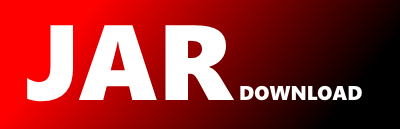
techflavors.tools.smc.converters.MessageConverters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simple-message-converters Show documentation
Show all versions of simple-message-converters Show documentation
Use to convert an object from one type to another using simple way
The newest version!
package techflavors.tools.smc.converters;
import java.lang.reflect.Array;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.inject.Inject;
import techflavors.tools.smc.utils.ReflectionUtil;
/**
* The core class for Message Conversion
*
*
* - If this component is used as part javax dependency injection framework
* (like Spring), create a bean as shown below
*
*
* {@code @Bean("RestConvertors")}
* public MessageConverters converters() {
* return new MessageConverters(
* "techflavors.tools.smc.samples.rest.convertors");
* }
*
*
*
* - For using this converter inject the converter as
*
*
* {@code @Inject @Named(name="RestConvertors") }
* MessageConverters messageConverters;
*
* public void somemethod(){
* messageConverters.toTargetType(obj);
* }
*
*
* - If it is used with non dependency framework, create a singleton instance
* of this class (per conversion) and invoke {@link #setMessageConverters(List)}
* and TestMessageConversionWithoutDependencyInjection
*
*
*
*
*/
public class MessageConverters {
private final int TO_TARGET = 1;
private final int TO_SOURCE = 2;
private Map, MessageConverter, ?>> messageConverters = new HashMap, MessageConverter, ?>>();
private String convertorsBasePackage;
/**
* Base package name. This is to avoid conflict between multiple converters
*
* @param convertorsBasePackage
*/
public MessageConverters(String convertorsBasePackage) {
this.convertorsBasePackage = convertorsBasePackage;
}
@Inject
public void setMessageConverters(List> converters) {
for (MessageConverter, ?> converter : converters) {
if (!converter.getClass().getPackage().getName().startsWith(convertorsBasePackage))
continue;
converter.messageConverters = this;
Class> sourceTypeClass = ReflectionUtil.getSuperClassGenericType(converter.getClass(), 0);
Class> targetTypeClass = ReflectionUtil.getSuperClassGenericType(converter.getClass(), 1);
Method toTargetTypeMethod = ReflectionUtil.findMethod(converter.getClass(), "toTargetType",
sourceTypeClass);
Method toTargetTypeMethodWithParam = ReflectionUtil.findMethod(converter.getClass(), "toTargetType",
sourceTypeClass, Map.class);
Method toSourceTypeMethod = ReflectionUtil.findMethod(converter.getClass(), "toSourceType",
targetTypeClass);
Method toSourceTypeWithParam = ReflectionUtil.findMethod(converter.getClass(), "toSourceType",
targetTypeClass, Map.class);
if (toTargetTypeMethod != null || toTargetTypeMethodWithParam != null) {
messageConverters.put(sourceTypeClass, converter);
}
if (toSourceTypeMethod != null || toSourceTypeWithParam != null) {
messageConverters.put(targetTypeClass, converter);
}
}
}
public TargetType toTargetType(Object data) {
return convertToTargetType(data, null);
}
public TargetType toTargetType(Object data, Map param) {
return convertToTargetType(data, param);
}
public SourceType toSourceType(Object data) {
return convertoSourceType(data, null);
}
public SourceType toSourceType(Object data, Map param) {
return convertoSourceType(data, param);
}
public Collection toTargetTypeAsCollection(Object data) {
return convertAsCollection(convertToTargetType(data, null));
}
public Collection toTargetTypeAsCollection(Object data, Map param) {
return convertAsCollection(convertToTargetType(data, param));
}
public Collection toSourceTypeAsCollection(Object data) {
return convertAsCollection(convertoSourceType(data, null));
}
public Collection toSourceTypeAsCollection(Object data, Map param) {
return convertAsCollection(convertoSourceType(data, param));
}
public TargetType[] toTargetTypeAsArray(Object data) {
return convertAsArray(convertToTargetType(data, null));
}
public TargetType[] toTargetTypeAsArray(Object data, Map param) {
return convertAsArray(convertToTargetType(data, param));
}
public SourceType[] toSourceTypeAsArray(Object data) {
return convertAsArray(convertoSourceType(data, null));
}
public SourceType[] toSourceTypeAsArray(Object data, Map param) {
return convertAsArray(convertoSourceType(data, param));
}
private TargetType convertToTargetType(Object data, Map param) {
return convert(data, param, TO_TARGET);
}
private SourceType convertoSourceType(Object data, Map param) {
return convert(data, param, TO_SOURCE);
}
@SuppressWarnings("unchecked")
private ReturnType convert(Object data, Map param, int dir) {
ReturnType result = null;
if (data instanceof Object[]) {
result = (ReturnType) convertInternal((Object[]) data, param, dir);
} else if (data instanceof List) {
result = (ReturnType) convertInternal((List>) data, param, dir);
} else if (data instanceof Set) {
result = (ReturnType) convertInternal((Set>) data, param, dir);
} else {
result = (ReturnType) convertInternal(data, param, dir);
}
return result;
}
private List> convertInternal(List> data, Map param, int dir) {
if (data == null || data.size() == 0) {
return Collections.EMPTY_LIST;
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy