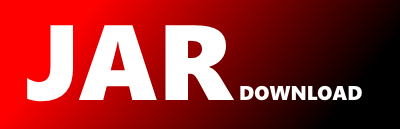
com.github.tadukoo.util.functional.function.ThrowingFunction7 Maven / Gradle / Ivy
package com.github.tadukoo.util.functional.function;
/**
* A function that takes seven arguments, returns a result,
* and may throw a {@link Throwable}.
*
* @param The 1st input argument type for the function
* @param The 2nd input argument type for the function
* @param The 3rd input argument type for the function
* @param The 4th input argument type for the function
* @param The 5th input argument type for the function
* @param The 6th input argument type for the function
* @param The 7th input argument type for the function
* @param The output result type for the function
* @param The type of {@link Throwable} thrown by the function
*
* @author Logan Ferree (Tadukoo)
* @version Alpha v.0.3
*/
@FunctionalInterface
public interface ThrowingFunction7{
/**
* Takes seven arguments and returns a result.
*
* @param a The 1st argument
* @param b The 2nd argument
* @param c The 3rd argument
* @param d The 4th argument
* @param e The 5th argument
* @param f The 6th argument
* @param g The 7th argument
* @return A result
* @throws T Determined by the function, not required
*/
R apply(A a, B b, C c, D d, E e, F f, G g) throws T;
/**
* Creates a ThrowingFunction7 that runs this ThrowingFunction7 and
* puts the result into the given {@link ThrowingFunction}.
*
* @param The output type of the {@link ThrowingFunction}
* @param after A {@link ThrowingFunction} to put the result of this ThrowingFunction7 into
* @return The ThrowingFunction7 made from composing this one and the given {@link ThrowingFunction}
*/
default ThrowingFunction7 andThen(
ThrowingFunction super R, ? extends S, ? extends T> after){
return (a, b, c, d, e, f, g) -> after.apply(this.apply(a, b, c, d, e, f, g));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy