(Field jsonField, String key) {
return DSL.field("{0}->{1}", Json.class, jsonField, key);
}
/**
* Get JSON object field as {@code text} rather than {@code json(b)}, using the ->>
* operator
*
* Example: '{"a":1,"b":2}'::json->>'b'
* Example result: 2
*
* @param jsonField The JSON {@code Field} to extract the field from
* @param key JSON field key name
* @return A {@code Field} representing the extracted array element, as text
*/
public static Field fieldByKeyText(Field jsonField, String key) {
return DSL.field("{0}->>{1}", String.class, jsonField, key);
}
/**
* Get JSON object at specified path using the #>
operator
*
* Example: '{"a": {"b":{"c": "foo"}}}'::json#>'{a,b}'
* Example result: {"c": "foo"}
*
* @param jsonField The JSON {@code Field} to extract the path from
* @param path Path to the the object to return
* @return A {@code Field} representing the object at the specified path
* @see #objectAtPath(Field, Collection)
*/
public static Field objectAtPath(Field jsonField, String... path) {
return DSL.field("{0}#>{1}", Json.class, jsonField, DSL.array(path));
}
/**
* Get JSON object at specified path using the #>
operator
*
* Example: '{"a": {"b":{"c": "foo"}}}'::json#>'{a,b}'
* Example result: {"c": "foo"}
*
* @param jsonField The JSON {@code Field} to extract the path from
* @param path Path to the the object to return
* @return A {@code Field} representing the object at the specified path
* @see #objectAtPath(Field, String...)
*/
public static Field objectAtPath(Field jsonField, Collection path) {
return objectAtPath(jsonField, path.toArray(new String[0]));
}
/**
* Get JSON object at specified path as {@code text} rather than {@code json(b)}, using the #>>
* operator
*
* Example: '{"a":[1,2,3],"b":[4,5,6]}'::json#>>'{a,2}'
* Example result: 3
*
* @param jsonField The JSON {@code Field} to extract the path from
* @param path Path to the the object to return
* @return A {@code Field} representing the object at the specified path, as text
* @see #objectAtPathText(Field, Collection)
*/
public static Field objectAtPathText(Field jsonField, String... path) {
return DSL.field("{0}#>>{1}", String.class, jsonField, DSL.array(path));
}
/**
* Get JSON object at specified path as {@code text} rather than {@code json(b)}, using the #>>
* operator
*
* Example: '{"a":[1,2,3],"b":[4,5,6]}'::json#>>'{a,2}'
* Example result: 3
*
* @param jsonField The JSON {@code Field} to extract the path from
* @param path Path to the the object to return
* @return A {@code Field} representing the object at the specified path, as text
* @see #objectAtPath(Field, String...)
*/
public static Field objectAtPathText(Field jsonField, Collection path) {
return objectAtPathText(jsonField, path.toArray(new String[0]));
}
}