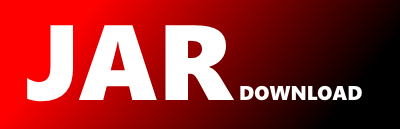
com.github.tadukoo.util.functional.function.ThrowingFunction Maven / Gradle / Ivy
package com.github.tadukoo.util.functional.function;
import java.util.function.Function;
/**
* A better version of Java's {@link Function} interface that
* allows for the functions to throw whatever {@link Throwable} is
* specified.
*
* @param The input argument type for the function
* @param The output result type for the function
* @param The type of {@link Throwable} thrown by the function
*
* @author Logan Ferree (Tadukoo)
* @version 0.1-Alpha-SNAPSHOT
*/
@FunctionalInterface
public interface ThrowingFunction{
/**
* Takes a single argument and returns a result.
*
* @param a The argument
* @return A result
* @throws T Determined by the function, not required
*/
R apply(A a) throws T;
/**
* Creates a ThrowingFunction that runs the given ThrowingFunction and puts the result
* into this ThrowingFunction.
*
* @param The input type to the composed ThrowingFunction
* @param before The ThrowingFunction to run before this one, and put the result into this one
* @return The ThrowingFunction made from composing this one and the given one
*/
default ThrowingFunction compose(ThrowingFunction super S, ? extends A, ? extends T> before){
return v -> this.apply(before.apply(v));
}
/**
* Creates a ThrowingFunction that runs this ThrowingFunction and puts the result
* into the given ThrowingFunction.
*
* @param The output type of the 2nd ThrowingFunction
* @param after A 2nd ThrowingFunction to put the result of this one into
* @return The ThrowingFunction made from composing this one and the given one
*/
default ThrowingFunction andThen(ThrowingFunction super R, ? extends S, ? extends T> after){
return a -> after.apply(this.apply(a));
}
/**
* Returns a ThrowingFunction that always returns its input argument
*
* @param The type of argument
* @param The {@link Throwable} being thrown
* @return A ThrowingFunction that always returns its input argument
*/
static ThrowingFunction identity(){
return a -> a;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy