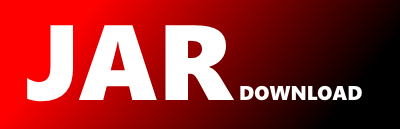
com.github.talberto.easybeans.atg.NucleusEntityManager Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2014 Tomas Rodriguez
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.talberto.easybeans.atg;
import java.util.Map;
import javax.transaction.TransactionManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import atg.dtm.TransactionDemarcation;
import atg.dtm.TransactionDemarcationException;
import atg.nucleus.GenericService;
import com.github.talberto.easybeans.api.EntityManager;
import com.github.talberto.easybeans.api.MappingException;
import com.google.common.collect.Maps;
/**
*
* @author Tomas Rodriguez ([email protected])
*
*/
public class NucleusEntityManager extends GenericService implements EntityManager {
protected Logger mLog = LoggerFactory.getLogger(this.getClass());
protected Map, RepositoryBeanMapper>> mMapperForType = Maps.newConcurrentMap();
protected TransactionManager mTransactionManager;
@Override
public T find(Class pType, Object pPk) {
mLog.trace("Entering find");
return findMapperFor(pType).find(pPk);
}
private RepositoryBeanMapper findMapperFor(Class pType) {
mLog.trace("Entering findMapperFor({})", pType);
// Look first in the map
mLog.debug("Looking for a RepositoryBeanMapper for the type {}", pType);
@SuppressWarnings("unchecked")
RepositoryBeanMapper mapper = (RepositoryBeanMapper) mMapperForType.get(pType);
if(mapper == null) {
mLog.debug("RepositoryBeanMapper for type {} not found, creating one", pType);
mapper = RepositoryBeanMapper.from(this, pType);
mMapperForType.put(pType, mapper);
}
return mapper;
}
@Override
public String create(T pBean) {
mLog.trace("Entering create");
TransactionDemarcation td = new TransactionDemarcation();
boolean rollback = true;
try {
td.begin(mTransactionManager, TransactionDemarcation.REQUIRED);
@SuppressWarnings("unchecked")
Class type = (Class) pBean.getClass();
String id = findMapperFor(type).create(pBean);
rollback = false;
return id;
} catch (TransactionDemarcationException e) {
throw new MappingException(e);
} finally {
try {
td.end(rollback);
} catch (TransactionDemarcationException e) {
throw new MappingException(e);
}
}
}
@Override
public void update(T pBean) {
mLog.trace("Entering update");
TransactionDemarcation td = new TransactionDemarcation();
boolean rollback = true;
try {
td.begin(mTransactionManager, TransactionDemarcation.REQUIRED);
@SuppressWarnings("unchecked")
Class type = (Class) pBean.getClass();
findMapperFor(type).update(pBean);
rollback = false;
} catch (TransactionDemarcationException e) {
throw new MappingException(e);
} finally {
try {
td.end(rollback);
} catch (TransactionDemarcationException e) {
throw new MappingException(e);
}
}
}
/**
* @return the transactionManager
*/
public TransactionManager getTransactionManager() {
return mTransactionManager;
}
/**
* @param pTransactionManager the transactionManager to set
*/
public void setTransactionManager(TransactionManager pTransactionManager) {
mTransactionManager = pTransactionManager;
}
@Override
public void delete(T pBean) {
mLog.trace("Entering delete");
TransactionDemarcation td = new TransactionDemarcation();
boolean rollback = true;
try {
td.begin(mTransactionManager, TransactionDemarcation.REQUIRED);
@SuppressWarnings("unchecked")
Class type = (Class) pBean.getClass();
findMapperFor(type).delete(pBean);
rollback = false;
} catch (TransactionDemarcationException e) {
throw new MappingException(e);
} finally {
try {
td.end(rollback);
} catch (TransactionDemarcationException e) {
throw new MappingException(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy