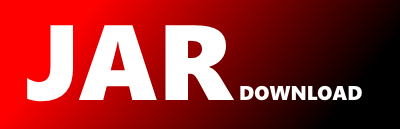
com.pacific.guava.jvm.domain.Source.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of guava-jvm Show documentation
Show all versions of guava-jvm Show documentation
One kind of boilerplate for writing Android apps using android official architecture component approach.
package com.pacific.guava.jvm.domain
sealed class Source {
data class Success(val data: T) : Source()
data class Error(val throwable: Throwable) : Source()
/**
* Returns the available data or throws [NullPointerException] if there is no data.
*/
fun requireData(): T {
return when (this) {
is Success -> data
is Error -> throw throwable
}
}
fun requireError(): Throwable {
return when (this) {
is Success -> throw IllegalStateException("no throwable for Success.Data")
is Error -> throwable
}
}
/**
* If this [Source] is of type [Source.Error], throws the exception
* Otherwise, does nothing.
*/
fun throwIfError() {
if (this is Error) {
throw throwable
}
}
/**
* If this [Source] is of type [Source.Error], returns the available error
* from it. Otherwise, returns `null`.
*/
fun errorOrNull(): Throwable? = when (this) {
is Error -> throwable
else -> null
}
/**
* If there is data available, returns it; otherwise returns null.
*/
fun dataOrNull(): T? = when (this) {
is Success -> data
else -> null
}
fun swapType(): Source = when (this) {
is Error -> Error(throwable)
is Success -> throw IllegalStateException("cannot swap type for Success.Data")
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy